holoviews.core Package#
core
Package#
- class holoviews.core.AdjointLayout(data, **params)[source]#
Bases:
Layoutable
,Dimensioned
An AdjointLayout provides a convenient container to lay out some marginal plots next to a primary plot. This is often useful to display the marginal distributions of a plot next to the primary plot. AdjointLayout accepts a list of up to three elements, which are laid out as follows with the names ‘main’, ‘top’ and ‘right’:
3 | ||___________|___| | | | 1: main | | | 2: right | 1 | 2 | 3: top | | | |___________|___|
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: group, cdims, vdimskdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[Dimension(‘AdjointLayout’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa26810>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Applies to the main object in the AdjointLayout.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get(key, default=None)[source]#
Returns the viewable corresponding to the supplied string or integer based key.
- Args:
key: Numeric or string index: 0) ‘main’ 1) ‘right’ 2) ‘top’ default: Value returned if key not found
- Returns:
Indexed value or supplied default
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from main element
- property label#
Label inherited from main element
- property main#
Returns the main element in the AdjointLayout
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to child up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- property right#
Returns the right marginal element in the AdjointLayout
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property top#
Returns the top marginal element in the AdjointLayout
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.AttrTree(items=None, identifier=None, parent=None, dir_mode='default')[source]#
Bases:
object
An AttrTree offers convenient, multi-level attribute access for collections of objects. AttrTree objects may also be combined together using the update method or merge classmethod. Here is an example of adding a ViewableElement to an AttrTree and accessing it:
>>> t = AttrTree() >>> t.Example.Path = 1 >>> t.Example.Path 1
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- class holoviews.core.BoundingBox(**args)[source]#
Bases:
BoundingRegion
A rectangular bounding box defined either by two points forming an axis-aligned rectangle (or simply a radius for a square).
- contains(x, y)[source]#
Returns true if the given point is contained within the bounding box, where all boundaries of the box are considered to be inclusive.
- contains_exclusive(x, y)[source]#
Return True if the given point is contained within the bounding box, where the bottom and right boundaries are considered exclusive.
- containsbb_exclusive(x)[source]#
Returns true if the given BoundingBox x is contained within the bounding box, where at least one of the boundaries of the box has to be exclusive.
- class holoviews.core.BoundingEllipse(**args)[source]#
Bases:
BoundingBox
Similar to BoundingBox, but the region is the ellipse inscribed within the rectangle.
- contains(x, y)[source]#
Returns true if the given point is contained within the bounding box, where all boundaries of the box are considered to be inclusive.
- contains_exclusive(x, y)[source]#
Return True if the given point is contained within the bounding box, where the bottom and right boundaries are considered exclusive.
- containsbb_exclusive(x)[source]#
Returns true if the given BoundingBox x is contained within the bounding box, where at least one of the boundaries of the box has to be exclusive.
- class holoviews.core.Collator(data=None, **params)[source]#
Bases:
NdMapping
Collator is an NdMapping type which can merge any number of HoloViews components with whatever level of nesting by inserting the Collators key dimensions on the HoloMaps. If the items in the Collator do not contain HoloMaps they will be created. Collator also supports filtering of Tree structures and dropping of constant dimensions.
Parameters inherited from:
group
= param.String(allow_refs=False, default=’Collator’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f9b8bd0>)A string describing the data wrapped by the object.
vdims
= param.List(allow_refs=False, bounds=(0, 0), default=[], label=’Vdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f9bb1d0>)Collator operates on HoloViews objects, if vdims are specified a value_transform function must also be supplied.
drop
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Drop’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f9b8bd0>)List of dimensions to drop when collating data, specified as strings.
drop_constant
= param.Boolean(allow_refs=False, default=False, label=’Drop constant’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f96b5d0>)Whether to demote any non-varying key dimensions to constant dimensions.
filters
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Filters’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f9b9150>)List of paths to drop when collating data, specified as strings or tuples.
progress_bar
= param.Parameter(allow_None=True, allow_refs=False, label=’Progress bar’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f652290>)The progress bar instance used to report progress. Set to None to disable progress bars.
merge_type
= param.ClassSelector(allow_refs=False, class_=<class ‘holoviews.core.ndmapping.NdMapping’>, default=<class ‘holoviews.core.spaces.HoloMap’>, label=’Merge type’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f9b9fd0>)value_transform
= param.Callable(allow_None=True, allow_refs=False, label=’Value transform’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f5ba750>)If supplied the function will be applied on each Collator value during collation. This may be used to apply an operation to the data or load references from disk before they are collated into a displayable HoloViews object.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property static_dimensions#
Return all constant dimensions.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.CompositeOverlay(data, kdims=None, vdims=None, **params)[source]#
Bases:
ViewableElement
,Composable
CompositeOverlay provides a common baseclass for Overlay classes.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdims- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, index=None, show_legend=False, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
- dimension: Dimension(s) to compute histogram on,
Falls back the plot dimensions by default.
num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram index (int, optional): Index of layer to apply hist to show_legend (bool, optional): Show legend in histogram
(don’t show legend by default).
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.Dataset(data=None, kdims=None, vdims=None, **kwargs)[source]#
Bases:
Element
Dataset provides a general baseclass for Element types that contain structured data and supports a range of data formats.
The Dataset class supports various methods offering a consistent way of working with the stored data regardless of the storage format used. These operations include indexing, selection and various ways of aggregating or collapsing the data with a supplied function.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’Dataset’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f93e710>)A string describing the data wrapped by the object.
datatype
= param.List(allow_refs=False, bounds=(0, None), default=[‘dataframe’, ‘dictionary’, ‘grid’, ‘xarray’, ‘multitabular’, ‘spatialpandas’, ‘dask_spatialpandas’, ‘dask’, ‘cuDF’, ‘array’, ‘ibis’], label=’Datatype’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14f99c950>)A priority list of the data types to be used for storage on the .data attribute. If the input supplied to the element constructor cannot be put into the requested format, the next format listed will be used until a suitable format is found (or the data fails to be understood).
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the Dataset
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or array of values, matching the length or shape of the Dataset.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int): Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- aggregate(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Aggregates data on the supplied dimensions.
Aggregates over the supplied key dimensions with the defined function or dim_transform specified as a tuple of the transformed dimension name and dim transform.
- Args:
- dimensions: Dimension(s) to aggregate on
Default to all key dimensions
- function: Aggregation function or transform to apply
Supports both simple functions and dimension transforms
- spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **kwargs: Keyword arguments either passed to the aggregation function
or to create new names for the transformed variables
- Returns:
Returns the aggregated Dataset
- array(dimensions=None)[source]#
Convert dimension values to columnar array.
- Args:
dimensions: List of dimensions to return
- Returns:
Array of columns corresponding to each dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- closest(coords=None, **kwargs)[source]#
Snaps coordinate(s) to closest coordinate in Dataset
- Args:
coords: List of coordinates expressed as tuples **kwargs: Coordinates defined as keyword pairs
- Returns:
List of tuples of the snapped coordinates
- Raises:
NotImplementedError: Raised if snapping is not supported
- columns(dimensions=None)[source]#
Convert dimension values to a dictionary.
Returns a dictionary of column arrays along each dimension of the element.
- Args:
dimensions: Dimensions to return as columns
- Returns:
Dictionary of arrays for each dimension
- compute()[source]#
Computes the data to a data format that stores the daata in memory, e.g. a Dask dataframe or array is converted to a Pandas DataFrame or NumPy array.
- Returns:
Dataset with the data stored in in-memory format
- property dataset#
The Dataset that this object was created from
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- groupby(dimensions=None, container_type=<class 'holoviews.core.spaces.HoloMap'>, group_type=None, dynamic=False, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of element and histogram or just the histogram
- property iloc#
Returns iloc indexer with support for columnar indexing.
Returns an iloc object providing a convenient interface to slice and index into the Dataset using row and column indices. Allow selection by integer index, slice and list of integer indices and boolean arrays.
Examples:
Index the first row and column:
dataset.iloc[0, 0]
Select rows 1 and 2 with a slice:
dataset.iloc[1:3, :]
Select with a list of integer coordinates:
dataset.iloc[[0, 2, 3]]
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- property ndloc#
Returns ndloc indexer with support for gridded indexing.
Returns an ndloc object providing nd-array like indexing for gridded datasets. Follows NumPy array indexing conventions, allowing for indexing, slicing and selecting a list of indices on multi-dimensional arrays using integer indices. The order of array indices is inverted relative to the Dataset key dimensions, e.g. an Image with key dimensions ‘x’ and ‘y’ can be indexed with
image.ndloc[iy, ix]
, whereiy
andix
are integer indices along the y and x dimensions.Examples:
Index value in 2D array:
dataset.ndloc[3, 1]
Slice along y-axis of 2D array:
dataset.ndloc[2:5, :]
Vectorized (non-orthogonal) indexing along x- and y-axes:
dataset.ndloc[[1, 2, 3], [0, 2, 3]]
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- persist()[source]#
Persists the results of a lazy data interface to memory to speed up data manipulation and visualization. If the particular data backend already holds the data in memory this is a no-op. Unlike the compute method this maintains the same data type.
- Returns:
Dataset with the data persisted to memory
- property pipeline#
Chain operation that evaluates the sequence of operations that was used to create this object, starting with the Dataset stored in dataset property
- range(dim, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reduce(dimensions=None, function=None, spreadfn=None, **reductions)[source]#
Applies reduction along the specified dimension(s).
Allows reducing the values along one or more key dimension with the supplied function. Supports two signatures:
Reducing with a list of dimensions, e.g.:
ds.reduce([‘x’], np.mean)
Defining a reduction using keywords, e.g.:
ds.reduce(x=np.mean)
- Args:
- dimensions: Dimension(s) to apply reduction on
Defaults to all key dimensions
function: Reduction operation to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **reductions: Keyword argument defining reduction
Allows reduction to be defined as keyword pair of dimension and function
- Returns:
The Dataset after reductions have been applied.
- reindex(kdims=None, vdims=None)[source]#
Reindexes Dataset dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.x
- Args:
kdims (optional): New list of key dimensionsx vdims (optional): New list of value dimensions
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- sample(samples=None, bounds=None, closest=True, **kwargs)[source]#
Samples values at supplied coordinates.
Allows sampling of element with a list of coordinates matching the key dimensions, returning a new object containing just the selected samples. Supports multiple signatures:
Sampling with a list of coordinates, e.g.:
ds.sample([(0, 0), (0.1, 0.2), …])
Sampling a range or grid of coordinates, e.g.:
1D: ds.sample(3) 2D: ds.sample((3, 3))
Sampling by keyword, e.g.:
ds.sample(x=0)
- Args:
samples: List of nd-coordinates to sample bounds: Bounds of the region to sample
Defined as two-tuple for 1D sampling and four-tuple for 2D sampling.
closest: Whether to snap to closest coordinates **kwargs: Coordinates specified as keyword pairs
Keywords of dimensions and scalar coordinates
- Returns:
Element containing the sampled coordinates
- select(selection_expr=None, selection_specs=None, **selection)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
predicate expression: A holoviews.dim expression, e.g.:
from holoviews import dim ds.select(selection_expr=dim(‘x’) % 2 == 0)
- Args:
- selection_expr: holoviews.dim predicate expression
specifying selection.
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property shape#
Returns the shape of the data.
- sort(by=None, reverse=False)[source]#
Sorts the data by the values along the supplied dimensions.
- Args:
by: Dimension(s) to sort by reverse (bool, optional): Reverse sort order
- Returns:
Sorted Dataset
- property to#
Returns the conversion interface with methods to convert Dataset
- transform(*args, **kwargs)[source]#
Transforms the Dataset according to a dimension transform.
Transforms may be supplied as tuples consisting of the dimension(s) and the dim transform to apply or keyword arguments mapping from dimension(s) to dim transforms. If the arg or kwarg declares multiple dimensions the dim transform should return a tuple of values for each.
A transform may override an existing dimension or add a new one in which case it will be added as an additional value dimension.
- Args:
- args: Specify the output arguments and transforms as a
tuple of dimension specs and dim transforms
drop (bool): Whether to drop all variables not part of the transform keep_index (bool): Whether to keep indexes
Whether to apply transform on datastructure with index, e.g. pandas.Series or xarray.DataArray, (important for dask datastructures where index may be required to align datasets).
kwargs: Specify new dimensions in the form new_dim=dim_transform
- Returns:
Transformed dataset with new dimensions
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.Dimension(spec, **params)[source]#
Bases:
Parameterized
Dimension objects are used to specify some important general features that may be associated with a collection of values.
For instance, a Dimension may specify that a set of numeric values actually correspond to ‘Height’ (dimension name), in units of meters, with a descriptive label ‘Height of adult males’.
All dimensions object have a name that identifies them and a label containing a suitable description. If the label is not explicitly specified it matches the name.
These two parameters define the core identity of the dimension object and must match if two dimension objects are to be considered equivalent. All other parameters are considered optional metadata and are not used when testing for equality.
Unlike all the other parameters, these core parameters can be used to construct a Dimension object from a tuple. This format is sufficient to define an identical Dimension:
Dimension(‘a’, label=’Dimension A’) == Dimension((‘a’, ‘Dimension A’))
Everything else about a dimension is considered to reflect non-semantic preferences. Examples include the default value (which may be used in a visualization to set an initial slider position), how the value is to rendered as text (which may be used to specify the printed floating point precision) or a suitable range of values to consider for a particular analysis.
Units#
Full unit support with automated conversions are on the HoloViews roadmap. Once rich unit objects are supported, the unit (or more specifically the type of unit) will be part of the core dimension specification used to establish equality.
Until this feature is implemented, there are two auxiliary parameters that hold some partial information about the unit: the name of the unit and whether or not it is cyclic. The name of the unit is used as part of the pretty-printed representation and knowing whether it is cyclic is important for certain operations.
label
= param.String(allow_None=True, allow_refs=False, label=’Label’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa4f2d0>)Unrestricted label used to describe the dimension. A label should succinctly describe the dimension and may contain any characters, including Unicode and LaTeX expression.
cyclic
= param.Boolean(allow_refs=False, default=False, label=’Cyclic’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa5c150>)Whether the range of this feature is cyclic such that the maximum allowed value (defined by the range parameter) is continuous with the minimum allowed value.
default
= param.Parameter(allow_None=True, allow_refs=False, label=’Default’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa2c050>)Default value of the Dimension which may be useful for widget or other situations that require an initial or default value.
nodata
= param.Integer(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Nodata’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa5e110>)Optional missing-data value for integer data. If non-None, data with this value will be replaced with NaN.
range
= param.Tuple(allow_refs=False, default=(None, None), label=’Range’, length=2, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa2c310>)Specifies the minimum and maximum allowed values for a Dimension. None is used to represent an unlimited bound.
soft_range
= param.Tuple(allow_refs=False, default=(None, None), label=’Soft range’, length=2, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa5e2d0>)Specifies a minimum and maximum reference value, which may be overridden by the data.
step
= param.Number(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Step’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14e183850>)Optional floating point step specifying how frequently the underlying space should be sampled. May be used to define a discrete sampling over the range.
type
= param.Parameter(allow_None=True, allow_refs=False, label=’Type’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa5ec90>)Optional type associated with the Dimension values. The type may be an inbuilt constructor (such as int, str, float) or a custom class object.
unit
= param.String(allow_None=True, allow_refs=False, label=’Unit’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa71510>)Optional unit string associated with the Dimension. For instance, the string ‘m’ may be used represent units of meters and ‘s’ to represent units of seconds.
value_format
= param.Callable(allow_None=True, allow_refs=False, label=’Value format’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa2c310>)Formatting function applied to each value before display.
values
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Values’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa71510>)Optional specification of the allowed value set for the dimension that may also be used to retain a categorical ordering.
- clone(spec=None, **overrides)[source]#
Clones the Dimension with new parameters
Derive a new Dimension that inherits existing parameters except for the supplied, explicit overrides
- Args:
spec (tuple, optional): Dimension tuple specification **overrides: Dimension parameter overrides
- Returns:
Cloned Dimension object
- property pprint_label#
The pretty-printed label string for the Dimension
- pprint_value(value, print_unit=False)[source]#
Applies the applicable formatter to the value.
- Args:
value: Dimension value to format
- Returns:
Formatted dimension value
- pprint_value_string(value)[source]#
Pretty print the dimension value and unit with title_format
- Args:
value: Dimension value to format
- Returns:
Formatted dimension value string with unit
- property spec#
“Returns the Dimensions tuple specification
- Returns:
tuple: Dimension tuple specification
- class holoviews.core.Dimensioned(data, kdims=None, vdims=None, **params)[source]#
Bases:
LabelledData
Dimensioned is a base class that allows the data contents of a class to be associated with dimensions. The contents associated with dimensions may be partitioned into one of three types
- key dimensions: These are the dimensions that can be indexed via
the __getitem__ method. Dimension objects supporting key dimensions must support indexing over these dimensions and may also support slicing. This list ordering of dimensions describes the positional components of each multi-dimensional indexing operation.
For instance, if the key dimension names are ‘weight’ followed by ‘height’ for Dimensioned object ‘obj’, then obj[80,175] indexes a weight of 80 and height of 175.
Accessed using either kdims.
- value dimensions: These dimensions correspond to any data held
on the Dimensioned object not in the key dimensions. Indexing by value dimension is supported by dimension name (when there are multiple possible value dimensions); no slicing semantics is supported and all the data associated with that dimension will be returned at once. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either vdims.
- deep dimensions: These are dynamically computed dimensions that
belong to other Dimensioned objects that are nested in the data. Objects that support this should enable the _deep_indexable flag. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either ddims.
Dimensioned class support generalized methods for finding the range and type of values along a particular Dimension. The range method relies on the appropriate implementation of the dimension_values methods on subclasses.
The index of an arbitrary dimension is its positional index in the list of all dimensions, starting with the key dimensions, followed by the value dimensions and ending with the deep dimensions.
Parameters inherited from:
group
= param.String(allow_refs=False, constant=True, default=’Dimensioned’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa67290>)A string describing the data wrapped by the object.
cdims
= param.Dict(allow_refs=False, class_=<class ‘dict’>, default={}, label=’Cdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa40b90>)The constant dimensions defined as a dictionary of Dimension:value pairs providing additional dimension information about the object. Aliased with constant_dimensions.
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa67290>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
vdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Vdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa40ad0>)The value dimensions defined as the list of dimensions used to describe the components of the data. If multiple value dimensions are supplied, a particular value dimension may be indexed by name after the key dimensions. Aliased with value_dimensions.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.DynamicMap(callback, initial_items=None, streams=None, **params)[source]#
Bases:
HoloMap
A DynamicMap is a type of HoloMap where the elements are dynamically generated by a callable. The callable is invoked with values associated with the key dimensions or with values supplied by stream parameters.
Parameters inherited from:
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa7d5d0>)The key dimensions of a DynamicMap map to the arguments of the callback. This mapping can be by position or by name.
callback
= param.ClassSelector(allow_None=True, allow_refs=False, class_=<class ‘holoviews.core.spaces.Callable’>, constant=True, label=’Callback’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa7ee10>)The callable used to generate the elements. The arguments to the callable includes any number of declared key dimensions as well as any number of stream parameters defined on the input streams. If the callable is an instance of Callable it will be used directly, otherwise it will be automatically wrapped in one.
streams
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Streams’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa7c910>)List of Stream instances to associate with the DynamicMap. The set of parameter values across these streams will be supplied as keyword arguments to the callback when the events are received, updating the streams. Can also be supplied as a dictionary that maps parameters or panel widgets to callback argument names that will then be automatically converted to the equivalent list format.
cache_size
= param.Integer(allow_refs=False, bounds=(1, None), default=500, inclusive_bounds=(True, True), label=’Cache size’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa7ec90>)The number of entries to cache for fast access. This is an LRU cache where the least recently used item is overwritten once the cache is full.
positional_stream_args
= param.Boolean(allow_refs=False, constant=True, default=False, label=’Positional stream args’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fa7f410>)If False, stream parameters are passed to the callback as keyword arguments. If True, stream parameters are passed to callback as positional arguments. Each positional argument is a dict containing the contents of a stream. The positional stream arguments follow the positional arguments for each kdim, and they are ordered to match the order of the DynamicMap’s streams list.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- collate()[source]#
Unpacks DynamicMap into container of DynamicMaps
Collation allows unpacking DynamicMaps which return Layout, NdLayout or GridSpace objects into a single such object containing DynamicMaps. Assumes that the items in the layout or grid that is returned do not change.
- Returns:
Collated container containing DynamicMaps
- property current_key#
Returns the current key value.
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs DynamicMap of nested DynamicMaps into a single DynamicMap that returns a non-dynamic element
Decollation allows packing a DynamicMap of nested DynamicMaps into a single DynamicMap that returns a simple (non-dynamic) element. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a non-dynamic element
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- event(**kwargs)[source]#
Updates attached streams and triggers events
Automatically find streams matching the supplied kwargs to update and trigger events on them.
- Args:
**kwargs: Events to update streams with
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- grid(dimensions=None, **kwargs)[source]#
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a GridSpace.
Args: dimensions: Dimension/str or list
Dimension or list of dimensions to group by
Returns: grid: GridSpace
GridSpace with supplied dimensions
- property group#
Group inherited from items
- groupby(dimensions=None, container_type=None, group_type=None, **kwargs)[source]#
Groups DynamicMap by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of DynamicMap and adjoined histogram if adjoin=True, otherwise just the histogram
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- layout(dimensions=None, **kwargs)[source]#
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a NdLayout.
Args: dimensions: Dimension/str or list
Dimension or list of dimensions to group by
Returns: layout: NdLayout
NdLayout with supplied dimensions
- map(map_fn, specs=None, clone=True, link_inputs=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options defined in a flat format to the objects returned by the DynamicMap. If the options are to be set directly on the objects returned by the DynamicMap a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- overlay(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and overlay each group
Groups data by supplied dimension(s) overlaying the groups along the dimension(s).
- Args:
dimensions: Dimension(s) of dimensions to group by
- Returns:
NdOverlay object(s) with supplied dimensions
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reorders key dimensions on DynamicMap
Create a new object with a reordered set of key dimensions. Dropping dimensions is not allowed on a DynamicMap.
- Args:
kdims: List of dimensions to reindex the mapping with force: Not applicable to a DynamicMap
- Returns:
Reindexed DynamicMap
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- property unbounded#
Returns a list of key dimensions that are unbounded, excluding stream parameters. If any of these key dimensions are unbounded, the DynamicMap as a whole is also unbounded.
- class holoviews.core.Element(data, kdims=None, vdims=None, **params)[source]#
Bases:
ViewableElement
,Composable
,Overlayable
Element is the atomic datastructure used to wrap some data with an associated visual representation, e.g. an element may represent a set of points, an image or a curve. Elements provide a common API for interacting with data of different types and define how the data map to a set of dimensions and how those map to the visual representation.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’Element’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fab7450>)A string describing the data wrapped by the object.
- array(dimensions=None)[source]#
Convert dimension values to columnar array.
- Args:
dimensions: List of dimensions to return
- Returns:
Array of columns corresponding to each dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- closest(coords, **kwargs)[source]#
Snap list or dict of coordinates to closest position.
- Args:
coords: List of 1D or 2D coordinates **kwargs: Coordinates specified as keyword pairs
- Returns:
List of tuples of the snapped coordinates
- Raises:
NotImplementedError: Raised if snapping is not supported
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reduce(dimensions=None, function=None, spreadfn=None, **reduction)[source]#
Applies reduction along the specified dimension(s).
Allows reducing the values along one or more key dimension with the supplied function. Supports two signatures:
Reducing with a list of dimensions, e.g.:
ds.reduce([‘x’], np.mean)
Defining a reduction using keywords, e.g.:
ds.reduce(x=np.mean)
- Args:
- dimensions: Dimension(s) to apply reduction on
Defaults to all key dimensions
function: Reduction operation to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **reductions: Keyword argument defining reduction
Allows reduction to be defined as keyword pair of dimension and function
- Returns:
The element after reductions have been applied.
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- sample(samples=None, bounds=None, closest=False, **sample_values)[source]#
Samples values at supplied coordinates.
Allows sampling of element with a list of coordinates matching the key dimensions, returning a new object containing just the selected samples. Supports multiple signatures:
Sampling with a list of coordinates, e.g.:
ds.sample([(0, 0), (0.1, 0.2), …])
Sampling a range or grid of coordinates, e.g.:
1D: ds.sample(3) 2D: ds.sample((3, 3))
Sampling by keyword, e.g.:
ds.sample(x=0)
- Args:
samples: List of nd-coordinates to sample bounds: Bounds of the region to sample
Defined as two-tuple for 1D sampling and four-tuple for 2D sampling.
closest: Whether to snap to closest coordinates **kwargs: Coordinates specified as keyword pairs
Keywords of dimensions and scalar coordinates
- Returns:
Element containing the sampled coordinates
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.Element2D(data, kdims=None, vdims=None, **params)[source]#
Bases:
Element
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsextents
= param.Tuple(allow_refs=False, default=(None, None, None, None), label=’Extents’, length=4, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fac4890>)Allows overriding the extents of the Element in 2D space defined as four-tuple defining the (left, bottom, right and top) edges.
- array(dimensions=None)[source]#
Convert dimension values to columnar array.
- Args:
dimensions: List of dimensions to return
- Returns:
Array of columns corresponding to each dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- closest(coords, **kwargs)[source]#
Snap list or dict of coordinates to closest position.
- Args:
coords: List of 1D or 2D coordinates **kwargs: Coordinates specified as keyword pairs
- Returns:
List of tuples of the snapped coordinates
- Raises:
NotImplementedError: Raised if snapping is not supported
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reduce(dimensions=None, function=None, spreadfn=None, **reduction)[source]#
Applies reduction along the specified dimension(s).
Allows reducing the values along one or more key dimension with the supplied function. Supports two signatures:
Reducing with a list of dimensions, e.g.:
ds.reduce([‘x’], np.mean)
Defining a reduction using keywords, e.g.:
ds.reduce(x=np.mean)
- Args:
- dimensions: Dimension(s) to apply reduction on
Defaults to all key dimensions
function: Reduction operation to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **reductions: Keyword argument defining reduction
Allows reduction to be defined as keyword pair of dimension and function
- Returns:
The element after reductions have been applied.
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- sample(samples=None, bounds=None, closest=False, **sample_values)[source]#
Samples values at supplied coordinates.
Allows sampling of element with a list of coordinates matching the key dimensions, returning a new object containing just the selected samples. Supports multiple signatures:
Sampling with a list of coordinates, e.g.:
ds.sample([(0, 0), (0.1, 0.2), …])
Sampling a range or grid of coordinates, e.g.:
1D: ds.sample(3) 2D: ds.sample((3, 3))
Sampling by keyword, e.g.:
ds.sample(x=0)
- Args:
samples: List of nd-coordinates to sample bounds: Bounds of the region to sample
Defined as two-tuple for 1D sampling and four-tuple for 2D sampling.
closest: Whether to snap to closest coordinates **kwargs: Coordinates specified as keyword pairs
Keywords of dimensions and scalar coordinates
- Returns:
Element containing the sampled coordinates
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.Element3D(data, kdims=None, vdims=None, **params)[source]#
Bases:
Element2D
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsextents
= param.Tuple(allow_refs=False, default=(None, None, None, None, None, None), label=’Extents’, length=6, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fb01450>)Allows overriding the extents of the Element in 3D space defined as (xmin, ymin, zmin, xmax, ymax, zmax).
- array(dimensions=None)[source]#
Convert dimension values to columnar array.
- Args:
dimensions: List of dimensions to return
- Returns:
Array of columns corresponding to each dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- closest(coords, **kwargs)[source]#
Snap list or dict of coordinates to closest position.
- Args:
coords: List of 1D or 2D coordinates **kwargs: Coordinates specified as keyword pairs
- Returns:
List of tuples of the snapped coordinates
- Raises:
NotImplementedError: Raised if snapping is not supported
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reduce(dimensions=None, function=None, spreadfn=None, **reduction)[source]#
Applies reduction along the specified dimension(s).
Allows reducing the values along one or more key dimension with the supplied function. Supports two signatures:
Reducing with a list of dimensions, e.g.:
ds.reduce([‘x’], np.mean)
Defining a reduction using keywords, e.g.:
ds.reduce(x=np.mean)
- Args:
- dimensions: Dimension(s) to apply reduction on
Defaults to all key dimensions
function: Reduction operation to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **reductions: Keyword argument defining reduction
Allows reduction to be defined as keyword pair of dimension and function
- Returns:
The element after reductions have been applied.
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- sample(samples=None, bounds=None, closest=False, **sample_values)[source]#
Samples values at supplied coordinates.
Allows sampling of element with a list of coordinates matching the key dimensions, returning a new object containing just the selected samples. Supports multiple signatures:
Sampling with a list of coordinates, e.g.:
ds.sample([(0, 0), (0.1, 0.2), …])
Sampling a range or grid of coordinates, e.g.:
1D: ds.sample(3) 2D: ds.sample((3, 3))
Sampling by keyword, e.g.:
ds.sample(x=0)
- Args:
samples: List of nd-coordinates to sample bounds: Bounds of the region to sample
Defined as two-tuple for 1D sampling and four-tuple for 2D sampling.
closest: Whether to snap to closest coordinates **kwargs: Coordinates specified as keyword pairs
Keywords of dimensions and scalar coordinates
- Returns:
Element containing the sampled coordinates
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.Empty(*, cdims, kdims, vdims, group, label, name)[source]#
Bases:
Dimensioned
,Composable
Empty may be used to define an empty placeholder in a Layout. It can be placed in a Layout just like any regular Element and container type via the + operator or by passing it to the Layout constructor as a part of a list.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, default=’Empty’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fb0bb50>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.GridMatrix(initial_items=None, kdims=None, **params)[source]#
Bases:
GridSpace
GridMatrix is container type for heterogeneous Element types laid out in a grid. Unlike a GridSpace the axes of the Grid must not represent an actual coordinate space, but may be used to plot various dimensions against each other. The GridMatrix is usually constructed using the gridmatrix operation, which will generate a GridMatrix plotting each dimension in an Element against each other.
Parameters inherited from:
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace
Decollation allows packing a GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a GridSpace
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- keys(full_grid=False)[source]#
Returns the keys of the GridSpace
- Args:
full_grid (bool, optional): Return full cross-product of keys
- Returns:
List of keys
- property label#
Label inherited from items
- property last#
The last of a GridSpace is another GridSpace constituted of the last of the individual elements. To access the elements by their X,Y position, either index the position directly or use the items() method.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property shape#
Returns the 2D shape of the GridSpace as (rows, cols).
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.GridSpace(initial_items=None, kdims=None, **params)[source]#
Bases:
Layoutable
,UniformNdMapping
Grids are distinct from Layouts as they ensure all contained elements to be of the same type. Unlike Layouts, which have integer keys, Grids usually have floating point keys, which correspond to a grid sampling in some two-dimensional space. This two-dimensional space may have to arbitrary dimensions, e.g. for 2D parameter spaces.
Parameters inherited from:
kdims
= param.List(allow_refs=False, bounds=(1, 2), default=[Dimension(‘X’), Dimension(‘Y’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fb20790>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace
Decollation allows packing a GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a GridSpace
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- keys(full_grid=False)[source]#
Returns the keys of the GridSpace
- Args:
full_grid (bool, optional): Return full cross-product of keys
- Returns:
List of keys
- property label#
Label inherited from items
- property last#
The last of a GridSpace is another GridSpace constituted of the last of the individual elements. To access the elements by their X,Y position, either index the position directly or use the items() method.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property shape#
Returns the 2D shape of the GridSpace as (rows, cols).
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.HoloMap(initial_items=None, kdims=None, group=None, label=None, **params)[source]#
Bases:
Layoutable
,UniformNdMapping
,Overlayable
A HoloMap is an n-dimensional mapping of viewable elements or overlays. Each item in a HoloMap has an tuple key defining the values along each of the declared key dimensions, defining the discretely sampled space of values.
The visual representation of a HoloMap consists of the viewable objects inside the HoloMap which can be explored by varying one or more widgets mapping onto the key dimensions of the HoloMap.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- collate(merge_type=None, drop=None, drop_constant=False)[source]#
Collate allows reordering nested containers
Collation allows collapsing nested mapping types by merging their dimensions. In simple terms in merges nested containers into a single merged type.
In the simple case a HoloMap containing other HoloMaps can easily be joined in this way. However collation is particularly useful when the objects being joined are deeply nested, e.g. you want to join multiple Layouts recorded at different times, collation will return one Layout containing HoloMaps indexed by Time. Changing the merge_type will allow merging the outer Dimension into any other UniformNdMapping type.
- Args:
merge_type: Type of the object to merge with drop: List of dimensions to drop drop_constant: Drop constant dimensions automatically
- Returns:
Collated Layout or HoloMap
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs HoloMap of DynamicMaps into a single DynamicMap that returns an HoloMap
Decollation allows packing a HoloMap of DynamicMaps into a single DynamicMap that returns an HoloMap of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns an HoloMap
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- grid(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and lay out groups in grid
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a GridSpace.
Args: dimensions: Dimension/str or list
Dimension or list of dimensions to group by
- Returns:
GridSpace with supplied dimensions
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, individually=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of HoloMap and histograms or just the histograms
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- layout(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and lay out groups
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a NdLayout.
- Args:
dimensions: Dimension(s) to group by
- Returns:
NdLayout with supplied dimensions
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, **kwargs)[source]#
Applies simplified option definition returning a new object
Applies options defined in a flat format to the objects returned by the DynamicMap. If the options are to be set directly on the objects in the HoloMap a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- overlay(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and overlay each group
Groups data by supplied dimension(s) overlaying the groups along the dimension(s).
- Args:
dimensions: Dimension(s) of dimensions to group by
- Returns:
NdOverlay object(s) with supplied dimensions
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.Layout(items=None, identifier=None, parent=None, **kwargs)[source]#
Bases:
Layoutable
,ViewableTree
A Layout is an ViewableTree with ViewableElement objects as leaf values. Unlike ViewableTree, a Layout supports a rich display, displaying leaf items in a grid style layout. In addition to the usual ViewableTree indexing, Layout supports indexing of items by their row and column index in the layout.
The maximum number of columns in such a layout may be controlled with the cols method.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’Layout’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fbb0650>)A string describing the data wrapped by the object.
- cols(ncols)[source]#
Sets the maximum number of columns in the NdLayout.
Any items beyond the set number of cols will flow onto a new row. The number of columns control the indexing and display semantics of the NdLayout.
- Args:
ncols (int): Number of columns to set on the NdLayout
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs Layout of DynamicMaps into a single DynamicMap that returns a Layout
Decollation allows packing a Layout of DynamicMaps into a single DynamicMap that returns a Layout of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a Layout
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- property shape#
Tuple indicating the number of rows and columns in the Layout.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
- class holoviews.core.MultiDimensionalMapping(initial_items=None, kdims=None, **params)[source]#
Bases:
Dimensioned
An MultiDimensionalMapping is a Dimensioned mapping (like a dictionary or array) that uses fixed-length multidimensional keys. This behaves like a sparse N-dimensional array that does not require a dense sampling over the multidimensional space.
If the underlying value for each (key, value) pair also supports indexing (such as a dictionary, array, or list), fully qualified (deep) indexing may be used from the top level, with the first N dimensions of the index selecting a particular Dimensioned object and the remaining dimensions indexing into that object.
For instance, for a MultiDimensionalMapping with dimensions “Year” and “Month” and underlying values that are 2D floating-point arrays indexed by (r,c), a 2D array may be indexed with x[2000,3] and a single floating-point number may be indexed as x[2000,3,1,9].
In practice, this class is typically only used as an abstract base class, because the NdMapping subclass extends it with a range of useful slicing methods for selecting subsets of the data. Even so, keeping the slicing support separate from the indexing and data storage methods helps make both classes easier to understand.
Parameters inherited from:
group
= param.String(allow_refs=False, constant=True, default=’MultiDimensionalMapping’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fbd0350>)A string describing the data wrapped by the object.
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[Dimension(‘Default’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fbd31d0>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
vdims
= param.List(allow_refs=False, bounds=(0, 0), constant=True, default=[], label=’Vdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fbd0310>)The value dimensions defined as the list of dimensions used to describe the components of the data. If multiple value dimensions are supplied, a particular value dimension may be indexed by name after the key dimensions. Aliased with value_dimensions.
sort
= param.Boolean(allow_refs=False, default=True, label=’Sort’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fbd2bd0>)Whether the items should be sorted in the constructor.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.NdLayout(initial_items=None, kdims=None, **params)[source]#
Bases:
Layoutable
,UniformNdMapping
NdLayout is a UniformNdMapping providing an n-dimensional data structure to display the contained Elements and containers in a layout. Using the cols method the NdLayout can be rearranged with the desired number of columns.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- cols(ncols)[source]#
Sets the maximum number of columns in the NdLayout.
Any items beyond the set number of cols will flow onto a new row. The number of columns control the indexing and display semantics of the NdLayout.
- Args:
ncols (int): Number of columns to set on the NdLayout
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- grid_items()[source]#
Compute a dict of {(row,column): (key, value)} elements from the current set of items and specified number of columns.
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns another NdLayout constituted of the last views of the individual elements (if they are maps).
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property shape#
Tuple indicating the number of rows and columns in the NdLayout.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.NdMapping(initial_items=None, kdims=None, **params)[source]#
Bases:
MultiDimensionalMapping
NdMapping supports the same indexing semantics as MultiDimensionalMapping but also supports slicing semantics.
Slicing semantics on an NdMapping is dependent on the ordering semantics of the keys. As MultiDimensionalMapping sort the keys, a slice on an NdMapping is effectively a way of filtering out the keys that are outside the slice range.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sortgroup
= param.String(allow_refs=False, constant=True, default=’NdMapping’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fc24810>)A string describing the data wrapped by the object.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.NdOverlay(overlays=None, kdims=None, **params)[source]#
Bases:
Overlayable
,UniformNdMapping
,CompositeOverlay
An NdOverlay allows a group of NdOverlay to be overlaid together. NdOverlay can be indexed out of an overlay and an overlay is an iterable that iterates over the contained layers.
Parameters inherited from:
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[Dimension(‘Element’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fc398d0>)List of dimensions the NdOverlay can be indexed by.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs NdOverlay of DynamicMaps into a single DynamicMap that returns an NdOverlay
Decollation allows packing a NdOverlay of DynamicMaps into a single DynamicMap that returns an NdOverlay of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns an NdOverlay
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, index=None, show_legend=False, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
- dimension: Dimension(s) to compute histogram on,
Falls back the plot dimensions by default.
num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram index (int, optional): Index of layer to apply hist to show_legend (bool, optional): Show legend in histogram
(don’t show legend by default).
- Returns:
AdjointLayout of element and histogram or just the histogram
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.Operation(*, dynamic, group, input_ranges, link_inputs, streams, name)[source]#
Bases:
ParameterizedFunction
An Operation process an Element or HoloMap at the level of individual elements or overlays. If a holomap is passed in as input, a processed holomap is returned as output where the individual elements have been transformed accordingly. An Operation may turn overlays in new elements or vice versa.
An Operation can be set to be dynamic, which will return a DynamicMap with a callback that will apply the operation dynamically. An Operation may also supply a list of Stream classes on a streams parameter, which can allow dynamic control over the parameters on the operation.
group
= param.String(allow_refs=False, default=’Operation’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fc741d0>)The group string used to identify the output of the Operation. By default this should match the operation name.
dynamic
= param.ObjectSelector(allow_refs=False, default=’default’, label=’Dynamic’, names={}, nested_refs=False, objects=[‘default’, True, False], rx=<param.reactive.reactive_ops object at 0x14fc75cd0>)Whether the operation should be applied dynamically when a specific frame is requested, specified as a Boolean. If set to ‘default’ the mode will be determined based on the input type, i.e. if the data is a DynamicMap it will stay dynamic.
input_ranges
= param.ClassSelector(allow_None=True, allow_refs=False, class_=(<class ‘dict’>, <class ‘tuple’>), default={}, label=’Input ranges’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fc741d0>)Ranges to be used for input normalization (if applicable) in a format appropriate for the Normalization.ranges parameter. By default, no normalization is applied. If key-wise normalization is required, a 2-tuple may be supplied where the first component is a Normalization.ranges list and the second component is Normalization.keys.
link_inputs
= param.Boolean(allow_refs=False, default=False, label=’Link inputs’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fc74e10>)If the operation is dynamic, whether or not linked streams should be transferred from the operation inputs for backends that support linked streams. For example if an operation is applied to a DynamicMap with an RangeXY, this switch determines whether the corresponding visualization should update this stream with range changes originating from the newly generated axes.
streams
= param.ClassSelector(allow_refs=False, class_=(<class ‘dict’>, <class ‘list’>), default=[], label=’Streams’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fc76950>)List of streams that are applied if dynamic=True, allowing for dynamic interaction with the plot.
- classmethod get_overlay_bounds(overlay)[source]#
Returns the extents if all the elements of an overlay agree on a consistent extents, otherwise raises an exception.
- classmethod get_overlay_label(overlay, default_label='')[source]#
Returns a label if all the elements of an overlay agree on a consistent label, otherwise returns the default label.
- classmethod instance(**params)[source]#
Return an instance of this class, copying parameters from any existing instance provided.
- class holoviews.core.Overlay(items=None, group=None, label=None, **params)[source]#
Bases:
ViewableTree
,CompositeOverlay
,Layoutable
,Overlayable
An Overlay consists of multiple Elements (potentially of heterogeneous type) presented one on top each other with a particular z-ordering.
Overlays along with elements constitute the only valid leaf types of a Layout and in fact extend the Layout structure. Overlays are constructed using the * operator (building an identical structure to the + operator).
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdims- clone(data=None, shared_data=True, new_type=None, link=True, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collate()[source]#
Collates any objects in the Overlay resolving any issues the recommended nesting structure.
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs Overlay of DynamicMaps into a single DynamicMap that returns an Overlay
Decollation allows packing an Overlay of DynamicMaps into a single DynamicMap that returns an Overlay of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns an Overlay
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a layer in the Overlay.
Get a particular layer in the Overlay using its path string or an integer index.
- Args:
identifier: Index or path string of the item to return default: Value to return if no item is found
- Returns:
The indexed layer of the Overlay
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
str(object=’’) -> str str(bytes_or_buffer[, encoding[, errors]]) -> str
Create a new string object from the given object. If encoding or errors is specified, then the object must expose a data buffer that will be decoded using the given encoding and error handler. Otherwise, returns the result of object.__str__() (if defined) or repr(object). encoding defaults to sys.getdefaultencoding(). errors defaults to ‘strict’.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, index=None, show_legend=False, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
- dimension: Dimension(s) to compute histogram on,
Falls back the plot dimensions by default.
num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram index (int, optional): Index of layer to apply hist to show_legend (bool, optional): Show legend in histogram
(don’t show legend by default).
- Returns:
AdjointLayout of element and histogram or just the histogram
- property label#
str(object=’’) -> str str(bytes_or_buffer[, encoding[, errors]]) -> str
Create a new string object from the given object. If encoding or errors is specified, then the object must expose a data buffer that will be decoded using the given encoding and error handler. Otherwise, returns the result of object.__str__() (if defined) or repr(object). encoding defaults to sys.getdefaultencoding(). errors defaults to ‘strict’.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
- class holoviews.core.SheetCoordinateSystem(bounds, xdensity, ydensity=None)[source]#
Bases:
object
Provides methods to allow conversion between sheet and matrix coordinates.
- closest_cell_center(x, y)[source]#
Given arbitrary sheet coordinates, return the sheet coordinates of the center of the closest unit.
- matrix2sheet(float_row, float_col)[source]#
Convert a floating-point location (float_row,float_col) in matrix coordinates to its corresponding location (x,y) in sheet coordinates.
Valid for scalar or array float_row and float_col.
Inverse of sheet2matrix().
- matrixidx2sheet(row, col)[source]#
Return (x,y) where x and y are the floating point coordinates of the center of the given matrix cell (row,col). If the matrix cell represents a 0.2 by 0.2 region, then the center location returned would be 0.1,0.1.
NOTE: This is NOT the strict mathematical inverse of sheet2matrixidx(), because sheet2matrixidx() discards all but the integer portion of the continuous matrix coordinate.
Valid only for scalar or array row and col.
- sheet2matrix(x, y)[source]#
Convert a point (x,y) in Sheet coordinates to continuous matrix coordinates.
Returns (float_row,float_col), where float_row corresponds to y, and float_col to x.
Valid for scalar or array x and y.
Note about Bounds For a Sheet with BoundingBox(points=((-0.5,-0.5),(0.5,0.5))) and density=3, x=-0.5 corresponds to float_col=0.0 and x=0.5 corresponds to float_col=3.0. float_col=3.0 is not inside the matrix representing this Sheet, which has the three columns (0,1,2). That is, x=-0.5 is inside the BoundingBox but x=0.5 is outside. Similarly, y=0.5 is inside (at row 0) but y=-0.5 is outside (at row 3) (it’s the other way round for y because the matrix row index increases as y decreases).
- sheet2matrixidx(x, y)[source]#
Convert a point (x,y) in sheet coordinates to the integer row and column index of the matrix cell in which that point falls, given a bounds and density. Returns (row,column).
Note that if coordinates along the right or bottom boundary are passed into this function, the returned matrix coordinate of the boundary will be just outside the matrix, because the right and bottom boundaries are exclusive.
Valid for scalar or array x and y.
- sheetcoordinates_of_matrixidx()[source]#
Return x,y where x is a vector of sheet coordinates representing the x-center of each matrix cell, and y represents the corresponding y-center of the cell.
- property xdensity#
The spacing between elements in an underlying matrix representation, in the x direction.
- property ydensity#
The spacing between elements in an underlying matrix representation, in the y direction.
- class holoviews.core.Tabular(data, kdims=None, vdims=None, **params)[source]#
Bases:
Element
Baseclass to give an elements providing an API to generate a tabular representation of the object.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdims- array(dimensions=None)[source]#
Convert dimension values to columnar array.
- Args:
dimensions: List of dimensions to return
- Returns:
Array of columns corresponding to each dimension
- cell_type(row, col)[source]#
Type of the table cell, either ‘data’ or ‘heading’
- Args:
row (int): Integer index of table row col (int): Integer index of table column
- Returns:
Type of the table cell, either ‘data’ or ‘heading’
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- closest(coords, **kwargs)[source]#
Snap list or dict of coordinates to closest position.
- Args:
coords: List of 1D or 2D coordinates **kwargs: Coordinates specified as keyword pairs
- Returns:
List of tuples of the snapped coordinates
- Raises:
NotImplementedError: Raised if snapping is not supported
- property cols#
Number of columns in table
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- pprint_cell(row, col)[source]#
Formatted contents of table cell.
- Args:
row (int): Integer index of table row col (int): Integer index of table column
- Returns:
Formatted table cell contents
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reduce(dimensions=None, function=None, spreadfn=None, **reduction)[source]#
Applies reduction along the specified dimension(s).
Allows reducing the values along one or more key dimension with the supplied function. Supports two signatures:
Reducing with a list of dimensions, e.g.:
ds.reduce([‘x’], np.mean)
Defining a reduction using keywords, e.g.:
ds.reduce(x=np.mean)
- Args:
- dimensions: Dimension(s) to apply reduction on
Defaults to all key dimensions
function: Reduction operation to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **reductions: Keyword argument defining reduction
Allows reduction to be defined as keyword pair of dimension and function
- Returns:
The element after reductions have been applied.
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- property rows#
Number of rows in table (including header)
- sample(samples=None, bounds=None, closest=False, **sample_values)[source]#
Samples values at supplied coordinates.
Allows sampling of element with a list of coordinates matching the key dimensions, returning a new object containing just the selected samples. Supports multiple signatures:
Sampling with a list of coordinates, e.g.:
ds.sample([(0, 0), (0.1, 0.2), …])
Sampling a range or grid of coordinates, e.g.:
1D: ds.sample(3) 2D: ds.sample((3, 3))
Sampling by keyword, e.g.:
ds.sample(x=0)
- Args:
samples: List of nd-coordinates to sample bounds: Bounds of the region to sample
Defined as two-tuple for 1D sampling and four-tuple for 2D sampling.
closest: Whether to snap to closest coordinates **kwargs: Coordinates specified as keyword pairs
Keywords of dimensions and scalar coordinates
- Returns:
Element containing the sampled coordinates
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.UniformNdMapping(initial_items=None, kdims=None, group=None, label=None, **params)[source]#
Bases:
NdMapping
A UniformNdMapping is a map of Dimensioned objects and is itself indexed over a number of specified dimensions. The dimension may be a spatial dimension (i.e., a ZStack), time (specifying a frame sequence) or any other combination of Dimensions.
UniformNdMapping objects can be sliced, sampled, reduced, overlaid and split along its and its containing Element’s dimensions. Subclasses should implement the appropriate slicing, sampling and reduction methods for their Dimensioned type.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.ViewableElement(data, kdims=None, vdims=None, **params)[source]#
Bases:
Dimensioned
A ViewableElement is a dimensioned datastructure that may be associated with a corresponding atomic visualization. An atomic visualization will display the data on a single set of axes (i.e. excludes multiple subplots that are displayed at once). The only new parameter introduced by ViewableElement is the title associated with the object for display.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’ViewableElement’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fce9f10>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.ViewableTree(items=None, identifier=None, parent=None, **kwargs)[source]#
Bases:
AttrTree
,Dimensioned
A ViewableTree is an AttrTree with Viewable objects as its leaf nodes. It combines the tree like data structure of a tree while extending it with the deep indexable properties of Dimensioned and LabelledData objects.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’ViewableTree’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x14fd18110>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
accessors
Module#
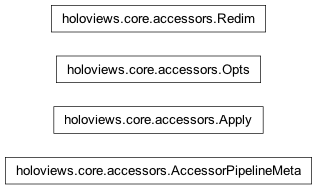
Module for accessor objects for viewable HoloViews objects.
- class holoviews.core.accessors.Apply(obj, mode=None)[source]#
Bases:
object
Utility to apply a function or operation to all viewable elements inside the object.
- aggregate(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Applies a aggregate function to all ViewableElements.
See
Dimensioned.aggregate()
andApply.__call__()
for more information.
- opts(*args, **kwargs)[source]#
Applies options to all ViewableElement objects.
See
Dimensioned.opts()
andApply.__call__()
for more information.
- reduce(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Applies a reduce function to all ViewableElement objects.
See
Dimensioned.opts()
andApply.__call__()
for more information.
- sample(samples=None, bounds=None, **kwargs)[source]#
Samples element values at supplied coordinates.
See
Dataset.sample()
andApply.__call__()
for more information.
boundingregion
Module#

Bounding regions and bounding boxes.
File originally part of the Topographica project.
- class holoviews.core.boundingregion.AARectangle(*points)[source]#
Bases:
object
Axis-aligned rectangle class.
Defines the smallest axis-aligned rectangle that encloses a set of points.
Usage: aar = AARectangle( (x1,y1),(x2,y2), … , (xN,yN) )
- class holoviews.core.boundingregion.BoundingBox(**args)[source]#
Bases:
BoundingRegion
A rectangular bounding box defined either by two points forming an axis-aligned rectangle (or simply a radius for a square).
- contains(x, y)[source]#
Returns true if the given point is contained within the bounding box, where all boundaries of the box are considered to be inclusive.
- contains_exclusive(x, y)[source]#
Return True if the given point is contained within the bounding box, where the bottom and right boundaries are considered exclusive.
- containsbb_exclusive(x)[source]#
Returns true if the given BoundingBox x is contained within the bounding box, where at least one of the boundaries of the box has to be exclusive.
- class holoviews.core.boundingregion.BoundingEllipse(**args)[source]#
Bases:
BoundingBox
Similar to BoundingBox, but the region is the ellipse inscribed within the rectangle.
- contains(x, y)[source]#
Returns true if the given point is contained within the bounding box, where all boundaries of the box are considered to be inclusive.
- contains_exclusive(x, y)[source]#
Return True if the given point is contained within the bounding box, where the bottom and right boundaries are considered exclusive.
- containsbb_exclusive(x)[source]#
Returns true if the given BoundingBox x is contained within the bounding box, where at least one of the boundaries of the box has to be exclusive.
decollate
Module#
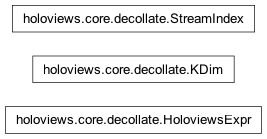
- holoviews.core.decollate.Expr#
alias of
HoloviewsExpr
- holoviews.core.decollate.KDimIndex#
alias of
KDim
- class holoviews.core.decollate.StreamIndex(index)#
Bases:
tuple
- count(value, /)#
Return number of occurrences of value.
- index#
Alias for field number 0
- holoviews.core.decollate.decollate(hvobj)[source]#
Decollate transforms a potentially nested dynamic HoloViews object into single DynamicMap that returns a non-dynamic HoloViews object. All nested streams in the input object are copied and attached to the resulting DynamicMap.
- Args:
hvobj: Holoviews object
- Returns:
DynamicMap
- holoviews.core.decollate.to_expr_extract_streams(hvobj, kdims, streams, original_streams, stream_mapping, container_key=None)[source]#
Build a HoloViewsExpr expression tree from a potentially nested dynamic HoloViews object, extracting the streams and replacing them with StreamIndex objects.
This function is recursive an assumes that initialize_dynamic has already been called on the input object.
- Args:
- hvobj: Element or DynamicMap or Layout
Potentially dynamic HoloViews object to represent as a HoloviewsExpr
- kdims: list of Dimensions
List that DynamicMap key-dimension objects should be added to
- streams: list of Stream
List that cloned extracted streams should be added to
- original_streams: list of Stream
List that original extracted streams should be added to
- stream_mapping: dict
dict to be populated with mappings from container keys to extracted Stream objects, as described by the Callable parameter of the same name.
- container_key: int or tuple
key into parent container that is associated to hvobj, or None if hvobj is not in a container
- Returns:
HoloviewsExpr expression representing hvobj if hvobj is dynamic. Otherwise, return hvobj itself
dimension
Module#

Provides Dimension objects for tracking the properties of a value, axis or map dimension. Also supplies the Dimensioned abstract baseclass for classes that accept Dimension values.
- class holoviews.core.dimension.Dimension(spec, **params)[source]#
Bases:
Parameterized
Dimension objects are used to specify some important general features that may be associated with a collection of values.
For instance, a Dimension may specify that a set of numeric values actually correspond to ‘Height’ (dimension name), in units of meters, with a descriptive label ‘Height of adult males’.
All dimensions object have a name that identifies them and a label containing a suitable description. If the label is not explicitly specified it matches the name.
These two parameters define the core identity of the dimension object and must match if two dimension objects are to be considered equivalent. All other parameters are considered optional metadata and are not used when testing for equality.
Unlike all the other parameters, these core parameters can be used to construct a Dimension object from a tuple. This format is sufficient to define an identical Dimension:
Dimension(‘a’, label=’Dimension A’) == Dimension((‘a’, ‘Dimension A’))
Everything else about a dimension is considered to reflect non-semantic preferences. Examples include the default value (which may be used in a visualization to set an initial slider position), how the value is to rendered as text (which may be used to specify the printed floating point precision) or a suitable range of values to consider for a particular analysis.
Units#
Full unit support with automated conversions are on the HoloViews roadmap. Once rich unit objects are supported, the unit (or more specifically the type of unit) will be part of the core dimension specification used to establish equality.
Until this feature is implemented, there are two auxiliary parameters that hold some partial information about the unit: the name of the unit and whether or not it is cyclic. The name of the unit is used as part of the pretty-printed representation and knowing whether it is cyclic is important for certain operations.
label
= param.String(allow_None=True, allow_refs=False, label=’Label’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x158c6f4d0>)Unrestricted label used to describe the dimension. A label should succinctly describe the dimension and may contain any characters, including Unicode and LaTeX expression.
cyclic
= param.Boolean(allow_refs=False, default=False, label=’Cyclic’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159649b90>)Whether the range of this feature is cyclic such that the maximum allowed value (defined by the range parameter) is continuous with the minimum allowed value.
default
= param.Parameter(allow_None=True, allow_refs=False, label=’Default’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x158c8b8d0>)Default value of the Dimension which may be useful for widget or other situations that require an initial or default value.
nodata
= param.Integer(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Nodata’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1595d9090>)Optional missing-data value for integer data. If non-None, data with this value will be replaced with NaN.
range
= param.Tuple(allow_refs=False, default=(None, None), label=’Range’, length=2, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159660510>)Specifies the minimum and maximum allowed values for a Dimension. None is used to represent an unlimited bound.
soft_range
= param.Tuple(allow_refs=False, default=(None, None), label=’Soft range’, length=2, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159663ed0>)Specifies a minimum and maximum reference value, which may be overridden by the data.
step
= param.Number(allow_None=True, allow_refs=False, inclusive_bounds=(True, True), label=’Step’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1595eaa90>)Optional floating point step specifying how frequently the underlying space should be sampled. May be used to define a discrete sampling over the range.
type
= param.Parameter(allow_None=True, allow_refs=False, label=’Type’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1595dad90>)Optional type associated with the Dimension values. The type may be an inbuilt constructor (such as int, str, float) or a custom class object.
unit
= param.String(allow_None=True, allow_refs=False, label=’Unit’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1595d9fd0>)Optional unit string associated with the Dimension. For instance, the string ‘m’ may be used represent units of meters and ‘s’ to represent units of seconds.
value_format
= param.Callable(allow_None=True, allow_refs=False, label=’Value format’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1595c7850>)Formatting function applied to each value before display.
values
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Values’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15965be10>)Optional specification of the allowed value set for the dimension that may also be used to retain a categorical ordering.
- clone(spec=None, **overrides)[source]#
Clones the Dimension with new parameters
Derive a new Dimension that inherits existing parameters except for the supplied, explicit overrides
- Args:
spec (tuple, optional): Dimension tuple specification **overrides: Dimension parameter overrides
- Returns:
Cloned Dimension object
- property pprint_label#
The pretty-printed label string for the Dimension
- pprint_value(value, print_unit=False)[source]#
Applies the applicable formatter to the value.
- Args:
value: Dimension value to format
- Returns:
Formatted dimension value
- pprint_value_string(value)[source]#
Pretty print the dimension value and unit with title_format
- Args:
value: Dimension value to format
- Returns:
Formatted dimension value string with unit
- property spec#
“Returns the Dimensions tuple specification
- Returns:
tuple: Dimension tuple specification
- class holoviews.core.dimension.Dimensioned(data, kdims=None, vdims=None, **params)[source]#
Bases:
LabelledData
Dimensioned is a base class that allows the data contents of a class to be associated with dimensions. The contents associated with dimensions may be partitioned into one of three types
- key dimensions: These are the dimensions that can be indexed via
the __getitem__ method. Dimension objects supporting key dimensions must support indexing over these dimensions and may also support slicing. This list ordering of dimensions describes the positional components of each multi-dimensional indexing operation.
For instance, if the key dimension names are ‘weight’ followed by ‘height’ for Dimensioned object ‘obj’, then obj[80,175] indexes a weight of 80 and height of 175.
Accessed using either kdims.
- value dimensions: These dimensions correspond to any data held
on the Dimensioned object not in the key dimensions. Indexing by value dimension is supported by dimension name (when there are multiple possible value dimensions); no slicing semantics is supported and all the data associated with that dimension will be returned at once. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either vdims.
- deep dimensions: These are dynamically computed dimensions that
belong to other Dimensioned objects that are nested in the data. Objects that support this should enable the _deep_indexable flag. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either ddims.
Dimensioned class support generalized methods for finding the range and type of values along a particular Dimension. The range method relies on the appropriate implementation of the dimension_values methods on subclasses.
The index of an arbitrary dimension is its positional index in the list of all dimensions, starting with the key dimensions, followed by the value dimensions and ending with the deep dimensions.
Parameters inherited from:
group
= param.String(allow_refs=False, constant=True, default=’Dimensioned’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1597f4750>)A string describing the data wrapped by the object.
cdims
= param.Dict(allow_refs=False, class_=<class ‘dict’>, default={}, label=’Cdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1597f59d0>)The constant dimensions defined as a dictionary of Dimension:value pairs providing additional dimension information about the object. Aliased with constant_dimensions.
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1597f4750>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
vdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Vdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1597f5d50>)The value dimensions defined as the list of dimensions used to describe the components of the data. If multiple value dimensions are supplied, a particular value dimension may be indexed by name after the key dimensions. Aliased with value_dimensions.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.dimension.LabelledData(data, id=None, plot_id=None, **params)[source]#
Bases:
Parameterized
LabelledData is a mix-in class designed to introduce the group and label parameters (and corresponding methods) to any class containing data. This class assumes that the core data contents will be held in the attribute called ‘data’.
Used together, group and label are designed to allow a simple and flexible means of addressing data. For instance, if you are collecting the heights of people in different demographics, you could specify the values of your objects as ‘Height’ and then use the label to specify the (sub)population.
In this scheme, one object may have the parameters set to [group=’Height’, label=’Children’] and another may use [group=’Height’, label=’Adults’].
Note: Another level of specification is implicit in the type (i.e class) of the LabelledData object. A full specification of a LabelledData object is therefore given by the tuple (<type>, <group>, label>). This additional level of specification is used in the traverse method.
Any strings can be used for the group and label, but it can be convenient to use a capitalized string of alphanumeric characters, in which case the keys used for matching in the matches and traverse method will correspond exactly to {type}.{group}.{label}. Otherwise the strings provided will be sanitized to be valid capitalized Python identifiers, which works fine but can sometimes be confusing.
group
= param.String(allow_refs=False, constant=True, default=’LabelledData’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15981e8d0>)A string describing the type of data contained by the object. By default this will typically mirror the class name.
label
= param.String(allow_refs=False, constant=True, default=’’, label=’Label’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15966d050>)Optional label describing the data, typically reflecting where or how it was measured. The label should allow a specific measurement or dataset to be referenced for a given group.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.dimension.ViewableElement(data, kdims=None, vdims=None, **params)[source]#
Bases:
Dimensioned
A ViewableElement is a dimensioned datastructure that may be associated with a corresponding atomic visualization. An atomic visualization will display the data on a single set of axes (i.e. excludes multiple subplots that are displayed at once). The only new parameter introduced by ViewableElement is the title associated with the object for display.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’ViewableElement’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15968f650>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.dimension.ViewableTree(items=None, identifier=None, parent=None, **kwargs)[source]#
Bases:
AttrTree
,Dimensioned
A ViewableTree is an AttrTree with Viewable objects as its leaf nodes. It combines the tree like data structure of a tree while extending it with the deep indexable properties of Dimensioned and LabelledData objects.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’ViewableTree’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1596a6d10>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
- holoviews.core.dimension.asdim(dimension)[source]#
Convert the input to a Dimension.
- Args:
dimension: tuple, dict or string type to convert to Dimension
- Returns:
A Dimension object constructed from the dimension spec. No copy is performed if the input is already a Dimension.
- holoviews.core.dimension.dimension_name(dimension)[source]#
Return the Dimension.name for a dimension-like object.
- Args:
dimension: Dimension or dimension string, tuple or dict
- Returns:
The name of the Dimension or what would be the name if the input as converted to a Dimension.
- holoviews.core.dimension.param_aliases(d)[source]#
Called from __setstate__ in LabelledData in order to load old pickles with outdated parameter names.
Warning: We want to keep pickle hacking to a minimum!
- holoviews.core.dimension.process_dimensions(kdims, vdims)[source]#
Converts kdims and vdims to Dimension objects.
- Args:
- kdims: List or single key dimension(s) specified as strings,
tuples dicts or Dimension objects.
- vdims: List or single value dimension(s) specified as strings,
tuples dicts or Dimension objects.
- Returns:
Dictionary containing kdims and vdims converted to Dimension objects:
{‘kdims’: [Dimension(‘x’)], ‘vdims’: [Dimension(‘y’)]
element
Module#

- class holoviews.core.element.Collator(data=None, **params)[source]#
Bases:
NdMapping
Collator is an NdMapping type which can merge any number of HoloViews components with whatever level of nesting by inserting the Collators key dimensions on the HoloMaps. If the items in the Collator do not contain HoloMaps they will be created. Collator also supports filtering of Tree structures and dropping of constant dimensions.
Parameters inherited from:
group
= param.String(allow_refs=False, default=’Collator’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1597b9450>)A string describing the data wrapped by the object.
vdims
= param.List(allow_refs=False, bounds=(0, 0), default=[], label=’Vdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159a04510>)Collator operates on HoloViews objects, if vdims are specified a value_transform function must also be supplied.
drop
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Drop’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159a05010>)List of dimensions to drop when collating data, specified as strings.
drop_constant
= param.Boolean(allow_refs=False, default=False, label=’Drop constant’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159a048d0>)Whether to demote any non-varying key dimensions to constant dimensions.
filters
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Filters’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159a04610>)List of paths to drop when collating data, specified as strings or tuples.
progress_bar
= param.Parameter(allow_None=True, allow_refs=False, label=’Progress bar’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1597fb1d0>)The progress bar instance used to report progress. Set to None to disable progress bars.
merge_type
= param.ClassSelector(allow_refs=False, class_=<class ‘holoviews.core.ndmapping.NdMapping’>, default=<class ‘holoviews.core.spaces.HoloMap’>, label=’Merge type’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159a05190>)value_transform
= param.Callable(allow_None=True, allow_refs=False, label=’Value transform’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159848e10>)If supplied the function will be applied on each Collator value during collation. This may be used to apply an operation to the data or load references from disk before they are collated into a displayable HoloViews object.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property static_dimensions#
Return all constant dimensions.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.element.CompositeOverlay(data, kdims=None, vdims=None, **params)[source]#
Bases:
ViewableElement
,Composable
CompositeOverlay provides a common baseclass for Overlay classes.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdims- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, index=None, show_legend=False, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
- dimension: Dimension(s) to compute histogram on,
Falls back the plot dimensions by default.
num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram index (int, optional): Index of layer to apply hist to show_legend (bool, optional): Show legend in histogram
(don’t show legend by default).
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.element.Dimensioned(data, kdims=None, vdims=None, **params)[source]#
Bases:
LabelledData
Dimensioned is a base class that allows the data contents of a class to be associated with dimensions. The contents associated with dimensions may be partitioned into one of three types
- key dimensions: These are the dimensions that can be indexed via
the __getitem__ method. Dimension objects supporting key dimensions must support indexing over these dimensions and may also support slicing. This list ordering of dimensions describes the positional components of each multi-dimensional indexing operation.
For instance, if the key dimension names are ‘weight’ followed by ‘height’ for Dimensioned object ‘obj’, then obj[80,175] indexes a weight of 80 and height of 175.
Accessed using either kdims.
- value dimensions: These dimensions correspond to any data held
on the Dimensioned object not in the key dimensions. Indexing by value dimension is supported by dimension name (when there are multiple possible value dimensions); no slicing semantics is supported and all the data associated with that dimension will be returned at once. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either vdims.
- deep dimensions: These are dynamically computed dimensions that
belong to other Dimensioned objects that are nested in the data. Objects that support this should enable the _deep_indexable flag. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either ddims.
Dimensioned class support generalized methods for finding the range and type of values along a particular Dimension. The range method relies on the appropriate implementation of the dimension_values methods on subclasses.
The index of an arbitrary dimension is its positional index in the list of all dimensions, starting with the key dimensions, followed by the value dimensions and ending with the deep dimensions.
Parameters inherited from:
group
= param.String(allow_refs=False, constant=True, default=’Dimensioned’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1599e6c90>)A string describing the data wrapped by the object.
cdims
= param.Dict(allow_refs=False, class_=<class ‘dict’>, default={}, label=’Cdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1599f1390>)The constant dimensions defined as a dictionary of Dimension:value pairs providing additional dimension information about the object. Aliased with constant_dimensions.
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1599e6c90>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
vdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Vdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1599f1290>)The value dimensions defined as the list of dimensions used to describe the components of the data. If multiple value dimensions are supplied, a particular value dimension may be indexed by name after the key dimensions. Aliased with value_dimensions.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.element.Element(data, kdims=None, vdims=None, **params)[source]#
Bases:
ViewableElement
,Composable
,Overlayable
Element is the atomic datastructure used to wrap some data with an associated visual representation, e.g. an element may represent a set of points, an image or a curve. Elements provide a common API for interacting with data of different types and define how the data map to a set of dimensions and how those map to the visual representation.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’Element’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159938790>)A string describing the data wrapped by the object.
- array(dimensions=None)[source]#
Convert dimension values to columnar array.
- Args:
dimensions: List of dimensions to return
- Returns:
Array of columns corresponding to each dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- closest(coords, **kwargs)[source]#
Snap list or dict of coordinates to closest position.
- Args:
coords: List of 1D or 2D coordinates **kwargs: Coordinates specified as keyword pairs
- Returns:
List of tuples of the snapped coordinates
- Raises:
NotImplementedError: Raised if snapping is not supported
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reduce(dimensions=None, function=None, spreadfn=None, **reduction)[source]#
Applies reduction along the specified dimension(s).
Allows reducing the values along one or more key dimension with the supplied function. Supports two signatures:
Reducing with a list of dimensions, e.g.:
ds.reduce([‘x’], np.mean)
Defining a reduction using keywords, e.g.:
ds.reduce(x=np.mean)
- Args:
- dimensions: Dimension(s) to apply reduction on
Defaults to all key dimensions
function: Reduction operation to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **reductions: Keyword argument defining reduction
Allows reduction to be defined as keyword pair of dimension and function
- Returns:
The element after reductions have been applied.
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- sample(samples=None, bounds=None, closest=False, **sample_values)[source]#
Samples values at supplied coordinates.
Allows sampling of element with a list of coordinates matching the key dimensions, returning a new object containing just the selected samples. Supports multiple signatures:
Sampling with a list of coordinates, e.g.:
ds.sample([(0, 0), (0.1, 0.2), …])
Sampling a range or grid of coordinates, e.g.:
1D: ds.sample(3) 2D: ds.sample((3, 3))
Sampling by keyword, e.g.:
ds.sample(x=0)
- Args:
samples: List of nd-coordinates to sample bounds: Bounds of the region to sample
Defined as two-tuple for 1D sampling and four-tuple for 2D sampling.
closest: Whether to snap to closest coordinates **kwargs: Coordinates specified as keyword pairs
Keywords of dimensions and scalar coordinates
- Returns:
Element containing the sampled coordinates
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.element.Element2D(data, kdims=None, vdims=None, **params)[source]#
Bases:
Element
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsextents
= param.Tuple(allow_refs=False, default=(None, None, None, None), label=’Extents’, length=4, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159a6e150>)Allows overriding the extents of the Element in 2D space defined as four-tuple defining the (left, bottom, right and top) edges.
- array(dimensions=None)[source]#
Convert dimension values to columnar array.
- Args:
dimensions: List of dimensions to return
- Returns:
Array of columns corresponding to each dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- closest(coords, **kwargs)[source]#
Snap list or dict of coordinates to closest position.
- Args:
coords: List of 1D or 2D coordinates **kwargs: Coordinates specified as keyword pairs
- Returns:
List of tuples of the snapped coordinates
- Raises:
NotImplementedError: Raised if snapping is not supported
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reduce(dimensions=None, function=None, spreadfn=None, **reduction)[source]#
Applies reduction along the specified dimension(s).
Allows reducing the values along one or more key dimension with the supplied function. Supports two signatures:
Reducing with a list of dimensions, e.g.:
ds.reduce([‘x’], np.mean)
Defining a reduction using keywords, e.g.:
ds.reduce(x=np.mean)
- Args:
- dimensions: Dimension(s) to apply reduction on
Defaults to all key dimensions
function: Reduction operation to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **reductions: Keyword argument defining reduction
Allows reduction to be defined as keyword pair of dimension and function
- Returns:
The element after reductions have been applied.
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- sample(samples=None, bounds=None, closest=False, **sample_values)[source]#
Samples values at supplied coordinates.
Allows sampling of element with a list of coordinates matching the key dimensions, returning a new object containing just the selected samples. Supports multiple signatures:
Sampling with a list of coordinates, e.g.:
ds.sample([(0, 0), (0.1, 0.2), …])
Sampling a range or grid of coordinates, e.g.:
1D: ds.sample(3) 2D: ds.sample((3, 3))
Sampling by keyword, e.g.:
ds.sample(x=0)
- Args:
samples: List of nd-coordinates to sample bounds: Bounds of the region to sample
Defined as two-tuple for 1D sampling and four-tuple for 2D sampling.
closest: Whether to snap to closest coordinates **kwargs: Coordinates specified as keyword pairs
Keywords of dimensions and scalar coordinates
- Returns:
Element containing the sampled coordinates
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.element.Element3D(data, kdims=None, vdims=None, **params)[source]#
Bases:
Element2D
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsextents
= param.Tuple(allow_refs=False, default=(None, None, None, None, None, None), label=’Extents’, length=6, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159a8f210>)Allows overriding the extents of the Element in 3D space defined as (xmin, ymin, zmin, xmax, ymax, zmax).
- array(dimensions=None)[source]#
Convert dimension values to columnar array.
- Args:
dimensions: List of dimensions to return
- Returns:
Array of columns corresponding to each dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- closest(coords, **kwargs)[source]#
Snap list or dict of coordinates to closest position.
- Args:
coords: List of 1D or 2D coordinates **kwargs: Coordinates specified as keyword pairs
- Returns:
List of tuples of the snapped coordinates
- Raises:
NotImplementedError: Raised if snapping is not supported
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reduce(dimensions=None, function=None, spreadfn=None, **reduction)[source]#
Applies reduction along the specified dimension(s).
Allows reducing the values along one or more key dimension with the supplied function. Supports two signatures:
Reducing with a list of dimensions, e.g.:
ds.reduce([‘x’], np.mean)
Defining a reduction using keywords, e.g.:
ds.reduce(x=np.mean)
- Args:
- dimensions: Dimension(s) to apply reduction on
Defaults to all key dimensions
function: Reduction operation to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **reductions: Keyword argument defining reduction
Allows reduction to be defined as keyword pair of dimension and function
- Returns:
The element after reductions have been applied.
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- sample(samples=None, bounds=None, closest=False, **sample_values)[source]#
Samples values at supplied coordinates.
Allows sampling of element with a list of coordinates matching the key dimensions, returning a new object containing just the selected samples. Supports multiple signatures:
Sampling with a list of coordinates, e.g.:
ds.sample([(0, 0), (0.1, 0.2), …])
Sampling a range or grid of coordinates, e.g.:
1D: ds.sample(3) 2D: ds.sample((3, 3))
Sampling by keyword, e.g.:
ds.sample(x=0)
- Args:
samples: List of nd-coordinates to sample bounds: Bounds of the region to sample
Defined as two-tuple for 1D sampling and four-tuple for 2D sampling.
closest: Whether to snap to closest coordinates **kwargs: Coordinates specified as keyword pairs
Keywords of dimensions and scalar coordinates
- Returns:
Element containing the sampled coordinates
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.element.GridSpace(initial_items=None, kdims=None, **params)[source]#
Bases:
Layoutable
,UniformNdMapping
Grids are distinct from Layouts as they ensure all contained elements to be of the same type. Unlike Layouts, which have integer keys, Grids usually have floating point keys, which correspond to a grid sampling in some two-dimensional space. This two-dimensional space may have to arbitrary dimensions, e.g. for 2D parameter spaces.
Parameters inherited from:
kdims
= param.List(allow_refs=False, bounds=(1, 2), default=[Dimension(‘X’), Dimension(‘Y’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159ab2350>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace
Decollation allows packing a GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a GridSpace
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- keys(full_grid=False)[source]#
Returns the keys of the GridSpace
- Args:
full_grid (bool, optional): Return full cross-product of keys
- Returns:
List of keys
- property label#
Label inherited from items
- property last#
The last of a GridSpace is another GridSpace constituted of the last of the individual elements. To access the elements by their X,Y position, either index the position directly or use the items() method.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property shape#
Returns the 2D shape of the GridSpace as (rows, cols).
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.element.HoloMap(initial_items=None, kdims=None, group=None, label=None, **params)[source]#
Bases:
Layoutable
,UniformNdMapping
,Overlayable
A HoloMap is an n-dimensional mapping of viewable elements or overlays. Each item in a HoloMap has an tuple key defining the values along each of the declared key dimensions, defining the discretely sampled space of values.
The visual representation of a HoloMap consists of the viewable objects inside the HoloMap which can be explored by varying one or more widgets mapping onto the key dimensions of the HoloMap.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- collate(merge_type=None, drop=None, drop_constant=False)[source]#
Collate allows reordering nested containers
Collation allows collapsing nested mapping types by merging their dimensions. In simple terms in merges nested containers into a single merged type.
In the simple case a HoloMap containing other HoloMaps can easily be joined in this way. However collation is particularly useful when the objects being joined are deeply nested, e.g. you want to join multiple Layouts recorded at different times, collation will return one Layout containing HoloMaps indexed by Time. Changing the merge_type will allow merging the outer Dimension into any other UniformNdMapping type.
- Args:
merge_type: Type of the object to merge with drop: List of dimensions to drop drop_constant: Drop constant dimensions automatically
- Returns:
Collated Layout or HoloMap
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs HoloMap of DynamicMaps into a single DynamicMap that returns an HoloMap
Decollation allows packing a HoloMap of DynamicMaps into a single DynamicMap that returns an HoloMap of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns an HoloMap
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- grid(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and lay out groups in grid
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a GridSpace.
Args: dimensions: Dimension/str or list
Dimension or list of dimensions to group by
- Returns:
GridSpace with supplied dimensions
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, individually=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of HoloMap and histograms or just the histograms
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- layout(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and lay out groups
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a NdLayout.
- Args:
dimensions: Dimension(s) to group by
- Returns:
NdLayout with supplied dimensions
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, **kwargs)[source]#
Applies simplified option definition returning a new object
Applies options defined in a flat format to the objects returned by the DynamicMap. If the options are to be set directly on the objects in the HoloMap a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- overlay(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and overlay each group
Groups data by supplied dimension(s) overlaying the groups along the dimension(s).
- Args:
dimensions: Dimension(s) of dimensions to group by
- Returns:
NdOverlay object(s) with supplied dimensions
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.element.Layout(items=None, identifier=None, parent=None, **kwargs)[source]#
Bases:
Layoutable
,ViewableTree
A Layout is an ViewableTree with ViewableElement objects as leaf values. Unlike ViewableTree, a Layout supports a rich display, displaying leaf items in a grid style layout. In addition to the usual ViewableTree indexing, Layout supports indexing of items by their row and column index in the layout.
The maximum number of columns in such a layout may be controlled with the cols method.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’Layout’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159b03450>)A string describing the data wrapped by the object.
- cols(ncols)[source]#
Sets the maximum number of columns in the NdLayout.
Any items beyond the set number of cols will flow onto a new row. The number of columns control the indexing and display semantics of the NdLayout.
- Args:
ncols (int): Number of columns to set on the NdLayout
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs Layout of DynamicMaps into a single DynamicMap that returns a Layout
Decollation allows packing a Layout of DynamicMaps into a single DynamicMap that returns a Layout of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a Layout
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- property shape#
Tuple indicating the number of rows and columns in the Layout.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
- class holoviews.core.element.NdLayout(initial_items=None, kdims=None, **params)[source]#
Bases:
Layoutable
,UniformNdMapping
NdLayout is a UniformNdMapping providing an n-dimensional data structure to display the contained Elements and containers in a layout. Using the cols method the NdLayout can be rearranged with the desired number of columns.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- cols(ncols)[source]#
Sets the maximum number of columns in the NdLayout.
Any items beyond the set number of cols will flow onto a new row. The number of columns control the indexing and display semantics of the NdLayout.
- Args:
ncols (int): Number of columns to set on the NdLayout
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- grid_items()[source]#
Compute a dict of {(row,column): (key, value)} elements from the current set of items and specified number of columns.
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns another NdLayout constituted of the last views of the individual elements (if they are maps).
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property shape#
Tuple indicating the number of rows and columns in the NdLayout.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.element.NdMapping(initial_items=None, kdims=None, **params)[source]#
Bases:
MultiDimensionalMapping
NdMapping supports the same indexing semantics as MultiDimensionalMapping but also supports slicing semantics.
Slicing semantics on an NdMapping is dependent on the ordering semantics of the keys. As MultiDimensionalMapping sort the keys, a slice on an NdMapping is effectively a way of filtering out the keys that are outside the slice range.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sortgroup
= param.String(allow_refs=False, constant=True, default=’NdMapping’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159b5ca10>)A string describing the data wrapped by the object.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.element.NdOverlay(overlays=None, kdims=None, **params)[source]#
Bases:
Overlayable
,UniformNdMapping
,CompositeOverlay
An NdOverlay allows a group of NdOverlay to be overlaid together. NdOverlay can be indexed out of an overlay and an overlay is an iterable that iterates over the contained layers.
Parameters inherited from:
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[Dimension(‘Element’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159b72e50>)List of dimensions the NdOverlay can be indexed by.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs NdOverlay of DynamicMaps into a single DynamicMap that returns an NdOverlay
Decollation allows packing a NdOverlay of DynamicMaps into a single DynamicMap that returns an NdOverlay of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns an NdOverlay
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, index=None, show_legend=False, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
- dimension: Dimension(s) to compute histogram on,
Falls back the plot dimensions by default.
num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram index (int, optional): Index of layer to apply hist to show_legend (bool, optional): Show legend in histogram
(don’t show legend by default).
- Returns:
AdjointLayout of element and histogram or just the histogram
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.element.Tabular(data, kdims=None, vdims=None, **params)[source]#
Bases:
Element
Baseclass to give an elements providing an API to generate a tabular representation of the object.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdims- array(dimensions=None)[source]#
Convert dimension values to columnar array.
- Args:
dimensions: List of dimensions to return
- Returns:
Array of columns corresponding to each dimension
- cell_type(row, col)[source]#
Type of the table cell, either ‘data’ or ‘heading’
- Args:
row (int): Integer index of table row col (int): Integer index of table column
- Returns:
Type of the table cell, either ‘data’ or ‘heading’
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- closest(coords, **kwargs)[source]#
Snap list or dict of coordinates to closest position.
- Args:
coords: List of 1D or 2D coordinates **kwargs: Coordinates specified as keyword pairs
- Returns:
List of tuples of the snapped coordinates
- Raises:
NotImplementedError: Raised if snapping is not supported
- property cols#
Number of columns in table
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- pprint_cell(row, col)[source]#
Formatted contents of table cell.
- Args:
row (int): Integer index of table row col (int): Integer index of table column
- Returns:
Formatted table cell contents
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reduce(dimensions=None, function=None, spreadfn=None, **reduction)[source]#
Applies reduction along the specified dimension(s).
Allows reducing the values along one or more key dimension with the supplied function. Supports two signatures:
Reducing with a list of dimensions, e.g.:
ds.reduce([‘x’], np.mean)
Defining a reduction using keywords, e.g.:
ds.reduce(x=np.mean)
- Args:
- dimensions: Dimension(s) to apply reduction on
Defaults to all key dimensions
function: Reduction operation to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
- **reductions: Keyword argument defining reduction
Allows reduction to be defined as keyword pair of dimension and function
- Returns:
The element after reductions have been applied.
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- property rows#
Number of rows in table (including header)
- sample(samples=None, bounds=None, closest=False, **sample_values)[source]#
Samples values at supplied coordinates.
Allows sampling of element with a list of coordinates matching the key dimensions, returning a new object containing just the selected samples. Supports multiple signatures:
Sampling with a list of coordinates, e.g.:
ds.sample([(0, 0), (0.1, 0.2), …])
Sampling a range or grid of coordinates, e.g.:
1D: ds.sample(3) 2D: ds.sample((3, 3))
Sampling by keyword, e.g.:
ds.sample(x=0)
- Args:
samples: List of nd-coordinates to sample bounds: Bounds of the region to sample
Defined as two-tuple for 1D sampling and four-tuple for 2D sampling.
closest: Whether to snap to closest coordinates **kwargs: Coordinates specified as keyword pairs
Keywords of dimensions and scalar coordinates
- Returns:
Element containing the sampled coordinates
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.element.ViewableElement(data, kdims=None, vdims=None, **params)[source]#
Bases:
Dimensioned
A ViewableElement is a dimensioned datastructure that may be associated with a corresponding atomic visualization. An atomic visualization will display the data on a single set of axes (i.e. excludes multiple subplots that are displayed at once). The only new parameter introduced by ViewableElement is the title associated with the object for display.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’ViewableElement’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159bcf310>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
io
Module#

Module defining input/output interfaces to HoloViews.
There are two components for input/output:
- Exporters: Process (composite) HoloViews objects one at a time. For
instance, an exporter may render a HoloViews object as a svg or perhaps pickle it.
- Archives: A collection of HoloViews objects that are first collected
then processed together. For instance, collecting HoloViews objects for a report then generating a PDF or collecting HoloViews objects to dump to HDF5.
- class holoviews.core.io.Archive(*, exporters, name)[source]#
Bases:
Parameterized
An Archive is a means to collect and store a collection of HoloViews objects in any number of different ways. Examples of possible archives:
Generating tar or zip files (compressed or uncompressed).
Collating a report or document (e.g. PDF, HTML, LaTex).
Storing a collection of HoloViews objects to a database or HDF5.
exporters
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Exporters’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159f974d0>)The exporter functions used to convert HoloViews objects into the appropriate format(s).
- class holoviews.core.io.Deserializer(*, deserializer, name)[source]#
Bases:
Importer
A generic importer that supports any arbitrary de-serializer.
deserializer
= param.Callable(allow_refs=False, label=’Deserializer’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a8cd310>)The deserializer function, set to Store.load by default. The deserializer should take a file-like object that can be read from until the first object has been deserialized. If the file has not been exhausted, the deserializer should be able to continue parsing and loading objects. Any suitable deserializer may be used. For instance, pickle.load may be used although this will not load customized options.
- deserializer(filename)[source]#
Equivalent to pickle.load except that the HoloViews trees is restored appropriately.
- class holoviews.core.io.Exporter(*, info_fn, key_fn, name)[source]#
Bases:
ParameterizedFunction
An Exporter is a parameterized function that accepts a HoloViews object and converts it to a new some new format. This mechanism is designed to be very general so here are a few examples:
Pickling: Native Python, supported by HoloViews. Rendering: Any plotting backend may be used (default uses matplotlib) Storage: Saving to a database (e.g. SQL), HDF5 etc.
key_fn
= param.Callable(allow_None=True, allow_refs=False, label=’Key fn’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a917250>)Function that generates the metadata key from the HoloViews object being saved. The metadata key is a single high-dimensional key of values associated with dimension labels. The returned dictionary must have string keys and simple literals that may be conveniently used for dictionary-style indexing. Returns an empty dictionary by default.
info_fn
= param.Callable(allow_refs=False, label=’Info fn’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a915bd0>)Function that generates additional metadata information from the HoloViews object being saved. Unlike metadata keys, the information returned may be unsuitable for use as a key index and may include entries such as the object’s repr. Regardless, the info metadata should still only contain items that will be quick to load and inspect.
- classmethod encode(entry)[source]#
Classmethod that applies conditional encoding based on mime-type. Given an entry as returned by __call__ return the data in the appropriate encoding.
- classmethod instance(**params)[source]#
Return an instance of this class, copying parameters from any existing instance provided.
- save(obj, basename, fmt=None, key=None, info=None, **kwargs)[source]#
Similar to the call method except saves exporter data to disk into a file with specified basename. For exporters that support multiple formats, the fmt argument may also be supplied (which typically corresponds to the file-extension).
The supplied metadata key and info dictionaries will be used to update the output of the relevant key and info functions which is then saved (if supported).
- class holoviews.core.io.FileArchive(*, archive_format, dimension_formatter, export_name, filename_formatter, flush_archive, max_filename, object_formatter, pack, root, timestamp_format, unique_name, exporters, name)[source]#
Bases:
Archive
A file archive stores files on disk, either unpacked in a directory or in an archive format (e.g. a zip file).
exporters
= param.List(allow_refs=False, bounds=(0, None), default=[<class ‘holoviews.core.io.Pickler’>], label=’Exporters’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90fc90>)The exporter functions used to convert HoloViews objects into the appropriate format(s).
dimension_formatter
= param.String(allow_refs=False, default=’{name}_{range}’, label=’Dimension formatter’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90ca50>)A string formatter for the output file based on the supplied HoloViews objects dimension names and values. Valid fields are the {name}, {range} and {unit} of the dimensions.
object_formatter
= param.Callable(allow_refs=False, label=’Object formatter’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90ec10>)Callable that given an object returns a string suitable for inclusion in file and directory names. This is what generates the value used in the {obj} field of the filename formatter.
filename_formatter
= param.String(allow_refs=False, default=’{dimensions},{obj}’, label=’Filename formatter’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90ca50>)A string formatter for output filename based on the HoloViews object that is being rendered to disk. The available fields are the {type}, {group}, {label}, {obj} of the holoviews object added to the archive as well as {timestamp}, {obj} and {SHA}. The {timestamp} is the export timestamp using timestamp_format, {obj} is the object representation as returned by object_formatter and {SHA} is the SHA of the {obj} value used to compress it into a shorter string.
timestamp_format
= param.String(allow_refs=False, default=’%Y_%m_%d-%H_%M_%S’, label=’Timestamp format’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90d2d0>)The timestamp format that will be substituted for the {timestamp} field in the export name.
root
= param.String(allow_refs=False, default=’.’, label=’Root’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90cc50>)The root directory in which the output directory is located. May be an absolute or relative path.
archive_format
= param.ObjectSelector(allow_refs=False, default=’zip’, label=’Archive format’, names={}, nested_refs=False, objects=[‘zip’, ‘tar’], rx=<param.reactive.reactive_ops object at 0x15a90d250>)The archive format to use if there are multiple files and pack is set to True. Supported formats include ‘zip’ and ‘tar’.
pack
= param.Boolean(allow_refs=False, default=False, label=’Pack’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90ca50>)Whether or not to pack to contents into the specified archive format. If pack is False, the contents will be output to a directory. Note that if there is only a single file in the archive, no packing will occur and no directory is created. Instead, the file is treated as a single-file archive.
export_name
= param.String(allow_refs=False, default=’{timestamp}’, label=’Export name’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90d2d0>)The name assigned to the overall export. If an archive file is used, this is the correspond filename (e.g. of the exporter zip file). Alternatively, if unpack=False, this is the name of the output directory. Lastly, for archives of a single file, this is the basename of the output file. The {timestamp} field is available to include the timestamp at the time of export in the chosen timestamp format.
unique_name
= param.Boolean(allow_refs=False, default=False, label=’Unique name’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90e950>)Whether the export name should be made unique with a numeric suffix. If set to False, any existing export of the same name will be removed and replaced.
max_filename
= param.Integer(allow_refs=False, bounds=(0, None), default=100, inclusive_bounds=(True, True), label=’Max filename’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90da90>)Maximum length to enforce on generated filenames. 100 is the practical maximum for zip and tar file generation, but you may wish to use a lower value to avoid long filenames.
flush_archive
= param.Boolean(allow_refs=False, default=True, label=’Flush archive’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a90d250>)Flushed the contents of the archive after export.
- add(obj=None, filename=None, data=None, info=None, **kwargs)[source]#
If a filename is supplied, it will be used. Otherwise, a filename will be generated from the supplied object. Note that if the explicit filename uses the {timestamp} field, it will be formatted upon export.
The data to be archived is either supplied explicitly as ‘data’ or automatically rendered from the object.
- object_formatter()[source]#
Simple name_generator designed for HoloViews objects.
Objects are labeled with {group}-{label} for each nested object, based on a depth-first search. Adjacent objects with identical representations yield only a single copy of the representation, to avoid long names for the common case of a container whose element(s) share the same group and label.
- class holoviews.core.io.Importer(*, name)[source]#
Bases:
ParameterizedFunction
An Importer is a parameterized function that accepts some data in some format and returns a HoloViews object. This mechanism is designed to be very general so here are a few examples:
Unpickling: Native Python, supported by HoloViews. Servers: Loading data over a network connection. Storage: Loading from a database (e.g. SQL), HDF5 etc.
- class holoviews.core.io.Pickler(*, compress, protocol, info_fn, key_fn, name)[source]#
Bases:
Exporter
The recommended pickler for serializing HoloViews object to a .hvz file (a simple zip archive of pickle files). In addition to the functionality offered by Store.dump and Store.load, this file format offers three additional features:
Optional (zip) compression.
Ability to save and load components of a Layout independently.
Support for metadata per saved component.
The output file with the .hvz file extension is simply a zip archive containing pickled HoloViews objects.
Parameters inherited from:
holoviews.core.io.Exporter
: key_fn, info_fnprotocol
= param.Integer(allow_refs=False, default=2, inclusive_bounds=(True, True), label=’Protocol’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aa56a90>)The pickling protocol where 0 is ASCII, 1 supports old Python versions and 2 is efficient for new style classes.
compress
= param.Boolean(allow_refs=False, default=True, label=’Compress’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a94e290>)Whether compression is enabled or not
- classmethod encode(entry)[source]#
Classmethod that applies conditional encoding based on mime-type. Given an entry as returned by __call__ return the data in the appropriate encoding.
- classmethod instance(**params)[source]#
Return an instance of this class, copying parameters from any existing instance provided.
- save(obj, filename, key=None, info=None, **kwargs)[source]#
Similar to the call method except saves exporter data to disk into a file with specified basename. For exporters that support multiple formats, the fmt argument may also be supplied (which typically corresponds to the file-extension).
The supplied metadata key and info dictionaries will be used to update the output of the relevant key and info functions which is then saved (if supported).
- class holoviews.core.io.Reference(*, name)[source]#
Bases:
Parameterized
A Reference allows access to an object to be deferred until it is needed in the appropriate context. References are used by Collector to capture the state of an object at collection time.
One particularly important property of references is that they should be pickleable. This means that you can pickle Collectors so that you can unpickle them in different environments and still collect from the required object.
A Reference only needs to have a resolved_type property and a resolve method. The constructor will take some specification of where to find the target object (may be the object itself).
- resolve(container=None)[source]#
Return the referenced object. Optionally, a container may be passed in from which the object is to be resolved.
- property resolved_type#
Returns the type of the object resolved by this references. If multiple types are possible, the return is a tuple of types.
- class holoviews.core.io.Serializer(*, file_ext, mime_type, serializer, info_fn, key_fn, name)[source]#
Bases:
Exporter
A generic exporter that supports any arbitrary serializer
Parameters inherited from:
holoviews.core.io.Exporter
: key_fn, info_fnserializer
= param.Callable(allow_refs=False, label=’Serializer’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aa58f50>)The serializer function, set to Store.dumps by default. The serializer should take an object and output a serialization as a string or byte stream. Any suitable serializer may be used. For instance, pickle.dumps may be used although this will not save customized options.
mime_type
= param.String(allow_None=True, allow_refs=False, default=’application/python-pickle’, label=’Mime type’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aa5b750>)The mime-type associated with the serializer (if applicable).
file_ext
= param.String(allow_refs=False, default=’pkl’, label=’File ext’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aa59190>)The file extension associated with the corresponding file format (if applicable).
- classmethod encode(entry)[source]#
Classmethod that applies conditional encoding based on mime-type. Given an entry as returned by __call__ return the data in the appropriate encoding.
- classmethod instance(**params)[source]#
Return an instance of this class, copying parameters from any existing instance provided.
- save(obj, filename, info=None, key=None, **kwargs)[source]#
Similar to the call method except saves exporter data to disk into a file with specified basename. For exporters that support multiple formats, the fmt argument may also be supplied (which typically corresponds to the file-extension).
The supplied metadata key and info dictionaries will be used to update the output of the relevant key and info functions which is then saved (if supported).
- class holoviews.core.io.Unpickler(*, name)[source]#
Bases:
Importer
The inverse of Pickler used to load the .hvz file format which is simply a zip archive of pickle objects.
Unlike a regular pickle file, info and key metadata as well as individual components of a Layout may be loaded without needing to load the entire file into memory.
The components that may be individually loaded may be found using the entries method.
- collect(files, drop=None, metadata=True)[source]#
Given a list or NdMapping type containing file paths return a Layout of Collators, which can be called to load a given set of files using the current Importer.
If supplied as a list each file is expected to disambiguate itself with contained metadata. If an NdMapping type is supplied additional key dimensions may be supplied as long as they do not clash with the file metadata. Any key dimension may be dropped by name by supplying a drop argument.
- holoviews.core.io.sanitizer(name, replacements=None)[source]#
String sanitizer to avoid problematic characters in filenames.
- holoviews.core.io.simple_name_generator(obj)[source]#
Simple name_generator designed for HoloViews objects.
Objects are labeled with {group}-{label} for each nested object, based on a depth-first search. Adjacent objects with identical representations yield only a single copy of the representation, to avoid long names for the common case of a container whose element(s) share the same group and label.
layout
Module#

Supplies Pane, Layout, NdLayout and AdjointLayout. Pane extends View to allow multiple Views to be presented side-by-side in a NdLayout. An AdjointLayout allows one or two Views to be adjoined to a primary View to act as supplementary elements.
- class holoviews.core.layout.AdjointLayout(data, **params)[source]#
Bases:
Layoutable
,Dimensioned
An AdjointLayout provides a convenient container to lay out some marginal plots next to a primary plot. This is often useful to display the marginal distributions of a plot next to the primary plot. AdjointLayout accepts a list of up to three elements, which are laid out as follows with the names ‘main’, ‘top’ and ‘right’:
3 | ||___________|___| | | | 1: main | | | 2: right | 1 | 2 | 3: top | | | |___________|___|
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: group, cdims, vdimskdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[Dimension(‘AdjointLayout’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aa21710>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Applies to the main object in the AdjointLayout.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get(key, default=None)[source]#
Returns the viewable corresponding to the supplied string or integer based key.
- Args:
key: Numeric or string index: 0) ‘main’ 1) ‘right’ 2) ‘top’ default: Value returned if key not found
- Returns:
Indexed value or supplied default
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from main element
- property label#
Label inherited from main element
- property main#
Returns the main element in the AdjointLayout
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to child up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- property right#
Returns the right marginal element in the AdjointLayout
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property top#
Returns the top marginal element in the AdjointLayout
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.layout.Dimensioned(data, kdims=None, vdims=None, **params)[source]#
Bases:
LabelledData
Dimensioned is a base class that allows the data contents of a class to be associated with dimensions. The contents associated with dimensions may be partitioned into one of three types
- key dimensions: These are the dimensions that can be indexed via
the __getitem__ method. Dimension objects supporting key dimensions must support indexing over these dimensions and may also support slicing. This list ordering of dimensions describes the positional components of each multi-dimensional indexing operation.
For instance, if the key dimension names are ‘weight’ followed by ‘height’ for Dimensioned object ‘obj’, then obj[80,175] indexes a weight of 80 and height of 175.
Accessed using either kdims.
- value dimensions: These dimensions correspond to any data held
on the Dimensioned object not in the key dimensions. Indexing by value dimension is supported by dimension name (when there are multiple possible value dimensions); no slicing semantics is supported and all the data associated with that dimension will be returned at once. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either vdims.
- deep dimensions: These are dynamically computed dimensions that
belong to other Dimensioned objects that are nested in the data. Objects that support this should enable the _deep_indexable flag. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either ddims.
Dimensioned class support generalized methods for finding the range and type of values along a particular Dimension. The range method relies on the appropriate implementation of the dimension_values methods on subclasses.
The index of an arbitrary dimension is its positional index in the list of all dimensions, starting with the key dimensions, followed by the value dimensions and ending with the deep dimensions.
Parameters inherited from:
group
= param.String(allow_refs=False, constant=True, default=’Dimensioned’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aaf0cd0>)A string describing the data wrapped by the object.
cdims
= param.Dict(allow_refs=False, class_=<class ‘dict’>, default={}, label=’Cdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aae6fd0>)The constant dimensions defined as a dictionary of Dimension:value pairs providing additional dimension information about the object. Aliased with constant_dimensions.
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aaf3cd0>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
vdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Vdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aae5090>)The value dimensions defined as the list of dimensions used to describe the components of the data. If multiple value dimensions are supplied, a particular value dimension may be indexed by name after the key dimensions. Aliased with value_dimensions.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.layout.Empty(*, cdims, kdims, vdims, group, label, name)[source]#
Bases:
Dimensioned
,Composable
Empty may be used to define an empty placeholder in a Layout. It can be placed in a Layout just like any regular Element and container type via the + operator or by passing it to the Layout constructor as a part of a list.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, default=’Empty’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ab19f50>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.layout.Layout(items=None, identifier=None, parent=None, **kwargs)[source]#
Bases:
Layoutable
,ViewableTree
A Layout is an ViewableTree with ViewableElement objects as leaf values. Unlike ViewableTree, a Layout supports a rich display, displaying leaf items in a grid style layout. In addition to the usual ViewableTree indexing, Layout supports indexing of items by their row and column index in the layout.
The maximum number of columns in such a layout may be controlled with the cols method.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’Layout’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ab2fad0>)A string describing the data wrapped by the object.
- cols(ncols)[source]#
Sets the maximum number of columns in the NdLayout.
Any items beyond the set number of cols will flow onto a new row. The number of columns control the indexing and display semantics of the NdLayout.
- Args:
ncols (int): Number of columns to set on the NdLayout
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs Layout of DynamicMaps into a single DynamicMap that returns a Layout
Decollation allows packing a Layout of DynamicMaps into a single DynamicMap that returns a Layout of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a Layout
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- property shape#
Tuple indicating the number of rows and columns in the Layout.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
- class holoviews.core.layout.NdLayout(initial_items=None, kdims=None, **params)[source]#
Bases:
Layoutable
,UniformNdMapping
NdLayout is a UniformNdMapping providing an n-dimensional data structure to display the contained Elements and containers in a layout. Using the cols method the NdLayout can be rearranged with the desired number of columns.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- cols(ncols)[source]#
Sets the maximum number of columns in the NdLayout.
Any items beyond the set number of cols will flow onto a new row. The number of columns control the indexing and display semantics of the NdLayout.
- Args:
ncols (int): Number of columns to set on the NdLayout
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- grid_items()[source]#
Compute a dict of {(row,column): (key, value)} elements from the current set of items and specified number of columns.
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns another NdLayout constituted of the last views of the individual elements (if they are maps).
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property shape#
Tuple indicating the number of rows and columns in the NdLayout.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.layout.NdMapping(initial_items=None, kdims=None, **params)[source]#
Bases:
MultiDimensionalMapping
NdMapping supports the same indexing semantics as MultiDimensionalMapping but also supports slicing semantics.
Slicing semantics on an NdMapping is dependent on the ordering semantics of the keys. As MultiDimensionalMapping sort the keys, a slice on an NdMapping is effectively a way of filtering out the keys that are outside the slice range.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sortgroup
= param.String(allow_refs=False, constant=True, default=’NdMapping’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ab6c8d0>)A string describing the data wrapped by the object.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.layout.UniformNdMapping(initial_items=None, kdims=None, group=None, label=None, **params)[source]#
Bases:
NdMapping
A UniformNdMapping is a map of Dimensioned objects and is itself indexed over a number of specified dimensions. The dimension may be a spatial dimension (i.e., a ZStack), time (specifying a frame sequence) or any other combination of Dimensions.
UniformNdMapping objects can be sliced, sampled, reduced, overlaid and split along its and its containing Element’s dimensions. Subclasses should implement the appropriate slicing, sampling and reduction methods for their Dimensioned type.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.layout.ViewableElement(data, kdims=None, vdims=None, **params)[source]#
Bases:
Dimensioned
A ViewableElement is a dimensioned datastructure that may be associated with a corresponding atomic visualization. An atomic visualization will display the data on a single set of axes (i.e. excludes multiple subplots that are displayed at once). The only new parameter introduced by ViewableElement is the title associated with the object for display.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’ViewableElement’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15abad790>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.layout.ViewableTree(items=None, identifier=None, parent=None, **kwargs)[source]#
Bases:
AttrTree
,Dimensioned
A ViewableTree is an AttrTree with Viewable objects as its leaf nodes. It combines the tree like data structure of a tree while extending it with the deep indexable properties of Dimensioned and LabelledData objects.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’ViewableTree’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15abd7790>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
ndmapping
Module#

Supplies MultiDimensionalMapping and NdMapping which are multi-dimensional map types. The former class only allows indexing whereas the latter also enables slicing over multiple dimension ranges.
- class holoviews.core.ndmapping.MultiDimensionalMapping(initial_items=None, kdims=None, **params)[source]#
Bases:
Dimensioned
An MultiDimensionalMapping is a Dimensioned mapping (like a dictionary or array) that uses fixed-length multidimensional keys. This behaves like a sparse N-dimensional array that does not require a dense sampling over the multidimensional space.
If the underlying value for each (key, value) pair also supports indexing (such as a dictionary, array, or list), fully qualified (deep) indexing may be used from the top level, with the first N dimensions of the index selecting a particular Dimensioned object and the remaining dimensions indexing into that object.
For instance, for a MultiDimensionalMapping with dimensions “Year” and “Month” and underlying values that are 2D floating-point arrays indexed by (r,c), a 2D array may be indexed with x[2000,3] and a single floating-point number may be indexed as x[2000,3,1,9].
In practice, this class is typically only used as an abstract base class, because the NdMapping subclass extends it with a range of useful slicing methods for selecting subsets of the data. Even so, keeping the slicing support separate from the indexing and data storage methods helps make both classes easier to understand.
Parameters inherited from:
group
= param.String(allow_refs=False, constant=True, default=’MultiDimensionalMapping’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b409450>)A string describing the data wrapped by the object.
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[Dimension(‘Default’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b4c5b10>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
vdims
= param.List(allow_refs=False, bounds=(0, 0), constant=True, default=[], label=’Vdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b4c4150>)The value dimensions defined as the list of dimensions used to describe the components of the data. If multiple value dimensions are supplied, a particular value dimension may be indexed by name after the key dimensions. Aliased with value_dimensions.
sort
= param.Boolean(allow_refs=False, default=True, label=’Sort’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b4c5550>)Whether the items should be sorted in the constructor.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.ndmapping.NdMapping(initial_items=None, kdims=None, **params)[source]#
Bases:
MultiDimensionalMapping
NdMapping supports the same indexing semantics as MultiDimensionalMapping but also supports slicing semantics.
Slicing semantics on an NdMapping is dependent on the ordering semantics of the keys. As MultiDimensionalMapping sort the keys, a slice on an NdMapping is effectively a way of filtering out the keys that are outside the slice range.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sortgroup
= param.String(allow_refs=False, constant=True, default=’NdMapping’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b4d4690>)A string describing the data wrapped by the object.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.ndmapping.UniformNdMapping(initial_items=None, kdims=None, group=None, label=None, **params)[source]#
Bases:
NdMapping
A UniformNdMapping is a map of Dimensioned objects and is itself indexed over a number of specified dimensions. The dimension may be a spatial dimension (i.e., a ZStack), time (specifying a frame sequence) or any other combination of Dimensions.
UniformNdMapping objects can be sliced, sampled, reduced, overlaid and split along its and its containing Element’s dimensions. Subclasses should implement the appropriate slicing, sampling and reduction methods for their Dimensioned type.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.ndmapping.item_check(enabled)[source]#
Bases:
object
Context manager to allow creating NdMapping types without performing the usual item_checks, providing significant speedups when there are a lot of items. Should only be used when both keys and values are guaranteed to be the right type, as is the case for many internal operations.
- class holoviews.core.ndmapping.sorted_context(enabled)[source]#
Bases:
object
Context manager to temporarily disable sorting on NdMapping types. Retains the current sort order, which can be useful as an optimization on NdMapping instances where sort=True but the items are already known to have been sorted.
operation
Module#

Operations manipulate Elements, HoloMaps and Layouts, typically for the purposes of analysis or visualization.
- class holoviews.core.operation.Operation(*, dynamic, group, input_ranges, link_inputs, streams, name)[source]#
Bases:
ParameterizedFunction
An Operation process an Element or HoloMap at the level of individual elements or overlays. If a holomap is passed in as input, a processed holomap is returned as output where the individual elements have been transformed accordingly. An Operation may turn overlays in new elements or vice versa.
An Operation can be set to be dynamic, which will return a DynamicMap with a callback that will apply the operation dynamically. An Operation may also supply a list of Stream classes on a streams parameter, which can allow dynamic control over the parameters on the operation.
group
= param.String(allow_refs=False, default=’Operation’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b5f1450>)The group string used to identify the output of the Operation. By default this should match the operation name.
dynamic
= param.ObjectSelector(allow_refs=False, default=’default’, label=’Dynamic’, names={}, nested_refs=False, objects=[‘default’, True, False], rx=<param.reactive.reactive_ops object at 0x15b295590>)Whether the operation should be applied dynamically when a specific frame is requested, specified as a Boolean. If set to ‘default’ the mode will be determined based on the input type, i.e. if the data is a DynamicMap it will stay dynamic.
input_ranges
= param.ClassSelector(allow_None=True, allow_refs=False, class_=(<class ‘dict’>, <class ‘tuple’>), default={}, label=’Input ranges’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b2f2110>)Ranges to be used for input normalization (if applicable) in a format appropriate for the Normalization.ranges parameter. By default, no normalization is applied. If key-wise normalization is required, a 2-tuple may be supplied where the first component is a Normalization.ranges list and the second component is Normalization.keys.
link_inputs
= param.Boolean(allow_refs=False, default=False, label=’Link inputs’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b30e5d0>)If the operation is dynamic, whether or not linked streams should be transferred from the operation inputs for backends that support linked streams. For example if an operation is applied to a DynamicMap with an RangeXY, this switch determines whether the corresponding visualization should update this stream with range changes originating from the newly generated axes.
streams
= param.ClassSelector(allow_refs=False, class_=(<class ‘dict’>, <class ‘list’>), default=[], label=’Streams’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b2f2050>)List of streams that are applied if dynamic=True, allowing for dynamic interaction with the plot.
- classmethod get_overlay_bounds(overlay)[source]#
Returns the extents if all the elements of an overlay agree on a consistent extents, otherwise raises an exception.
- classmethod get_overlay_label(overlay, default_label='')[source]#
Returns a label if all the elements of an overlay agree on a consistent label, otherwise returns the default label.
- classmethod instance(**params)[source]#
Return an instance of this class, copying parameters from any existing instance provided.
- class holoviews.core.operation.OperationCallable(callable, **kwargs)[source]#
Bases:
Callable
OperationCallable allows wrapping an Operation and the objects it is processing to allow traversing the operations applied on a DynamicMap.
Parameters inherited from:
holoviews.core.spaces.Callable
: callable, inputs, operation_kwargs, link_inputs, memoize, stream_mappingoperation
= param.ClassSelector(allow_None=True, allow_refs=False, class_=<class ‘holoviews.core.operation.Operation’>, label=’Operation’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b14a950>)The Operation being wrapped into an OperationCallable.
- clone(callable=None, **overrides)[source]#
Clones the Callable optionally with new settings
- Args:
callable: New callable function to wrap **overrides: Parameter overrides to apply
- Returns:
Cloned Callable object
- property noargs#
Returns True if the callable takes no arguments
options
Module#
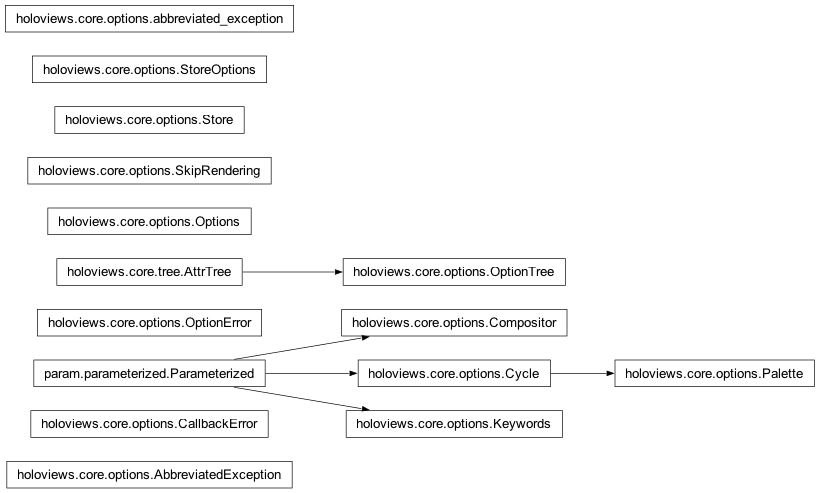
Options and OptionTrees allow different classes of options (e.g. matplotlib-specific styles and plot specific parameters) to be defined separately from the core data structures and away from visualization specific code.
There are three classes that form the options system:
Cycle:
Used to define infinite cycles over a finite set of elements, using either an explicit list or some pre-defined collection (e.g. from matplotlib rcParams). For instance, a Cycle object can be used loop a set of display colors for multiple curves on a single axis.
Options:
Containers of arbitrary keyword values, including optional keyword validation, support for Cycle objects and inheritance.
OptionTree:
A subclass of AttrTree that is used to define the inheritance relationships between a collection of Options objects. Each node of the tree supports a group of Options objects and the leaf nodes inherit their keyword values from parent nodes up to the root.
Store:
A singleton class that stores all global and custom options and links HoloViews objects, the chosen plotting backend and the IPython extension together.
- exception holoviews.core.options.AbbreviatedException(etype, value, traceback)[source]#
Bases:
Exception
Raised by the abbreviate_exception context manager when it is appropriate to present an abbreviated the traceback and exception message in the notebook.
Particularly useful when processing style options supplied by the user which may not be valid.
- add_note()#
Exception.add_note(note) – add a note to the exception
- print_traceback()[source]#
Print the traceback of the exception wrapped by the AbbreviatedException.
- with_traceback()#
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- exception holoviews.core.options.CallbackError[source]#
Bases:
RuntimeError
An error raised during a callback.
- add_note()#
Exception.add_note(note) – add a note to the exception
- with_traceback()#
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class holoviews.core.options.Compositor(pattern, operation, group, mode, transfer_options=False, transfer_parameters=False, output_type=None, backends=None, **kwargs)[source]#
Bases:
Parameterized
A Compositor is a way of specifying an operation to be automatically applied to Overlays that match a specified pattern upon display.
Any Operation that takes an Overlay as input may be used to define a compositor.
For instance, a compositor may be defined to automatically display three overlaid monochrome matrices as an RGB image as long as the values names of those matrices match ‘R’, ‘G’ and ‘B’.
mode
= param.ObjectSelector(allow_refs=False, default=’data’, label=’Mode’, names={}, nested_refs=False, objects=[‘data’, ‘display’], rx=<param.reactive.reactive_ops object at 0x15b03b890>)The mode of the Compositor object which may be either ‘data’ or ‘display’.
backends
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Backends’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b03ac90>)Defines which backends to apply the Compositor for.
operation
= param.Parameter(allow_None=True, allow_refs=False, label=’Operation’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ac30190>)The Operation to apply when collapsing overlays.
pattern
= param.String(allow_refs=False, default=’’, label=’Pattern’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b03b4d0>)The overlay pattern to be processed. An overlay pattern is a sequence of elements specified by dotted paths separated by * . For instance the following pattern specifies three overlaid matrices with values of ‘RedChannel’, ‘GreenChannel’ and ‘BlueChannel’ respectively: ‘Image.RedChannel * Image.GreenChannel * Image.BlueChannel. This pattern specification could then be associated with the RGB operation that returns a single RGB matrix for display.
group
= param.String(allow_None=True, allow_refs=False, default=’’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ac30190>)The group identifier for the output of this particular compositor
kwargs
= param.Dict(allow_None=True, allow_refs=False, class_=<class ‘dict’>, label=’Kwargs’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b03af50>)Optional set of parameters to pass to the operation.
transfer_options
= param.Boolean(allow_refs=False, default=False, label=’Transfer options’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ac30190>)Whether to transfer the options from the input to the output.
transfer_parameters
= param.Boolean(allow_refs=False, default=False, label=’Transfer parameters’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b03b710>)Whether to transfer plot options which match to the operation.
- apply(value, input_ranges, backend=None)[source]#
Apply the compositor on the input with the given input ranges.
- classmethod collapse(holomap, ranges=None, mode='data')[source]#
Given a map of Overlays, apply all applicable compositors.
- classmethod collapse_element(overlay, ranges=None, mode='data', backend=None)[source]#
Finds any applicable compositor and applies it.
- classmethod map(obj, mode='data', backend=None)[source]#
Applies compositor operations to any HoloViews element or container using the map method.
- match_level(overlay)[source]#
Given an overlay, return the match level and applicable slice of the overall overlay. The level an integer if there is a match or None if there is no match.
The level integer is the number of matching components. Higher values indicate a stronger match.
- property output_type#
Returns the operation output_type unless explicitly overridden in the kwargs.
- classmethod strongest_match(overlay, mode, backend=None)[source]#
Returns the single strongest matching compositor operation given an overlay. If no matches are found, None is returned.
The best match is defined as the compositor operation with the highest match value as returned by the match_level method.
- class holoviews.core.options.Cycle(cycle=None, **params)[source]#
Bases:
Parameterized
A simple container class that specifies cyclic options. A typical example would be to cycle the curve colors in an Overlay composed of an arbitrary number of curves. The values may be supplied as an explicit list or a key to look up in the default cycles attribute.
key
= param.String(allow_None=True, allow_refs=False, default=’default_colors’, label=’Key’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b0389d0>)The key in the default_cycles dictionary used to specify the color cycle if values is not supplied.
values
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Values’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aecfb90>)The values the cycle will iterate over.
- class holoviews.core.options.Keywords(values=None, target=None)[source]#
Bases:
Parameterized
A keywords objects represents a set of Python keywords. It is list-like and ordered but it is also a set without duplicates. When passed as **kwargs, Python keywords are not ordered but this class always lists keywords in sorted order.
In addition to containing the list of keywords, Keywords has an optional target which describes what the keywords are applicable to.
This class is for internal use only and should not be in the user namespace.
values
= param.List(allow_refs=False, bounds=(0, None), default=[], label=’Values’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aec1c50>)Set of keywords as a sorted list.
target
= param.String(allow_None=True, allow_refs=False, default=’’, label=’Target’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15aec3790>)Optional string description of what the keywords apply to.
- exception holoviews.core.options.OptionError(invalid_keyword, allowed_keywords, group_name=None, path=None)[source]#
Bases:
Exception
Custom exception raised when there is an attempt to apply invalid options. Stores the necessary information to construct a more readable message for the user if caught and processed appropriately.
- add_note()#
Exception.add_note(note) – add a note to the exception
- with_traceback()#
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class holoviews.core.options.OptionTree(items=None, identifier=None, parent=None, groups=None, options=None, backend=None, **kwargs)[source]#
Bases:
AttrTree
A subclass of AttrTree that is used to define the inheritance relationships between a collection of Options objects. Each node of the tree supports a group of Options objects and the leaf nodes inherit their keyword values from parent nodes up to the root.
Supports the ability to search the tree for the closest valid path using the find method, or compute the appropriate Options value given an object and a mode. For a given node of the tree, the options method computes a Options object containing the result of inheritance for a given group up to the root of the tree.
When constructing an OptionTree, you can specify the option groups as a list (i.e. empty initial option groups at the root) or as a dictionary (e.g. groups={‘style’:Option()}). You can also initialize the OptionTree with the options argument together with the **kwargs - see StoreOptions.merge_options for more information on the options specification syntax.
You can use the string specifier ‘.’ to refer to the root node in the options specification. This acts as an alternative was of specifying the options groups of the current node. Note that this approach method may only be used with the group lists format.
- closest(obj, group, defaults=True, backend=None)[source]#
This method is designed to be called from the root of the tree. Given any LabelledData object, this method will return the most appropriate Options object, including inheritance.
In addition, closest supports custom options by checking the object
- find(path, mode='node')[source]#
Find the closest node or path to an the arbitrary path that is supplied down the tree from the given node. The mode argument may be either ‘node’ or ‘path’ which determines the return type.
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- options(group, target=None, defaults=True, backend=None)[source]#
Using inheritance up to the root, get the complete Options object for the given node and the specified group.
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- class holoviews.core.options.Options(key=None, allowed_keywords=None, merge_keywords=True, max_cycles=None, **kwargs)[source]#
Bases:
object
An Options object holds a collection of keyword options. In addition, Options support (optional) keyword validation as well as infinite indexing over the set of supplied cyclic values.
Options support inheritance of setting values via the __call__ method. By calling an Options object with additional keywords, you can create a new Options object inheriting the parent options.
- property cyclic#
Returns True if the options cycle, otherwise False
- filtered(allowed)[source]#
Return a new Options object that is filtered by the specified list of keys. Mutating self.kwargs to filter is unsafe due to the option expansion that occurs on initialization.
- keywords_target(target)[source]#
Helper method to easily set the target on the allowed_keywords Keywords.
- max_cycles(num)[source]#
Truncates all contained Palette objects to a maximum number of samples and returns a new Options object containing the truncated or resampled Palettes.
- property options#
Access of the options keywords when no cycles are defined.
- class holoviews.core.options.Palette(key, **params)[source]#
Bases:
Cycle
Palettes allow easy specifying a discrete sampling of an existing colormap. Palettes may be supplied a key to look up a function function in the colormap class attribute. The function should accept a float scalar in the specified range and return a RGB(A) tuple. The number of samples may also be specified as a parameter.
The range and samples may conveniently be overridden with the __getitem__ method.
Parameters inherited from:
holoviews.core.options.Cycle
: valueskey
= param.String(allow_refs=False, default=’grayscale’, label=’Key’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b22b710>)Palettes look up the Palette values based on some key.
range
= param.NumericTuple(allow_refs=False, default=(0, 1), label=’Range’, length=2, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b11d350>)The range from which the Palette values are sampled.
samples
= param.Integer(allow_refs=False, default=32, inclusive_bounds=(True, True), label=’Samples’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b600150>)The number of samples in the given range to supply to the sample_fn.
sample_fn
= param.Callable(allow_refs=False, default=<function linspace at 0x105f07a30>, label=’Sample fn’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b11fa10>)The function to generate the samples, by default linear.
reverse
= param.Boolean(allow_refs=False, default=False, label=’Reverse’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15b22bad0>)Whether to reverse the palette.
- sample_fn(stop, num=50, endpoint=True, retstep=False, dtype=None, axis=0)[source]#
Return evenly spaced numbers over a specified interval.
Returns num evenly spaced samples, calculated over the interval [start, stop].
The endpoint of the interval can optionally be excluded.
Changed in version 1.16.0: Non-scalar start and stop are now supported.
Changed in version 1.20.0: Values are rounded towards
-inf
instead of0
when an integerdtype
is specified. The old behavior can still be obtained withnp.linspace(start, stop, num).astype(int)
Parameters#
- startarray_like
The starting value of the sequence.
- stoparray_like
The end value of the sequence, unless endpoint is set to False. In that case, the sequence consists of all but the last of
num + 1
evenly spaced samples, so that stop is excluded. Note that the step size changes when endpoint is False.- numint, optional
Number of samples to generate. Default is 50. Must be non-negative.
- endpointbool, optional
If True, stop is the last sample. Otherwise, it is not included. Default is True.
- retstepbool, optional
If True, return (samples, step), where step is the spacing between samples.
- dtypedtype, optional
The type of the output array. If dtype is not given, the data type is inferred from start and stop. The inferred dtype will never be an integer; float is chosen even if the arguments would produce an array of integers.
Added in version 1.9.0.
- axisint, optional
The axis in the result to store the samples. Relevant only if start or stop are array-like. By default (0), the samples will be along a new axis inserted at the beginning. Use -1 to get an axis at the end.
Added in version 1.16.0.
Returns#
- samplesndarray
There are num equally spaced samples in the closed interval
[start, stop]
or the half-open interval[start, stop)
(depending on whether endpoint is True or False).- stepfloat, optional
Only returned if retstep is True
Size of spacing between samples.
See Also#
- arangeSimilar to linspace, but uses a step size (instead of the
number of samples).
- geomspaceSimilar to linspace, but with numbers spaced evenly on a log
scale (a geometric progression).
- logspaceSimilar to geomspace, but with the end points specified as
logarithms.
how-to-partition
Examples#
>>> np.linspace(2.0, 3.0, num=5) array([2. , 2.25, 2.5 , 2.75, 3. ]) >>> np.linspace(2.0, 3.0, num=5, endpoint=False) array([2. , 2.2, 2.4, 2.6, 2.8]) >>> np.linspace(2.0, 3.0, num=5, retstep=True) (array([2. , 2.25, 2.5 , 2.75, 3. ]), 0.25)
Graphical illustration:
>>> import matplotlib.pyplot as plt >>> N = 8 >>> y = np.zeros(N) >>> x1 = np.linspace(0, 10, N, endpoint=True) >>> x2 = np.linspace(0, 10, N, endpoint=False) >>> plt.plot(x1, y, 'o') [<matplotlib.lines.Line2D object at 0x...>] >>> plt.plot(x2, y + 0.5, 'o') [<matplotlib.lines.Line2D object at 0x...>] >>> plt.ylim([-0.5, 1]) (-0.5, 1) >>> plt.show()
- exception holoviews.core.options.SkipRendering(message='', warn=True)[source]#
Bases:
Exception
A SkipRendering exception in the plotting code will make the display hooks fall back to a text repr. Used to skip rendering of DynamicMaps with exhausted element generators.
- add_note()#
Exception.add_note(note) – add a note to the exception
- with_traceback()#
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- class holoviews.core.options.Store[source]#
Bases:
object
The Store is what links up HoloViews objects to their corresponding options and to the appropriate classes of the chosen backend (e.g. for rendering).
In addition, Store supports pickle operations that automatically pickle and unpickle the corresponding options for a HoloViews object.
- classmethod add_style_opts(component, new_options, backend=None)[source]#
Given a component such as an Element (e.g. Image, Curve) or a container (e.g. Layout) specify new style options to be accepted by the corresponding plotting class.
Note: This is supplied for advanced users who know which additional style keywords are appropriate for the corresponding plotting class.
- classmethod dump(obj, file, protocol=0)[source]#
Equivalent to pickle.dump except that the HoloViews option tree is saved appropriately.
- classmethod dumps(obj, protocol=0)[source]#
Equivalent to pickle.dumps except that the HoloViews option tree is saved appropriately.
- classmethod info(obj, ansi=True, backend='matplotlib', visualization=True, recursive=False, pattern=None, elements=None)[source]#
Show information about a particular object or component class including the applicable style and plot options. Returns None if the object is not parameterized.
- classmethod load(filename)[source]#
Equivalent to pickle.load except that the HoloViews trees is restored appropriately.
- classmethod loaded_backends()[source]#
Returns a list of the backends that have been loaded, based on the available OptionTrees.
- classmethod loads(pickle_string)[source]#
Equivalent to pickle.loads except that the HoloViews trees is restored appropriately.
- classmethod lookup(backend, obj)[source]#
Given an object, lookup the corresponding customized option tree if a single custom tree is applicable.
- output_settings[source]#
alias of
OutputSettings
- classmethod register(associations, backend, style_aliases=None)[source]#
Register the supplied dictionary of associations between elements and plotting classes to the specified backend.
- classmethod render(obj)[source]#
Using any display hooks that have been registered, render the object to a dictionary of MIME types and metadata information.
- classmethod set_current_backend(backend)[source]#
Use this method to set the backend to run the switch hooks
- class holoviews.core.options.StoreOptions[source]#
Bases:
object
A collection of utilities for advanced users for creating and setting customized option trees on the Store. Designed for use by either advanced users or the %opts line and cell magics which use this machinery.
This class also holds general classmethods for working with OptionTree instances: as OptionTrees are designed for attribute access it is best to minimize the number of methods implemented on that class and implement the necessary utilities on StoreOptions instead.
Lastly this class offers a means to record all OptionErrors generated by an option specification. This is used for validation purposes.
- classmethod apply_customizations(spec, options)[source]#
Apply the given option specs to the supplied options tree.
- classmethod capture_ids(obj)[source]#
Given an list of ids, capture a list of ids that can be restored using the restore_ids.
- classmethod create_custom_trees(obj, options=None, backend=None)[source]#
Returns the appropriate set of customized subtree clones for an object, suitable for merging with Store.custom_options (i.e with the ids appropriately offset). Note if an object has no integer ids a new OptionTree is built.
The id_mapping return value is a list mapping the ids that need to be matched as set to their new values.
- classmethod expand_compositor_keys(spec)[source]#
Expands compositor definition keys into {type}.{group} keys. For instance a compositor operation returning a group string ‘Image’ of element type RGB expands to ‘RGB.Image’.
- classmethod id_offset()[source]#
Compute an appropriate offset for future id values given the set of ids currently defined across backends.
- classmethod merge_options(groups, options=None, **kwargs)[source]#
Given a full options dictionary and options groups specified as a keywords, return the full set of merged options:
>>> options={'Curve':{'style':dict(color='b')}} >>> style={'Curve':{'linewidth':10 }} >>> merged = StoreOptions.merge_options(['style'], options, style=style) >>> sorted(merged['Curve']['style'].items()) [('color', 'b'), ('linewidth', 10)]
- classmethod options(obj, options=None, **kwargs)[source]#
Context-manager for temporarily setting options on an object (if options is None, no options will be set) . Once the context manager exits, both the object and the Store will be left in exactly the same state they were in before the context manager was used.
See holoviews.core.options.set_options function for more information on the options specification format.
- classmethod propagate_ids(obj, match_id, new_id, applied_keys, backend=None)[source]#
Recursively propagate an id through an object for components matching the applied_keys. This method can only be called if there is a tree with a matching id in Store.custom_options
- classmethod record_skipped_option(error)[source]#
Record the OptionError associated with a skipped option if currently recording
- classmethod restore_ids(obj, ids)[source]#
Given an list of ids as captured with capture_ids, restore the ids. Note the structure of an object must not change between the calls to capture_ids and restore_ids.
- classmethod set_options(obj, options=None, backend=None, **kwargs)[source]#
Pure Python function for customize HoloViews objects in terms of their style, plot and normalization options.
The options specification is a dictionary containing the target for customization as a {type}.{group}.{label} keys. An example of such a key is ‘Image’ which would customize all Image components in the object. The key ‘Image.Channel’ would only customize Images in the object that have the group ‘Channel’.
The corresponding value is then a list of Option objects specified with an appropriate category (‘plot’, ‘style’ or ‘norm’). For instance, using the keys described above, the specs could be:
{‘Image:[Options(‘style’, cmap=’jet’)]}
Or setting two types of option at once:
- {‘Image.Channel’:[Options(‘plot’, size=50),
Options(‘style’, cmap=’Blues’)]}
Relationship to the %%opts magic#
This function matches the functionality supplied by the %%opts cell magic in the IPython extension. In fact, you can use the same syntax as the IPython cell magic to achieve the same customization as shown above:
from holoviews.util.parser import OptsSpec set_options(my_image, OptsSpec.parse(“Image (cmap=’jet’)”))
Then setting both plot and style options:
set_options(my_image, OptsSpec.parse(“Image [size=50] (cmap=’Blues’)”))
- classmethod start_recording_skipped()[source]#
Start collecting OptionErrors for all skipped options recorded with the record_skipped_option method
- classmethod state(obj, state=None)[source]#
Method to capture and restore option state. When called without any state supplied, the current state is returned. Then if this state is supplied back in a later call using the same object, the original state is restored.
- classmethod stop_recording_skipped()[source]#
Stop collecting OptionErrors recorded with the record_skipped_option method and return them
- classmethod tree_to_dict(tree)[source]#
Given an OptionTree, convert it into the equivalent dictionary format.
- classmethod update_backends(id_mapping, custom_trees, backend=None)[source]#
Given the id_mapping from previous ids to new ids and the new custom tree dictionary, update the current backend with the supplied trees and update the keys in the remaining backends to stay linked with the current object.
- classmethod validate_spec(spec, backends=None)[source]#
Given a specification, validated it against the options tree for the specified backends by raising OptionError for invalid options. If backends is None, validates against all the currently loaded backend.
Only useful when invalid keywords generate exceptions instead of skipping, i.e. Options.skip_invalid is False.
- class holoviews.core.options.abbreviated_exception[source]#
Bases:
object
Context manager used to to abbreviate tracebacks using an AbbreviatedException when a backend may raise an error due to incorrect style options.
- holoviews.core.options.cleanup_custom_options(id, weakref=None)[source]#
Cleans up unused custom trees if all objects referencing the custom id have been garbage collected or tree is otherwise unreferenced.
overlay
Module#

Supplies Layer and related classes that allow overlaying of Views, including Overlay. A Layer is the final extension of View base class that allows Views to be overlaid on top of each other.
Also supplies ViewMap which is the primary multi-dimensional Map type for indexing, slicing and animating collections of Views.
- class holoviews.core.overlay.AdjointLayout(data, **params)[source]#
Bases:
Layoutable
,Dimensioned
An AdjointLayout provides a convenient container to lay out some marginal plots next to a primary plot. This is often useful to display the marginal distributions of a plot next to the primary plot. AdjointLayout accepts a list of up to three elements, which are laid out as follows with the names ‘main’, ‘top’ and ‘right’:
3 | ||___________|___| | | | 1: main | | | 2: right | 1 | 2 | 3: top | | | |___________|___|
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: group, cdims, vdimskdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[Dimension(‘AdjointLayout’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159c77990>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Applies to the main object in the AdjointLayout.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get(key, default=None)[source]#
Returns the viewable corresponding to the supplied string or integer based key.
- Args:
key: Numeric or string index: 0) ‘main’ 1) ‘right’ 2) ‘top’ default: Value returned if key not found
- Returns:
Indexed value or supplied default
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from main element
- property label#
Label inherited from main element
- property main#
Returns the main element in the AdjointLayout
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to child up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- property right#
Returns the right marginal element in the AdjointLayout
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property top#
Returns the top marginal element in the AdjointLayout
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.overlay.CompositeOverlay(data, kdims=None, vdims=None, **params)[source]#
Bases:
ViewableElement
,Composable
CompositeOverlay provides a common baseclass for Overlay classes.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdims- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, index=None, show_legend=False, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
- dimension: Dimension(s) to compute histogram on,
Falls back the plot dimensions by default.
num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram index (int, optional): Index of layer to apply hist to show_legend (bool, optional): Show legend in histogram
(don’t show legend by default).
- Returns:
AdjointLayout of element and histogram or just the histogram
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.overlay.Dimensioned(data, kdims=None, vdims=None, **params)[source]#
Bases:
LabelledData
Dimensioned is a base class that allows the data contents of a class to be associated with dimensions. The contents associated with dimensions may be partitioned into one of three types
- key dimensions: These are the dimensions that can be indexed via
the __getitem__ method. Dimension objects supporting key dimensions must support indexing over these dimensions and may also support slicing. This list ordering of dimensions describes the positional components of each multi-dimensional indexing operation.
For instance, if the key dimension names are ‘weight’ followed by ‘height’ for Dimensioned object ‘obj’, then obj[80,175] indexes a weight of 80 and height of 175.
Accessed using either kdims.
- value dimensions: These dimensions correspond to any data held
on the Dimensioned object not in the key dimensions. Indexing by value dimension is supported by dimension name (when there are multiple possible value dimensions); no slicing semantics is supported and all the data associated with that dimension will be returned at once. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either vdims.
- deep dimensions: These are dynamically computed dimensions that
belong to other Dimensioned objects that are nested in the data. Objects that support this should enable the _deep_indexable flag. Note that it is not possible to mix value dimensions and deep dimensions.
Accessed using either ddims.
Dimensioned class support generalized methods for finding the range and type of values along a particular Dimension. The range method relies on the appropriate implementation of the dimension_values methods on subclasses.
The index of an arbitrary dimension is its positional index in the list of all dimensions, starting with the key dimensions, followed by the value dimensions and ending with the deep dimensions.
Parameters inherited from:
group
= param.String(allow_refs=False, constant=True, default=’Dimensioned’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a031150>)A string describing the data wrapped by the object.
cdims
= param.Dict(allow_refs=False, class_=<class ‘dict’>, default={}, label=’Cdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a212a10>)The constant dimensions defined as a dictionary of Dimension:value pairs providing additional dimension information about the object. Aliased with constant_dimensions.
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a031150>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
vdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Vdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15a289090>)The value dimensions defined as the list of dimensions used to describe the components of the data. If multiple value dimensions are supplied, a particular value dimension may be indexed by name after the key dimensions. Aliased with value_dimensions.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.overlay.Layout(items=None, identifier=None, parent=None, **kwargs)[source]#
Bases:
Layoutable
,ViewableTree
A Layout is an ViewableTree with ViewableElement objects as leaf values. Unlike ViewableTree, a Layout supports a rich display, displaying leaf items in a grid style layout. In addition to the usual ViewableTree indexing, Layout supports indexing of items by their row and column index in the layout.
The maximum number of columns in such a layout may be controlled with the cols method.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’Layout’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15991e9d0>)A string describing the data wrapped by the object.
- cols(ncols)[source]#
Sets the maximum number of columns in the NdLayout.
Any items beyond the set number of cols will flow onto a new row. The number of columns control the indexing and display semantics of the NdLayout.
- Args:
ncols (int): Number of columns to set on the NdLayout
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs Layout of DynamicMaps into a single DynamicMap that returns a Layout
Decollation allows packing a Layout of DynamicMaps into a single DynamicMap that returns a Layout of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a Layout
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- property shape#
Tuple indicating the number of rows and columns in the Layout.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
- class holoviews.core.overlay.NdOverlay(overlays=None, kdims=None, **params)[source]#
Bases:
Overlayable
,UniformNdMapping
,CompositeOverlay
An NdOverlay allows a group of NdOverlay to be overlaid together. NdOverlay can be indexed out of an overlay and an overlay is an iterable that iterates over the contained layers.
Parameters inherited from:
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[Dimension(‘Element’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159777b10>)List of dimensions the NdOverlay can be indexed by.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs NdOverlay of DynamicMaps into a single DynamicMap that returns an NdOverlay
Decollation allows packing a NdOverlay of DynamicMaps into a single DynamicMap that returns an NdOverlay of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns an NdOverlay
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, index=None, show_legend=False, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
- dimension: Dimension(s) to compute histogram on,
Falls back the plot dimensions by default.
num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram index (int, optional): Index of layer to apply hist to show_legend (bool, optional): Show legend in histogram
(don’t show legend by default).
- Returns:
AdjointLayout of element and histogram or just the histogram
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.overlay.Overlay(items=None, group=None, label=None, **params)[source]#
Bases:
ViewableTree
,CompositeOverlay
,Layoutable
,Overlayable
An Overlay consists of multiple Elements (potentially of heterogeneous type) presented one on top each other with a particular z-ordering.
Overlays along with elements constitute the only valid leaf types of a Layout and in fact extend the Layout structure. Overlays are constructed using the * operator (building an identical structure to the + operator).
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdims- clone(data=None, shared_data=True, new_type=None, link=True, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collate()[source]#
Collates any objects in the Overlay resolving any issues the recommended nesting structure.
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs Overlay of DynamicMaps into a single DynamicMap that returns an Overlay
Decollation allows packing an Overlay of DynamicMaps into a single DynamicMap that returns an Overlay of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns an Overlay
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a layer in the Overlay.
Get a particular layer in the Overlay using its path string or an integer index.
- Args:
identifier: Index or path string of the item to return default: Value to return if no item is found
- Returns:
The indexed layer of the Overlay
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
str(object=’’) -> str str(bytes_or_buffer[, encoding[, errors]]) -> str
Create a new string object from the given object. If encoding or errors is specified, then the object must expose a data buffer that will be decoded using the given encoding and error handler. Otherwise, returns the result of object.__str__() (if defined) or repr(object). encoding defaults to sys.getdefaultencoding(). errors defaults to ‘strict’.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, index=None, show_legend=False, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
- dimension: Dimension(s) to compute histogram on,
Falls back the plot dimensions by default.
num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram index (int, optional): Index of layer to apply hist to show_legend (bool, optional): Show legend in histogram
(don’t show legend by default).
- Returns:
AdjointLayout of element and histogram or just the histogram
- property label#
str(object=’’) -> str str(bytes_or_buffer[, encoding[, errors]]) -> str
Create a new string object from the given object. If encoding or errors is specified, then the object must expose a data buffer that will be decoded using the given encoding and error handler. Otherwise, returns the result of object.__str__() (if defined) or repr(object). encoding defaults to sys.getdefaultencoding(). errors defaults to ‘strict’.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
- class holoviews.core.overlay.Overlayable[source]#
Bases:
object
Overlayable provides a mix-in class to support the mul operation for overlaying multiple elements.
- class holoviews.core.overlay.UniformNdMapping(initial_items=None, kdims=None, group=None, label=None, **params)[source]#
Bases:
NdMapping
A UniformNdMapping is a map of Dimensioned objects and is itself indexed over a number of specified dimensions. The dimension may be a spatial dimension (i.e., a ZStack), time (specifying a frame sequence) or any other combination of Dimensions.
UniformNdMapping objects can be sliced, sampled, reduced, overlaid and split along its and its containing Element’s dimensions. Subclasses should implement the appropriate slicing, sampling and reduction methods for their Dimensioned type.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.overlay.ViewableElement(data, kdims=None, vdims=None, **params)[source]#
Bases:
Dimensioned
A ViewableElement is a dimensioned datastructure that may be associated with a corresponding atomic visualization. An atomic visualization will display the data on a single set of axes (i.e. excludes multiple subplots that are displayed at once). The only new parameter introduced by ViewableElement is the title associated with the object for display.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’ViewableElement’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x159886810>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- class holoviews.core.overlay.ViewableTree(items=None, identifier=None, parent=None, **kwargs)[source]#
Bases:
AttrTree
,Dimensioned
A ViewableTree is an AttrTree with Viewable objects as its leaf nodes. It combines the tree like data structure of a tree while extending it with the deep indexable properties of Dimensioned and LabelledData objects.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdims, kdims, vdimsgroup
= param.String(allow_refs=False, constant=True, default=’ViewableTree’, label=’Group’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x1595a9a50>)A string describing the data wrapped by the object.
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- property ddims#
The list of deep dimensions
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
Concatenates values on all nodes with requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property uniform#
Whether items in tree have uniform dimensions
pprint
Module#

HoloViews can be used to build highly-nested data-structures containing large amounts of raw data. As a result, it is difficult to generate a readable representation that is both informative yet concise.
As a result, HoloViews does not attempt to build representations that can be evaluated with eval; such representations would typically be far too large to be practical. Instead, all HoloViews objects can be represented as tree structures, showing how to access and index into your data.
- class holoviews.core.pprint.InfoPrinter[source]#
Bases:
object
Class for printing other information related to an object that is of use to the user.
- classmethod get_parameter_info(obj, ansi=False, show_values=True, pattern=None, max_col_len=40)[source]#
Get parameter information from the supplied class or object.
- classmethod heading(heading_text, char='=', level=0, ansi=False)[source]#
Turn the supplied heading text into a suitable heading with optional underline and color.
- class holoviews.core.pprint.PrettyPrinter(*, show_defaults, show_options, name)[source]#
Bases:
Parameterized
The PrettyPrinter used to print all HoloView objects via the pprint method.
show_defaults
= param.Boolean(allow_refs=False, default=False, label=’Show defaults’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15bfa4c90>)Whether to show default options as part of the repr. If show_options=False this has no effect.
show_options
= param.Boolean(allow_refs=False, default=False, label=’Show options’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15bf95590>)Whether to show options as part of the repr.
- element_info(node, siblings, level, value_dims)[source]#
Return the information summary for an Element. This consists of the dotted name followed by an value dimension names.
sheetcoords
Module#

File originally part of the Topographica project. Provides SheetCoordinateSystem, allowing conversion between continuous ‘sheet coordinates’ and integer matrix coordinates.
‘Sheet coordinates’ allow simulation parameters to be specified in units that are density-independent, whereas ‘matrix coordinates’ provide a means of realizing the continuous sheets.
Hence we can have a pair of ‘sheet coordinates’ (x,y); floating-point Cartesian coordinates indicating an arbitrary point on the sheet’s plane. We can also have a pair of ‘matrix coordinates’ (r,c), which are used to address an underlying matrix. These matrix coordinates are also floating-point numbers to allow precise conversion between the two schemes. Where it is necessary to address a specific element of the matrix (as is often the case in calculations), we also have the usual matrix index coordinates (r_idx, c_idx). We refer to these as matrixidx coordinates. SheetCoordinateSystem proxies methods for converting between sheet and matrix coordinates, as well as sheet and matrixidx coordinates.
Everyone should use these facilities for conversions between the two coordinate systems to guarantee consistency.
Example of how the matrix stores the representation of the Sheet#
For the purposes of this example, assume the goal is a Sheet with density=3 that has a 1 at (-1/2,-1/2), a 5 at (0.0,0.0), and a 9 at (1/2,1/2). More precisely, for this Sheet,
the continuous area from -1/2,-1/2 to -1/6,-1/6 has value 1, the continuous area from -1/6,-1/6 to 1/6,1/6 has value 5, and the continuous area from 1/6,1/6 to 1/2,1/2 has value 9.
With the rest of the elements filled in, the Sheet would look like:
(-1/2,1/2) -+-----+-----+-----+- (1/2,1/2)
| | | |
| 7 | 8 | 9 |
| | | |
(-1/2,1/6) -+-----+-----+-----+- (1/2,1/6)
| | | |
| 4 | 5 | 6 |
| | | |
(-1/2,-1/6) -+-----+-----+-----+- (1/2,-1/6)
| | | |
| 1 | 2 | 3 |
| | | |
(-1/2,-1/2) -+-----+-----+-----+- (1/2,-1/2)
where element 5 is centered on 0.0,0.0. A matrix that would match these Sheet coordinates is:
[[7 8 9]
[4 5 6]
[1 2 3]]
If we have such a matrix, we can access it in one of two ways: Sheet or matrix/matrixidx coordinates. In matrixidx coordinates, the matrix is indexed by rows and columns, and it is possible to ask for the element at location [0,2] (which returns 9 as in any normal row-major matrix). But the values can additionally be accessed in Sheet coordinates, where the matrix is indexed by a point in the Cartesian plane. In Sheet coordinates, it is possible to ask for the element at location (0.3,0.02), which returns floating-point matrix coordinates that can be cropped to give the nearest matrix element, namely the one with value 6.
Of course, it would be an error to try to pass matrix coordinates like [0,2] to the sheet2matrix calls; the result would be a value far outside of the actual matrix.
- class holoviews.core.sheetcoords.SheetCoordinateSystem(bounds, xdensity, ydensity=None)[source]#
Bases:
object
Provides methods to allow conversion between sheet and matrix coordinates.
- closest_cell_center(x, y)[source]#
Given arbitrary sheet coordinates, return the sheet coordinates of the center of the closest unit.
- matrix2sheet(float_row, float_col)[source]#
Convert a floating-point location (float_row,float_col) in matrix coordinates to its corresponding location (x,y) in sheet coordinates.
Valid for scalar or array float_row and float_col.
Inverse of sheet2matrix().
- matrixidx2sheet(row, col)[source]#
Return (x,y) where x and y are the floating point coordinates of the center of the given matrix cell (row,col). If the matrix cell represents a 0.2 by 0.2 region, then the center location returned would be 0.1,0.1.
NOTE: This is NOT the strict mathematical inverse of sheet2matrixidx(), because sheet2matrixidx() discards all but the integer portion of the continuous matrix coordinate.
Valid only for scalar or array row and col.
- sheet2matrix(x, y)[source]#
Convert a point (x,y) in Sheet coordinates to continuous matrix coordinates.
Returns (float_row,float_col), where float_row corresponds to y, and float_col to x.
Valid for scalar or array x and y.
Note about Bounds For a Sheet with BoundingBox(points=((-0.5,-0.5),(0.5,0.5))) and density=3, x=-0.5 corresponds to float_col=0.0 and x=0.5 corresponds to float_col=3.0. float_col=3.0 is not inside the matrix representing this Sheet, which has the three columns (0,1,2). That is, x=-0.5 is inside the BoundingBox but x=0.5 is outside. Similarly, y=0.5 is inside (at row 0) but y=-0.5 is outside (at row 3) (it’s the other way round for y because the matrix row index increases as y decreases).
- sheet2matrixidx(x, y)[source]#
Convert a point (x,y) in sheet coordinates to the integer row and column index of the matrix cell in which that point falls, given a bounds and density. Returns (row,column).
Note that if coordinates along the right or bottom boundary are passed into this function, the returned matrix coordinate of the boundary will be just outside the matrix, because the right and bottom boundaries are exclusive.
Valid for scalar or array x and y.
- sheetcoordinates_of_matrixidx()[source]#
Return x,y where x is a vector of sheet coordinates representing the x-center of each matrix cell, and y represents the corresponding y-center of the cell.
- property xdensity#
The spacing between elements in an underlying matrix representation, in the x direction.
- property ydensity#
The spacing between elements in an underlying matrix representation, in the y direction.
- class holoviews.core.sheetcoords.Slice(bounds, sheet_coordinate_system, force_odd=False, min_matrix_radius=1)[source]#
Bases:
ndarray
Represents a slice of a SheetCoordinateSystem; i.e., an array specifying the row and column start and end points for a submatrix of the SheetCoordinateSystem.
The slice is created from the supplied bounds by calculating the slice that corresponds most closely to the specified bounds. Therefore, the slice does not necessarily correspond exactly to the specified bounds. The bounds that do exactly correspond to the slice are available via the ‘bounds’ attribute.
Note that the slice does not respect the bounds of the SheetCoordinateSystem, and that actions such as translate() also do not respect the bounds. To ensure that the slice is within the SheetCoordinateSystem’s bounds, use crop_to_sheet().
- T#
View of the transposed array.
Same as
self.transpose()
.Examples#
>>> a = np.array([[1, 2], [3, 4]]) >>> a array([[1, 2], [3, 4]]) >>> a.T array([[1, 3], [2, 4]])
>>> a = np.array([1, 2, 3, 4]) >>> a array([1, 2, 3, 4]) >>> a.T array([1, 2, 3, 4])
See Also#
transpose
- all(axis=None, out=None, keepdims=False, *, where=True)#
Returns True if all elements evaluate to True.
Refer to numpy.all for full documentation.
See Also#
numpy.all : equivalent function
- any(axis=None, out=None, keepdims=False, *, where=True)#
Returns True if any of the elements of a evaluate to True.
Refer to numpy.any for full documentation.
See Also#
numpy.any : equivalent function
- argmax(axis=None, out=None, *, keepdims=False)#
Return indices of the maximum values along the given axis.
Refer to numpy.argmax for full documentation.
See Also#
numpy.argmax : equivalent function
- argmin(axis=None, out=None, *, keepdims=False)#
Return indices of the minimum values along the given axis.
Refer to numpy.argmin for detailed documentation.
See Also#
numpy.argmin : equivalent function
- argpartition(kth, axis=-1, kind='introselect', order=None)#
Returns the indices that would partition this array.
Refer to numpy.argpartition for full documentation.
Added in version 1.8.0.
See Also#
numpy.argpartition : equivalent function
- argsort(axis=-1, kind=None, order=None)#
Returns the indices that would sort this array.
Refer to numpy.argsort for full documentation.
See Also#
numpy.argsort : equivalent function
- astype(dtype, order='K', casting='unsafe', subok=True, copy=True)#
Copy of the array, cast to a specified type.
Parameters#
- dtypestr or dtype
Typecode or data-type to which the array is cast.
- order{‘C’, ‘F’, ‘A’, ‘K’}, optional
Controls the memory layout order of the result. ‘C’ means C order, ‘F’ means Fortran order, ‘A’ means ‘F’ order if all the arrays are Fortran contiguous, ‘C’ order otherwise, and ‘K’ means as close to the order the array elements appear in memory as possible. Default is ‘K’.
- casting{‘no’, ‘equiv’, ‘safe’, ‘same_kind’, ‘unsafe’}, optional
Controls what kind of data casting may occur. Defaults to ‘unsafe’ for backwards compatibility.
‘no’ means the data types should not be cast at all.
‘equiv’ means only byte-order changes are allowed.
‘safe’ means only casts which can preserve values are allowed.
‘same_kind’ means only safe casts or casts within a kind, like float64 to float32, are allowed.
‘unsafe’ means any data conversions may be done.
- subokbool, optional
If True, then sub-classes will be passed-through (default), otherwise the returned array will be forced to be a base-class array.
- copybool, optional
By default, astype always returns a newly allocated array. If this is set to false, and the dtype, order, and subok requirements are satisfied, the input array is returned instead of a copy.
Returns#
- arr_tndarray
Unless copy is False and the other conditions for returning the input array are satisfied (see description for copy input parameter), arr_t is a new array of the same shape as the input array, with dtype, order given by dtype, order.
Notes#
Changed in version 1.17.0: Casting between a simple data type and a structured one is possible only for “unsafe” casting. Casting to multiple fields is allowed, but casting from multiple fields is not.
Changed in version 1.9.0: Casting from numeric to string types in ‘safe’ casting mode requires that the string dtype length is long enough to store the max integer/float value converted.
Raises#
- ComplexWarning
When casting from complex to float or int. To avoid this, one should use
a.real.astype(t)
.
Examples#
>>> x = np.array([1, 2, 2.5]) >>> x array([1. , 2. , 2.5])
>>> x.astype(int) array([1, 2, 2])
- base#
Base object if memory is from some other object.
Examples#
The base of an array that owns its memory is None:
>>> x = np.array([1,2,3,4]) >>> x.base is None True
Slicing creates a view, whose memory is shared with x:
>>> y = x[2:] >>> y.base is x True
- byteswap(inplace=False)#
Swap the bytes of the array elements
Toggle between low-endian and big-endian data representation by returning a byteswapped array, optionally swapped in-place. Arrays of byte-strings are not swapped. The real and imaginary parts of a complex number are swapped individually.
Parameters#
- inplacebool, optional
If
True
, swap bytes in-place, default isFalse
.
Returns#
- outndarray
The byteswapped array. If inplace is
True
, this is a view to self.
Examples#
>>> A = np.array([1, 256, 8755], dtype=np.int16) >>> list(map(hex, A)) ['0x1', '0x100', '0x2233'] >>> A.byteswap(inplace=True) array([ 256, 1, 13090], dtype=int16) >>> list(map(hex, A)) ['0x100', '0x1', '0x3322']
Arrays of byte-strings are not swapped
>>> A = np.array([b'ceg', b'fac']) >>> A.byteswap() array([b'ceg', b'fac'], dtype='|S3')
A.newbyteorder().byteswap()
produces an array with the same valuesbut different representation in memory
>>> A = np.array([1, 2, 3]) >>> A.view(np.uint8) array([1, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 3, 0, 0, 0, 0, 0, 0, 0], dtype=uint8) >>> A.newbyteorder().byteswap(inplace=True) array([1, 2, 3]) >>> A.view(np.uint8) array([0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 3], dtype=uint8)
- choose(choices, out=None, mode='raise')#
Use an index array to construct a new array from a set of choices.
Refer to numpy.choose for full documentation.
See Also#
numpy.choose : equivalent function
- clip(min=None, max=None, out=None, **kwargs)#
Return an array whose values are limited to
[min, max]
. One of max or min must be given.Refer to numpy.clip for full documentation.
See Also#
numpy.clip : equivalent function
- compress(condition, axis=None, out=None)#
Return selected slices of this array along given axis.
Refer to numpy.compress for full documentation.
See Also#
numpy.compress : equivalent function
- conj()#
Complex-conjugate all elements.
Refer to numpy.conjugate for full documentation.
See Also#
numpy.conjugate : equivalent function
- conjugate()#
Return the complex conjugate, element-wise.
Refer to numpy.conjugate for full documentation.
See Also#
numpy.conjugate : equivalent function
- copy(order='C')#
Return a copy of the array.
Parameters#
- order{‘C’, ‘F’, ‘A’, ‘K’}, optional
Controls the memory layout of the copy. ‘C’ means C-order, ‘F’ means F-order, ‘A’ means ‘F’ if a is Fortran contiguous, ‘C’ otherwise. ‘K’ means match the layout of a as closely as possible. (Note that this function and
numpy.copy()
are very similar but have different default values for their order= arguments, and this function always passes sub-classes through.)
See also#
numpy.copy : Similar function with different default behavior numpy.copyto
Notes#
This function is the preferred method for creating an array copy. The function
numpy.copy()
is similar, but it defaults to using order ‘K’, and will not pass sub-classes through by default.Examples#
>>> x = np.array([[1,2,3],[4,5,6]], order='F')
>>> y = x.copy()
>>> x.fill(0)
>>> x array([[0, 0, 0], [0, 0, 0]])
>>> y array([[1, 2, 3], [4, 5, 6]])
>>> y.flags['C_CONTIGUOUS'] True
- ctypes#
An object to simplify the interaction of the array with the ctypes module.
This attribute creates an object that makes it easier to use arrays when calling shared libraries with the ctypes module. The returned object has, among others, data, shape, and strides attributes (see Notes below) which themselves return ctypes objects that can be used as arguments to a shared library.
Parameters#
None
Returns#
- cPython object
Possessing attributes data, shape, strides, etc.
See Also#
numpy.ctypeslib
Notes#
Below are the public attributes of this object which were documented in “Guide to NumPy” (we have omitted undocumented public attributes, as well as documented private attributes):
- _ctypes.data
A pointer to the memory area of the array as a Python integer. This memory area may contain data that is not aligned, or not in correct byte-order. The memory area may not even be writeable. The array flags and data-type of this array should be respected when passing this attribute to arbitrary C-code to avoid trouble that can include Python crashing. User Beware! The value of this attribute is exactly the same as
self._array_interface_['data'][0]
.Note that unlike
data_as
, a reference will not be kept to the array: code likectypes.c_void_p((a + b).ctypes.data)
will result in a pointer to a deallocated array, and should be spelt(a + b).ctypes.data_as(ctypes.c_void_p)
- _ctypes.shape
(c_intp*self.ndim): A ctypes array of length self.ndim where the basetype is the C-integer corresponding to
dtype('p')
on this platform (see ~numpy.ctypeslib.c_intp). This base-type could be ctypes.c_int, ctypes.c_long, or ctypes.c_longlong depending on the platform. The ctypes array contains the shape of the underlying array.
- _ctypes.strides
(c_intp*self.ndim): A ctypes array of length self.ndim where the basetype is the same as for the shape attribute. This ctypes array contains the strides information from the underlying array. This strides information is important for showing how many bytes must be jumped to get to the next element in the array.
- _ctypes.data_as(obj)
Return the data pointer cast to a particular c-types object. For example, calling
self._as_parameter_
is equivalent toself.data_as(ctypes.c_void_p)
. Perhaps you want to use the data as a pointer to a ctypes array of floating-point data:self.data_as(ctypes.POINTER(ctypes.c_double))
.The returned pointer will keep a reference to the array.
- _ctypes.shape_as(obj)
Return the shape tuple as an array of some other c-types type. For example:
self.shape_as(ctypes.c_short)
.
- _ctypes.strides_as(obj)
Return the strides tuple as an array of some other c-types type. For example:
self.strides_as(ctypes.c_longlong)
.
If the ctypes module is not available, then the ctypes attribute of array objects still returns something useful, but ctypes objects are not returned and errors may be raised instead. In particular, the object will still have the
as_parameter
attribute which will return an integer equal to the data attribute.Examples#
>>> import ctypes >>> x = np.array([[0, 1], [2, 3]], dtype=np.int32) >>> x array([[0, 1], [2, 3]], dtype=int32) >>> x.ctypes.data 31962608 # may vary >>> x.ctypes.data_as(ctypes.POINTER(ctypes.c_uint32)) <__main__.LP_c_uint object at 0x7ff2fc1fc200> # may vary >>> x.ctypes.data_as(ctypes.POINTER(ctypes.c_uint32)).contents c_uint(0) >>> x.ctypes.data_as(ctypes.POINTER(ctypes.c_uint64)).contents c_ulong(4294967296) >>> x.ctypes.shape <numpy.core._internal.c_long_Array_2 object at 0x7ff2fc1fce60> # may vary >>> x.ctypes.strides <numpy.core._internal.c_long_Array_2 object at 0x7ff2fc1ff320> # may vary
- cumprod(axis=None, dtype=None, out=None)#
Return the cumulative product of the elements along the given axis.
Refer to numpy.cumprod for full documentation.
See Also#
numpy.cumprod : equivalent function
- cumsum(axis=None, dtype=None, out=None)#
Return the cumulative sum of the elements along the given axis.
Refer to numpy.cumsum for full documentation.
See Also#
numpy.cumsum : equivalent function
- data#
Python buffer object pointing to the start of the array’s data.
- diagonal(offset=0, axis1=0, axis2=1)#
Return specified diagonals. In NumPy 1.9 the returned array is a read-only view instead of a copy as in previous NumPy versions. In a future version the read-only restriction will be removed.
Refer to
numpy.diagonal()
for full documentation.See Also#
numpy.diagonal : equivalent function
- dtype#
Data-type of the array’s elements.
Warning
Setting
arr.dtype
is discouraged and may be deprecated in the future. Setting will replace thedtype
without modifying the memory (see also ndarray.view and ndarray.astype).Parameters#
None
Returns#
d : numpy dtype object
See Also#
ndarray.astype : Cast the values contained in the array to a new data-type. ndarray.view : Create a view of the same data but a different data-type. numpy.dtype
Examples#
>>> x array([[0, 1], [2, 3]]) >>> x.dtype dtype('int32') >>> type(x.dtype) <type 'numpy.dtype'>
- dump(file)#
Dump a pickle of the array to the specified file. The array can be read back with pickle.load or numpy.load.
Parameters#
- filestr or Path
A string naming the dump file.
Changed in version 1.17.0: pathlib.Path objects are now accepted.
- dumps()#
Returns the pickle of the array as a string. pickle.loads will convert the string back to an array.
Parameters#
None
- fill(value)#
Fill the array with a scalar value.
Parameters#
- valuescalar
All elements of a will be assigned this value.
Examples#
>>> a = np.array([1, 2]) >>> a.fill(0) >>> a array([0, 0]) >>> a = np.empty(2) >>> a.fill(1) >>> a array([1., 1.])
Fill expects a scalar value and always behaves the same as assigning to a single array element. The following is a rare example where this distinction is important:
>>> a = np.array([None, None], dtype=object) >>> a[0] = np.array(3) >>> a array([array(3), None], dtype=object) >>> a.fill(np.array(3)) >>> a array([array(3), array(3)], dtype=object)
Where other forms of assignments will unpack the array being assigned:
>>> a[...] = np.array(3) >>> a array([3, 3], dtype=object)
- static findinputslice(coord, sliceshape, sheetshape)[source]#
Gets the matrix indices of a slice within an array of size sheetshape from a sliceshape, positioned at coord.
- flags#
Information about the memory layout of the array.
Attributes#
- C_CONTIGUOUS (C)
The data is in a single, C-style contiguous segment.
- F_CONTIGUOUS (F)
The data is in a single, Fortran-style contiguous segment.
- OWNDATA (O)
The array owns the memory it uses or borrows it from another object.
- WRITEABLE (W)
The data area can be written to. Setting this to False locks the data, making it read-only. A view (slice, etc.) inherits WRITEABLE from its base array at creation time, but a view of a writeable array may be subsequently locked while the base array remains writeable. (The opposite is not true, in that a view of a locked array may not be made writeable. However, currently, locking a base object does not lock any views that already reference it, so under that circumstance it is possible to alter the contents of a locked array via a previously created writeable view onto it.) Attempting to change a non-writeable array raises a RuntimeError exception.
- ALIGNED (A)
The data and all elements are aligned appropriately for the hardware.
- WRITEBACKIFCOPY (X)
This array is a copy of some other array. The C-API function PyArray_ResolveWritebackIfCopy must be called before deallocating to the base array will be updated with the contents of this array.
- FNC
F_CONTIGUOUS and not C_CONTIGUOUS.
- FORC
F_CONTIGUOUS or C_CONTIGUOUS (one-segment test).
- BEHAVED (B)
ALIGNED and WRITEABLE.
- CARRAY (CA)
BEHAVED and C_CONTIGUOUS.
- FARRAY (FA)
BEHAVED and F_CONTIGUOUS and not C_CONTIGUOUS.
Notes#
The flags object can be accessed dictionary-like (as in
a.flags['WRITEABLE']
), or by using lowercased attribute names (as ina.flags.writeable
). Short flag names are only supported in dictionary access.Only the WRITEBACKIFCOPY, WRITEABLE, and ALIGNED flags can be changed by the user, via direct assignment to the attribute or dictionary entry, or by calling ndarray.setflags.
The array flags cannot be set arbitrarily:
WRITEBACKIFCOPY can only be set
False
.ALIGNED can only be set
True
if the data is truly aligned.WRITEABLE can only be set
True
if the array owns its own memory or the ultimate owner of the memory exposes a writeable buffer interface or is a string.
Arrays can be both C-style and Fortran-style contiguous simultaneously. This is clear for 1-dimensional arrays, but can also be true for higher dimensional arrays.
Even for contiguous arrays a stride for a given dimension
arr.strides[dim]
may be arbitrary ifarr.shape[dim] == 1
or the array has no elements. It does not generally hold thatself.strides[-1] == self.itemsize
for C-style contiguous arrays orself.strides[0] == self.itemsize
for Fortran-style contiguous arrays is true.
- flat#
A 1-D iterator over the array.
This is a numpy.flatiter instance, which acts similarly to, but is not a subclass of, Python’s built-in iterator object.
See Also#
flatten : Return a copy of the array collapsed into one dimension.
flatiter
Examples#
>>> x = np.arange(1, 7).reshape(2, 3) >>> x array([[1, 2, 3], [4, 5, 6]]) >>> x.flat[3] 4 >>> x.T array([[1, 4], [2, 5], [3, 6]]) >>> x.T.flat[3] 5 >>> type(x.flat) <class 'numpy.flatiter'>
An assignment example:
>>> x.flat = 3; x array([[3, 3, 3], [3, 3, 3]]) >>> x.flat[[1,4]] = 1; x array([[3, 1, 3], [3, 1, 3]])
- flatten(order='C')#
Return a copy of the array collapsed into one dimension.
Parameters#
- order{‘C’, ‘F’, ‘A’, ‘K’}, optional
‘C’ means to flatten in row-major (C-style) order. ‘F’ means to flatten in column-major (Fortran- style) order. ‘A’ means to flatten in column-major order if a is Fortran contiguous in memory, row-major order otherwise. ‘K’ means to flatten a in the order the elements occur in memory. The default is ‘C’.
Returns#
- yndarray
A copy of the input array, flattened to one dimension.
See Also#
ravel : Return a flattened array. flat : A 1-D flat iterator over the array.
Examples#
>>> a = np.array([[1,2], [3,4]]) >>> a.flatten() array([1, 2, 3, 4]) >>> a.flatten('F') array([1, 3, 2, 4])
- getfield(dtype, offset=0)#
Returns a field of the given array as a certain type.
A field is a view of the array data with a given data-type. The values in the view are determined by the given type and the offset into the current array in bytes. The offset needs to be such that the view dtype fits in the array dtype; for example an array of dtype complex128 has 16-byte elements. If taking a view with a 32-bit integer (4 bytes), the offset needs to be between 0 and 12 bytes.
Parameters#
- dtypestr or dtype
The data type of the view. The dtype size of the view can not be larger than that of the array itself.
- offsetint
Number of bytes to skip before beginning the element view.
Examples#
>>> x = np.diag([1.+1.j]*2) >>> x[1, 1] = 2 + 4.j >>> x array([[1.+1.j, 0.+0.j], [0.+0.j, 2.+4.j]]) >>> x.getfield(np.float64) array([[1., 0.], [0., 2.]])
By choosing an offset of 8 bytes we can select the complex part of the array for our view:
>>> x.getfield(np.float64, offset=8) array([[1., 0.], [0., 4.]])
- imag#
The imaginary part of the array.
Examples#
>>> x = np.sqrt([1+0j, 0+1j]) >>> x.imag array([ 0. , 0.70710678]) >>> x.imag.dtype dtype('float64')
- item(*args)#
Copy an element of an array to a standard Python scalar and return it.
Parameters#
*args : Arguments (variable number and type)
none: in this case, the method only works for arrays with one element (a.size == 1), which element is copied into a standard Python scalar object and returned.
int_type: this argument is interpreted as a flat index into the array, specifying which element to copy and return.
tuple of int_types: functions as does a single int_type argument, except that the argument is interpreted as an nd-index into the array.
Returns#
- zStandard Python scalar object
A copy of the specified element of the array as a suitable Python scalar
Notes#
When the data type of a is longdouble or clongdouble, item() returns a scalar array object because there is no available Python scalar that would not lose information. Void arrays return a buffer object for item(), unless fields are defined, in which case a tuple is returned.
item is very similar to a[args], except, instead of an array scalar, a standard Python scalar is returned. This can be useful for speeding up access to elements of the array and doing arithmetic on elements of the array using Python’s optimized math.
Examples#
>>> np.random.seed(123) >>> x = np.random.randint(9, size=(3, 3)) >>> x array([[2, 2, 6], [1, 3, 6], [1, 0, 1]]) >>> x.item(3) 1 >>> x.item(7) 0 >>> x.item((0, 1)) 2 >>> x.item((2, 2)) 1
- itemset(*args)#
Insert scalar into an array (scalar is cast to array’s dtype, if possible)
There must be at least 1 argument, and define the last argument as item. Then,
a.itemset(*args)
is equivalent to but faster thana[args] = item
. The item should be a scalar value and args must select a single item in the array a.Parameters#
- *argsArguments
If one argument: a scalar, only used in case a is of size 1. If two arguments: the last argument is the value to be set and must be a scalar, the first argument specifies a single array element location. It is either an int or a tuple.
Notes#
Compared to indexing syntax, itemset provides some speed increase for placing a scalar into a particular location in an ndarray, if you must do this. However, generally this is discouraged: among other problems, it complicates the appearance of the code. Also, when using itemset (and item) inside a loop, be sure to assign the methods to a local variable to avoid the attribute look-up at each loop iteration.
Examples#
>>> np.random.seed(123) >>> x = np.random.randint(9, size=(3, 3)) >>> x array([[2, 2, 6], [1, 3, 6], [1, 0, 1]]) >>> x.itemset(4, 0) >>> x.itemset((2, 2), 9) >>> x array([[2, 2, 6], [1, 0, 6], [1, 0, 9]])
- itemsize#
Length of one array element in bytes.
Examples#
>>> x = np.array([1,2,3], dtype=np.float64) >>> x.itemsize 8 >>> x = np.array([1,2,3], dtype=np.complex128) >>> x.itemsize 16
- max(axis=None, out=None, keepdims=False, initial=<no value>, where=True)#
Return the maximum along a given axis.
Refer to numpy.amax for full documentation.
See Also#
numpy.amax : equivalent function
- mean(axis=None, dtype=None, out=None, keepdims=False, *, where=True)#
Returns the average of the array elements along given axis.
Refer to numpy.mean for full documentation.
See Also#
numpy.mean : equivalent function
- min(axis=None, out=None, keepdims=False, initial=<no value>, where=True)#
Return the minimum along a given axis.
Refer to numpy.amin for full documentation.
See Also#
numpy.amin : equivalent function
- nbytes#
Total bytes consumed by the elements of the array.
Notes#
Does not include memory consumed by non-element attributes of the array object.
See Also#
- sys.getsizeof
Memory consumed by the object itself without parents in case view. This does include memory consumed by non-element attributes.
Examples#
>>> x = np.zeros((3,5,2), dtype=np.complex128) >>> x.nbytes 480 >>> np.prod(x.shape) * x.itemsize 480
- ndim#
Number of array dimensions.
Examples#
>>> x = np.array([1, 2, 3]) >>> x.ndim 1 >>> y = np.zeros((2, 3, 4)) >>> y.ndim 3
- newbyteorder(new_order='S', /)#
Return the array with the same data viewed with a different byte order.
Equivalent to:
arr.view(arr.dtype.newbytorder(new_order))
Changes are also made in all fields and sub-arrays of the array data type.
Parameters#
- new_orderstring, optional
Byte order to force; a value from the byte order specifications below. new_order codes can be any of:
‘S’ - swap dtype from current to opposite endian
{‘<’, ‘little’} - little endian
{‘>’, ‘big’} - big endian
{‘=’, ‘native’} - native order, equivalent to sys.byteorder
{‘|’, ‘I’} - ignore (no change to byte order)
The default value (‘S’) results in swapping the current byte order.
Returns#
- new_arrarray
New array object with the dtype reflecting given change to the byte order.
- nonzero()#
Return the indices of the elements that are non-zero.
Refer to numpy.nonzero for full documentation.
See Also#
numpy.nonzero : equivalent function
- partition(kth, axis=-1, kind='introselect', order=None)#
Rearranges the elements in the array in such a way that the value of the element in kth position is in the position it would be in a sorted array. All elements smaller than the kth element are moved before this element and all equal or greater are moved behind it. The ordering of the elements in the two partitions is undefined.
Added in version 1.8.0.
Parameters#
- kthint or sequence of ints
Element index to partition by. The kth element value will be in its final sorted position and all smaller elements will be moved before it and all equal or greater elements behind it. The order of all elements in the partitions is undefined. If provided with a sequence of kth it will partition all elements indexed by kth of them into their sorted position at once.
Deprecated since version 1.22.0: Passing booleans as index is deprecated.
- axisint, optional
Axis along which to sort. Default is -1, which means sort along the last axis.
- kind{‘introselect’}, optional
Selection algorithm. Default is ‘introselect’.
- orderstr or list of str, optional
When a is an array with fields defined, this argument specifies which fields to compare first, second, etc. A single field can be specified as a string, and not all fields need to be specified, but unspecified fields will still be used, in the order in which they come up in the dtype, to break ties.
See Also#
numpy.partition : Return a partitioned copy of an array. argpartition : Indirect partition. sort : Full sort.
Notes#
See
np.partition
for notes on the different algorithms.Examples#
>>> a = np.array([3, 4, 2, 1]) >>> a.partition(3) >>> a array([2, 1, 3, 4])
>>> a.partition((1, 3)) >>> a array([1, 2, 3, 4])
- positionedcrop(x, y, sheet_coord_system)[source]#
Offset the bounds_template to this cf’s location and store the result in the ‘bounds’ attribute.
Also stores the input_sheet_slice for access by C.
- positionlesscrop(x, y, sheet_coord_system)[source]#
Return the correct slice for a weights/mask matrix at this ConnectionField’s location on the sheet (i.e. for getting the correct submatrix of the weights or mask in case the unit is near the edge of the sheet).
- prod(axis=None, dtype=None, out=None, keepdims=False, initial=1, where=True)#
Return the product of the array elements over the given axis
Refer to numpy.prod for full documentation.
See Also#
numpy.prod : equivalent function
- ptp(axis=None, out=None, keepdims=False)#
Peak to peak (maximum - minimum) value along a given axis.
Refer to numpy.ptp for full documentation.
See Also#
numpy.ptp : equivalent function
- put(indices, values, mode='raise')#
Set
a.flat[n] = values[n]
for all n in indices.Refer to numpy.put for full documentation.
See Also#
numpy.put : equivalent function
- ravel([order])#
Return a flattened array.
Refer to numpy.ravel for full documentation.
See Also#
numpy.ravel : equivalent function
ndarray.flat : a flat iterator on the array.
- real#
The real part of the array.
Examples#
>>> x = np.sqrt([1+0j, 0+1j]) >>> x.real array([ 1. , 0.70710678]) >>> x.real.dtype dtype('float64')
See Also#
numpy.real : equivalent function
- repeat(repeats, axis=None)#
Repeat elements of an array.
Refer to numpy.repeat for full documentation.
See Also#
numpy.repeat : equivalent function
- reshape(shape, order='C')#
Returns an array containing the same data with a new shape.
Refer to numpy.reshape for full documentation.
See Also#
numpy.reshape : equivalent function
Notes#
Unlike the free function numpy.reshape, this method on ndarray allows the elements of the shape parameter to be passed in as separate arguments. For example,
a.reshape(10, 11)
is equivalent toa.reshape((10, 11))
.
- resize(new_shape, refcheck=True)#
Change shape and size of array in-place.
Parameters#
- new_shapetuple of ints, or n ints
Shape of resized array.
- refcheckbool, optional
If False, reference count will not be checked. Default is True.
Returns#
None
Raises#
- ValueError
If a does not own its own data or references or views to it exist, and the data memory must be changed. PyPy only: will always raise if the data memory must be changed, since there is no reliable way to determine if references or views to it exist.
- SystemError
If the order keyword argument is specified. This behaviour is a bug in NumPy.
See Also#
resize : Return a new array with the specified shape.
Notes#
This reallocates space for the data area if necessary.
Only contiguous arrays (data elements consecutive in memory) can be resized.
The purpose of the reference count check is to make sure you do not use this array as a buffer for another Python object and then reallocate the memory. However, reference counts can increase in other ways so if you are sure that you have not shared the memory for this array with another Python object, then you may safely set refcheck to False.
Examples#
Shrinking an array: array is flattened (in the order that the data are stored in memory), resized, and reshaped:
>>> a = np.array([[0, 1], [2, 3]], order='C') >>> a.resize((2, 1)) >>> a array([[0], [1]])
>>> a = np.array([[0, 1], [2, 3]], order='F') >>> a.resize((2, 1)) >>> a array([[0], [2]])
Enlarging an array: as above, but missing entries are filled with zeros:
>>> b = np.array([[0, 1], [2, 3]]) >>> b.resize(2, 3) # new_shape parameter doesn't have to be a tuple >>> b array([[0, 1, 2], [3, 0, 0]])
Referencing an array prevents resizing…
>>> c = a >>> a.resize((1, 1)) Traceback (most recent call last): ... ValueError: cannot resize an array that references or is referenced ...
Unless refcheck is False:
>>> a.resize((1, 1), refcheck=False) >>> a array([[0]]) >>> c array([[0]])
- round(decimals=0, out=None)#
Return a with each element rounded to the given number of decimals.
Refer to numpy.around for full documentation.
See Also#
numpy.around : equivalent function
- searchsorted(v, side='left', sorter=None)#
Find indices where elements of v should be inserted in a to maintain order.
For full documentation, see numpy.searchsorted
See Also#
numpy.searchsorted : equivalent function
- setfield(val, dtype, offset=0)#
Put a value into a specified place in a field defined by a data-type.
Place val into a’s field defined by dtype and beginning offset bytes into the field.
Parameters#
- valobject
Value to be placed in field.
- dtypedtype object
Data-type of the field in which to place val.
- offsetint, optional
The number of bytes into the field at which to place val.
Returns#
None
See Also#
getfield
Examples#
>>> x = np.eye(3) >>> x.getfield(np.float64) array([[1., 0., 0.], [0., 1., 0.], [0., 0., 1.]]) >>> x.setfield(3, np.int32) >>> x.getfield(np.int32) array([[3, 3, 3], [3, 3, 3], [3, 3, 3]], dtype=int32) >>> x array([[1.0e+000, 1.5e-323, 1.5e-323], [1.5e-323, 1.0e+000, 1.5e-323], [1.5e-323, 1.5e-323, 1.0e+000]]) >>> x.setfield(np.eye(3), np.int32) >>> x array([[1., 0., 0.], [0., 1., 0.], [0., 0., 1.]])
- setflags(write=None, align=None, uic=None)#
Set array flags WRITEABLE, ALIGNED, WRITEBACKIFCOPY, respectively.
These Boolean-valued flags affect how numpy interprets the memory area used by a (see Notes below). The ALIGNED flag can only be set to True if the data is actually aligned according to the type. The WRITEBACKIFCOPY and flag can never be set to True. The flag WRITEABLE can only be set to True if the array owns its own memory, or the ultimate owner of the memory exposes a writeable buffer interface, or is a string. (The exception for string is made so that unpickling can be done without copying memory.)
Parameters#
- writebool, optional
Describes whether or not a can be written to.
- alignbool, optional
Describes whether or not a is aligned properly for its type.
- uicbool, optional
Describes whether or not a is a copy of another “base” array.
Notes#
Array flags provide information about how the memory area used for the array is to be interpreted. There are 7 Boolean flags in use, only four of which can be changed by the user: WRITEBACKIFCOPY, WRITEABLE, and ALIGNED.
WRITEABLE (W) the data area can be written to;
ALIGNED (A) the data and strides are aligned appropriately for the hardware (as determined by the compiler);
WRITEBACKIFCOPY (X) this array is a copy of some other array (referenced by .base). When the C-API function PyArray_ResolveWritebackIfCopy is called, the base array will be updated with the contents of this array.
All flags can be accessed using the single (upper case) letter as well as the full name.
Examples#
>>> y = np.array([[3, 1, 7], ... [2, 0, 0], ... [8, 5, 9]]) >>> y array([[3, 1, 7], [2, 0, 0], [8, 5, 9]]) >>> y.flags C_CONTIGUOUS : True F_CONTIGUOUS : False OWNDATA : True WRITEABLE : True ALIGNED : True WRITEBACKIFCOPY : False >>> y.setflags(write=0, align=0) >>> y.flags C_CONTIGUOUS : True F_CONTIGUOUS : False OWNDATA : True WRITEABLE : False ALIGNED : False WRITEBACKIFCOPY : False >>> y.setflags(uic=1) Traceback (most recent call last): File "<stdin>", line 1, in <module> ValueError: cannot set WRITEBACKIFCOPY flag to True
- shape#
Tuple of array dimensions.
The shape property is usually used to get the current shape of an array, but may also be used to reshape the array in-place by assigning a tuple of array dimensions to it. As with numpy.reshape, one of the new shape dimensions can be -1, in which case its value is inferred from the size of the array and the remaining dimensions. Reshaping an array in-place will fail if a copy is required.
Warning
Setting
arr.shape
is discouraged and may be deprecated in the future. Using ndarray.reshape is the preferred approach.Examples#
>>> x = np.array([1, 2, 3, 4]) >>> x.shape (4,) >>> y = np.zeros((2, 3, 4)) >>> y.shape (2, 3, 4) >>> y.shape = (3, 8) >>> y array([[ 0., 0., 0., 0., 0., 0., 0., 0.], [ 0., 0., 0., 0., 0., 0., 0., 0.], [ 0., 0., 0., 0., 0., 0., 0., 0.]]) >>> y.shape = (3, 6) Traceback (most recent call last): File "<stdin>", line 1, in <module> ValueError: total size of new array must be unchanged >>> np.zeros((4,2))[::2].shape = (-1,) Traceback (most recent call last): File "<stdin>", line 1, in <module> AttributeError: Incompatible shape for in-place modification. Use `.reshape()` to make a copy with the desired shape.
See Also#
numpy.shape : Equivalent getter function. numpy.reshape : Function similar to setting
shape
. ndarray.reshape : Method similar to settingshape
.
- size#
Number of elements in the array.
Equal to
np.prod(a.shape)
, i.e., the product of the array’s dimensions.Notes#
a.size returns a standard arbitrary precision Python integer. This may not be the case with other methods of obtaining the same value (like the suggested
np.prod(a.shape)
, which returns an instance ofnp.int_
), and may be relevant if the value is used further in calculations that may overflow a fixed size integer type.Examples#
>>> x = np.zeros((3, 5, 2), dtype=np.complex128) >>> x.size 30 >>> np.prod(x.shape) 30
- sort(axis=-1, kind=None, order=None)#
Sort an array in-place. Refer to numpy.sort for full documentation.
Parameters#
- axisint, optional
Axis along which to sort. Default is -1, which means sort along the last axis.
- kind{‘quicksort’, ‘mergesort’, ‘heapsort’, ‘stable’}, optional
Sorting algorithm. The default is ‘quicksort’. Note that both ‘stable’ and ‘mergesort’ use timsort under the covers and, in general, the actual implementation will vary with datatype. The ‘mergesort’ option is retained for backwards compatibility.
Changed in version 1.15.0: The ‘stable’ option was added.
- orderstr or list of str, optional
When a is an array with fields defined, this argument specifies which fields to compare first, second, etc. A single field can be specified as a string, and not all fields need be specified, but unspecified fields will still be used, in the order in which they come up in the dtype, to break ties.
See Also#
numpy.sort : Return a sorted copy of an array. numpy.argsort : Indirect sort. numpy.lexsort : Indirect stable sort on multiple keys. numpy.searchsorted : Find elements in sorted array. numpy.partition: Partial sort.
Notes#
See numpy.sort for notes on the different sorting algorithms.
Examples#
>>> a = np.array([[1,4], [3,1]]) >>> a.sort(axis=1) >>> a array([[1, 4], [1, 3]]) >>> a.sort(axis=0) >>> a array([[1, 3], [1, 4]])
Use the order keyword to specify a field to use when sorting a structured array:
>>> a = np.array([('a', 2), ('c', 1)], dtype=[('x', 'S1'), ('y', int)]) >>> a.sort(order='y') >>> a array([(b'c', 1), (b'a', 2)], dtype=[('x', 'S1'), ('y', '<i8')])
- squeeze(axis=None)#
Remove axes of length one from a.
Refer to numpy.squeeze for full documentation.
See Also#
numpy.squeeze : equivalent function
- std(axis=None, dtype=None, out=None, ddof=0, keepdims=False, *, where=True)#
Returns the standard deviation of the array elements along given axis.
Refer to numpy.std for full documentation.
See Also#
numpy.std : equivalent function
- strides#
Tuple of bytes to step in each dimension when traversing an array.
The byte offset of element
(i[0], i[1], ..., i[n])
in an array a is:offset = sum(np.array(i) * a.strides)
A more detailed explanation of strides can be found in the “ndarray.rst” file in the NumPy reference guide.
Warning
Setting
arr.strides
is discouraged and may be deprecated in the future. numpy.lib.stride_tricks.as_strided should be preferred to create a new view of the same data in a safer way.Notes#
Imagine an array of 32-bit integers (each 4 bytes):
x = np.array([[0, 1, 2, 3, 4], [5, 6, 7, 8, 9]], dtype=np.int32)
This array is stored in memory as 40 bytes, one after the other (known as a contiguous block of memory). The strides of an array tell us how many bytes we have to skip in memory to move to the next position along a certain axis. For example, we have to skip 4 bytes (1 value) to move to the next column, but 20 bytes (5 values) to get to the same position in the next row. As such, the strides for the array x will be
(20, 4)
.See Also#
numpy.lib.stride_tricks.as_strided
Examples#
>>> y = np.reshape(np.arange(2*3*4), (2,3,4)) >>> y array([[[ 0, 1, 2, 3], [ 4, 5, 6, 7], [ 8, 9, 10, 11]], [[12, 13, 14, 15], [16, 17, 18, 19], [20, 21, 22, 23]]]) >>> y.strides (48, 16, 4) >>> y[1,1,1] 17 >>> offset=sum(y.strides * np.array((1,1,1))) >>> offset/y.itemsize 17
>>> x = np.reshape(np.arange(5*6*7*8), (5,6,7,8)).transpose(2,3,1,0) >>> x.strides (32, 4, 224, 1344) >>> i = np.array([3,5,2,2]) >>> offset = sum(i * x.strides) >>> x[3,5,2,2] 813 >>> offset / x.itemsize 813
- submatrix(matrix)[source]#
Return the submatrix of the given matrix specified by this slice.
Equivalent to computing the intersection between the SheetCoordinateSystem’s bounds and the bounds, and returning the corresponding submatrix of the given matrix.
The submatrix is just a view into the sheet_matrix; it is not an independent copy.
- sum(axis=None, dtype=None, out=None, keepdims=False, initial=0, where=True)#
Return the sum of the array elements over the given axis.
Refer to numpy.sum for full documentation.
See Also#
numpy.sum : equivalent function
- swapaxes(axis1, axis2)#
Return a view of the array with axis1 and axis2 interchanged.
Refer to numpy.swapaxes for full documentation.
See Also#
numpy.swapaxes : equivalent function
- take(indices, axis=None, out=None, mode='raise')#
Return an array formed from the elements of a at the given indices.
Refer to numpy.take for full documentation.
See Also#
numpy.take : equivalent function
- tobytes(order='C')#
Construct Python bytes containing the raw data bytes in the array.
Constructs Python bytes showing a copy of the raw contents of data memory. The bytes object is produced in C-order by default. This behavior is controlled by the
order
parameter.Added in version 1.9.0.
Parameters#
- order{‘C’, ‘F’, ‘A’}, optional
Controls the memory layout of the bytes object. ‘C’ means C-order, ‘F’ means F-order, ‘A’ (short for Any) means ‘F’ if a is Fortran contiguous, ‘C’ otherwise. Default is ‘C’.
Returns#
- sbytes
Python bytes exhibiting a copy of a’s raw data.
See also#
- frombuffer
Inverse of this operation, construct a 1-dimensional array from Python bytes.
Examples#
>>> x = np.array([[0, 1], [2, 3]], dtype='<u2') >>> x.tobytes() b'\x00\x00\x01\x00\x02\x00\x03\x00' >>> x.tobytes('C') == x.tobytes() True >>> x.tobytes('F') b'\x00\x00\x02\x00\x01\x00\x03\x00'
- tofile(fid, sep='', format='%s')#
Write array to a file as text or binary (default).
Data is always written in ‘C’ order, independent of the order of a. The data produced by this method can be recovered using the function fromfile().
Parameters#
- fidfile or str or Path
An open file object, or a string containing a filename.
Changed in version 1.17.0: pathlib.Path objects are now accepted.
- sepstr
Separator between array items for text output. If “” (empty), a binary file is written, equivalent to
file.write(a.tobytes())
.- formatstr
Format string for text file output. Each entry in the array is formatted to text by first converting it to the closest Python type, and then using “format” % item.
Notes#
This is a convenience function for quick storage of array data. Information on endianness and precision is lost, so this method is not a good choice for files intended to archive data or transport data between machines with different endianness. Some of these problems can be overcome by outputting the data as text files, at the expense of speed and file size.
When fid is a file object, array contents are directly written to the file, bypassing the file object’s
write
method. As a result, tofile cannot be used with files objects supporting compression (e.g., GzipFile) or file-like objects that do not supportfileno()
(e.g., BytesIO).
- tolist()#
Return the array as an
a.ndim
-levels deep nested list of Python scalars.Return a copy of the array data as a (nested) Python list. Data items are converted to the nearest compatible builtin Python type, via the ~numpy.ndarray.item function.
If
a.ndim
is 0, then since the depth of the nested list is 0, it will not be a list at all, but a simple Python scalar.Parameters#
none
Returns#
- yobject, or list of object, or list of list of object, or …
The possibly nested list of array elements.
Notes#
The array may be recreated via
a = np.array(a.tolist())
, although this may sometimes lose precision.Examples#
For a 1D array,
a.tolist()
is almost the same aslist(a)
, except thattolist
changes numpy scalars to Python scalars:>>> a = np.uint32([1, 2]) >>> a_list = list(a) >>> a_list [1, 2] >>> type(a_list[0]) <class 'numpy.uint32'> >>> a_tolist = a.tolist() >>> a_tolist [1, 2] >>> type(a_tolist[0]) <class 'int'>
Additionally, for a 2D array,
tolist
applies recursively:>>> a = np.array([[1, 2], [3, 4]]) >>> list(a) [array([1, 2]), array([3, 4])] >>> a.tolist() [[1, 2], [3, 4]]
The base case for this recursion is a 0D array:
>>> a = np.array(1) >>> list(a) Traceback (most recent call last): ... TypeError: iteration over a 0-d array >>> a.tolist() 1
- tostring(order='C')#
A compatibility alias for tobytes, with exactly the same behavior.
Despite its name, it returns bytes not strs.
Deprecated since version 1.19.0.
- trace(offset=0, axis1=0, axis2=1, dtype=None, out=None)#
Return the sum along diagonals of the array.
Refer to numpy.trace for full documentation.
See Also#
numpy.trace : equivalent function
- transpose(*axes)#
Returns a view of the array with axes transposed.
Refer to numpy.transpose for full documentation.
Parameters#
axes : None, tuple of ints, or n ints
None or no argument: reverses the order of the axes.
tuple of ints: i in the j-th place in the tuple means that the array’s i-th axis becomes the transposed array’s j-th axis.
n ints: same as an n-tuple of the same ints (this form is intended simply as a “convenience” alternative to the tuple form).
Returns#
- pndarray
View of the array with its axes suitably permuted.
See Also#
transpose : Equivalent function. ndarray.T : Array property returning the array transposed. ndarray.reshape : Give a new shape to an array without changing its data.
Examples#
>>> a = np.array([[1, 2], [3, 4]]) >>> a array([[1, 2], [3, 4]]) >>> a.transpose() array([[1, 3], [2, 4]]) >>> a.transpose((1, 0)) array([[1, 3], [2, 4]]) >>> a.transpose(1, 0) array([[1, 3], [2, 4]])
>>> a = np.array([1, 2, 3, 4]) >>> a array([1, 2, 3, 4]) >>> a.transpose() array([1, 2, 3, 4])
- var(axis=None, dtype=None, out=None, ddof=0, keepdims=False, *, where=True)#
Returns the variance of the array elements, along given axis.
Refer to numpy.var for full documentation.
See Also#
numpy.var : equivalent function
- view([dtype][, type])#
New view of array with the same data.
Note
Passing None for
dtype
is different from omitting the parameter, since the former invokesdtype(None)
which is an alias fordtype('float_')
.Parameters#
- dtypedata-type or ndarray sub-class, optional
Data-type descriptor of the returned view, e.g., float32 or int16. Omitting it results in the view having the same data-type as a. This argument can also be specified as an ndarray sub-class, which then specifies the type of the returned object (this is equivalent to setting the
type
parameter).- typePython type, optional
Type of the returned view, e.g., ndarray or matrix. Again, omission of the parameter results in type preservation.
Notes#
a.view()
is used two different ways:a.view(some_dtype)
ora.view(dtype=some_dtype)
constructs a view of the array’s memory with a different data-type. This can cause a reinterpretation of the bytes of memory.a.view(ndarray_subclass)
ora.view(type=ndarray_subclass)
just returns an instance of ndarray_subclass that looks at the same array (same shape, dtype, etc.) This does not cause a reinterpretation of the memory.For
a.view(some_dtype)
, ifsome_dtype
has a different number of bytes per entry than the previous dtype (for example, converting a regular array to a structured array), then the last axis ofa
must be contiguous. This axis will be resized in the result.Changed in version 1.23.0: Only the last axis needs to be contiguous. Previously, the entire array had to be C-contiguous.
Examples#
>>> x = np.array([(1, 2)], dtype=[('a', np.int8), ('b', np.int8)])
Viewing array data using a different type and dtype:
>>> y = x.view(dtype=np.int16, type=np.matrix) >>> y matrix([[513]], dtype=int16) >>> print(type(y)) <class 'numpy.matrix'>
Creating a view on a structured array so it can be used in calculations
>>> x = np.array([(1, 2),(3,4)], dtype=[('a', np.int8), ('b', np.int8)]) >>> xv = x.view(dtype=np.int8).reshape(-1,2) >>> xv array([[1, 2], [3, 4]], dtype=int8) >>> xv.mean(0) array([2., 3.])
Making changes to the view changes the underlying array
>>> xv[0,1] = 20 >>> x array([(1, 20), (3, 4)], dtype=[('a', 'i1'), ('b', 'i1')])
Using a view to convert an array to a recarray:
>>> z = x.view(np.recarray) >>> z.a array([1, 3], dtype=int8)
Views share data:
>>> x[0] = (9, 10) >>> z[0] (9, 10)
Views that change the dtype size (bytes per entry) should normally be avoided on arrays defined by slices, transposes, fortran-ordering, etc.:
>>> x = np.array([[1, 2, 3], [4, 5, 6]], dtype=np.int16) >>> y = x[:, ::2] >>> y array([[1, 3], [4, 6]], dtype=int16) >>> y.view(dtype=[('width', np.int16), ('length', np.int16)]) Traceback (most recent call last): ... ValueError: To change to a dtype of a different size, the last axis must be contiguous >>> z = y.copy() >>> z.view(dtype=[('width', np.int16), ('length', np.int16)]) array([[(1, 3)], [(4, 6)]], dtype=[('width', '<i2'), ('length', '<i2')])
However, views that change dtype are totally fine for arrays with a contiguous last axis, even if the rest of the axes are not C-contiguous:
>>> x = np.arange(2 * 3 * 4, dtype=np.int8).reshape(2, 3, 4) >>> x.transpose(1, 0, 2).view(np.int16) array([[[ 256, 770], [3340, 3854]], [[1284, 1798], [4368, 4882]], [[2312, 2826], [5396, 5910]]], dtype=int16)
spaces
Module#

- class holoviews.core.spaces.Callable(callable, **params)[source]#
Bases:
Parameterized
Callable allows wrapping callbacks on one or more DynamicMaps allowing their inputs (and in future outputs) to be defined. This makes it possible to wrap DynamicMaps with streams and makes it possible to traverse the graph of operations applied to a DynamicMap.
Additionally, if the memoize attribute is True, a Callable will memoize the last returned value based on the arguments to the function and the state of all streams on its inputs, to avoid calling the function unnecessarily. Note that because memoization includes the streams found on the inputs it may be disabled if the stream requires it and is triggering.
A Callable may also specify a stream_mapping which specifies the objects that are associated with interactive (i.e. linked) streams when composite objects such as Layouts are returned from the callback. This is required for building interactive, linked visualizations (for the backends that support them) when returning Layouts, NdLayouts or GridSpace objects. When chaining multiple DynamicMaps into a pipeline, the link_inputs parameter declares whether the visualization generated using this Callable will inherit the linked streams. This parameter is used as a hint by the applicable backend.
The mapping should map from an appropriate key to a list of streams associated with the selected object. The appropriate key may be a type[.group][.label] specification for Layouts, an integer index or a suitable NdLayout/GridSpace key. For more information see the DynamicMap tutorial at holoviews.org.
callable
= param.Callable(allow_None=True, allow_refs=False, constant=True, label=’Callable’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c2d9450>)The callable function being wrapped.
inputs
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Inputs’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c48acd0>)The list of inputs the callable function is wrapping. Used to allow deep access to streams in chained Callables.
operation_kwargs
= param.Dict(allow_refs=False, class_=<class ‘dict’>, constant=True, default={}, label=’Operation kwargs’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c489cd0>)Potential dynamic keyword arguments associated with the operation.
link_inputs
= param.Boolean(allow_refs=False, default=True, label=’Link inputs’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c48a750>)If the Callable wraps around other DynamicMaps in its inputs, determines whether linked streams attached to the inputs are transferred to the objects returned by the Callable. For example the Callable wraps a DynamicMap with an RangeXY stream, this switch determines whether the corresponding visualization should update this stream with range changes originating from the newly generated axes.
memoize
= param.Boolean(allow_refs=False, default=True, label=’Memoize’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c489bd0>)Whether the return value of the callable should be memoized based on the call arguments and any streams attached to the inputs.
operation
= param.Callable(allow_None=True, allow_refs=False, label=’Operation’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c48add0>)The function being applied by the Callable. May be used to record the transform(s) being applied inside the callback function.
stream_mapping
= param.Dict(allow_refs=False, class_=<class ‘dict’>, constant=True, default={}, label=’Stream mapping’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c489cd0>)Defines how streams should be mapped to objects returned by the Callable, e.g. when it returns a Layout.
- clone(callable=None, **overrides)[source]#
Clones the Callable optionally with new settings
- Args:
callable: New callable function to wrap **overrides: Parameter overrides to apply
- Returns:
Cloned Callable object
- property noargs#
Returns True if the callable takes no arguments
- class holoviews.core.spaces.DynamicMap(callback, initial_items=None, streams=None, **params)[source]#
Bases:
HoloMap
A DynamicMap is a type of HoloMap where the elements are dynamically generated by a callable. The callable is invoked with values associated with the key dimensions or with values supplied by stream parameters.
Parameters inherited from:
kdims
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c48bb50>)The key dimensions of a DynamicMap map to the arguments of the callback. This mapping can be by position or by name.
callback
= param.ClassSelector(allow_None=True, allow_refs=False, class_=<class ‘holoviews.core.spaces.Callable’>, constant=True, label=’Callback’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c482cd0>)The callable used to generate the elements. The arguments to the callable includes any number of declared key dimensions as well as any number of stream parameters defined on the input streams. If the callable is an instance of Callable it will be used directly, otherwise it will be automatically wrapped in one.
streams
= param.List(allow_refs=False, bounds=(0, None), constant=True, default=[], label=’Streams’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c48bdd0>)List of Stream instances to associate with the DynamicMap. The set of parameter values across these streams will be supplied as keyword arguments to the callback when the events are received, updating the streams. Can also be supplied as a dictionary that maps parameters or panel widgets to callback argument names that will then be automatically converted to the equivalent list format.
cache_size
= param.Integer(allow_refs=False, bounds=(1, None), default=500, inclusive_bounds=(True, True), label=’Cache size’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c483e90>)The number of entries to cache for fast access. This is an LRU cache where the least recently used item is overwritten once the cache is full.
positional_stream_args
= param.Boolean(allow_refs=False, constant=True, default=False, label=’Positional stream args’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c489250>)If False, stream parameters are passed to the callback as keyword arguments. If True, stream parameters are passed to callback as positional arguments. Each positional argument is a dict containing the contents of a stream. The positional stream arguments follow the positional arguments for each kdim, and they are ordered to match the order of the DynamicMap’s streams list.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- collate()[source]#
Unpacks DynamicMap into container of DynamicMaps
Collation allows unpacking DynamicMaps which return Layout, NdLayout or GridSpace objects into a single such object containing DynamicMaps. Assumes that the items in the layout or grid that is returned do not change.
- Returns:
Collated container containing DynamicMaps
- property current_key#
Returns the current key value.
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs DynamicMap of nested DynamicMaps into a single DynamicMap that returns a non-dynamic element
Decollation allows packing a DynamicMap of nested DynamicMaps into a single DynamicMap that returns a simple (non-dynamic) element. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a non-dynamic element
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- event(**kwargs)[source]#
Updates attached streams and triggers events
Automatically find streams matching the supplied kwargs to update and trigger events on them.
- Args:
**kwargs: Events to update streams with
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- grid(dimensions=None, **kwargs)[source]#
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a GridSpace.
Args: dimensions: Dimension/str or list
Dimension or list of dimensions to group by
Returns: grid: GridSpace
GridSpace with supplied dimensions
- property group#
Group inherited from items
- groupby(dimensions=None, container_type=None, group_type=None, **kwargs)[source]#
Groups DynamicMap by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of DynamicMap and adjoined histogram if adjoin=True, otherwise just the histogram
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- layout(dimensions=None, **kwargs)[source]#
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a NdLayout.
Args: dimensions: Dimension/str or list
Dimension or list of dimensions to group by
Returns: layout: NdLayout
NdLayout with supplied dimensions
- map(map_fn, specs=None, clone=True, link_inputs=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options defined in a flat format to the objects returned by the DynamicMap. If the options are to be set directly on the objects returned by the DynamicMap a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- overlay(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and overlay each group
Groups data by supplied dimension(s) overlaying the groups along the dimension(s).
- Args:
dimensions: Dimension(s) of dimensions to group by
- Returns:
NdOverlay object(s) with supplied dimensions
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reorders key dimensions on DynamicMap
Create a new object with a reordered set of key dimensions. Dropping dimensions is not allowed on a DynamicMap.
- Args:
kdims: List of dimensions to reindex the mapping with force: Not applicable to a DynamicMap
- Returns:
Reindexed DynamicMap
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- property unbounded#
Returns a list of key dimensions that are unbounded, excluding stream parameters. If any of these key dimensions are unbounded, the DynamicMap as a whole is also unbounded.
- class holoviews.core.spaces.Generator(callable, **params)[source]#
Bases:
Callable
Generators are considered a special case of Callable that accept no arguments and never memoize.
Parameters inherited from:
holoviews.core.spaces.Callable
: inputs, operation_kwargs, link_inputs, memoize, operation, stream_mappingcallable
= param.ClassSelector(allow_None=True, allow_refs=False, class_=<class ‘generator’>, constant=True, label=’Callable’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c4cbf90>)The generator that is wrapped by this Generator.
- clone(callable=None, **overrides)[source]#
Clones the Callable optionally with new settings
- Args:
callable: New callable function to wrap **overrides: Parameter overrides to apply
- Returns:
Cloned Callable object
- property noargs#
Returns True if the callable takes no arguments
- class holoviews.core.spaces.GridMatrix(initial_items=None, kdims=None, **params)[source]#
Bases:
GridSpace
GridMatrix is container type for heterogeneous Element types laid out in a grid. Unlike a GridSpace the axes of the Grid must not represent an actual coordinate space, but may be used to plot various dimensions against each other. The GridMatrix is usually constructed using the gridmatrix operation, which will generate a GridMatrix plotting each dimension in an Element against each other.
Parameters inherited from:
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace
Decollation allows packing a GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a GridSpace
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- keys(full_grid=False)[source]#
Returns the keys of the GridSpace
- Args:
full_grid (bool, optional): Return full cross-product of keys
- Returns:
List of keys
- property label#
Label inherited from items
- property last#
The last of a GridSpace is another GridSpace constituted of the last of the individual elements. To access the elements by their X,Y position, either index the position directly or use the items() method.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property shape#
Returns the 2D shape of the GridSpace as (rows, cols).
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.spaces.GridSpace(initial_items=None, kdims=None, **params)[source]#
Bases:
Layoutable
,UniformNdMapping
Grids are distinct from Layouts as they ensure all contained elements to be of the same type. Unlike Layouts, which have integer keys, Grids usually have floating point keys, which correspond to a grid sampling in some two-dimensional space. This two-dimensional space may have to arbitrary dimensions, e.g. for 2D parameter spaces.
Parameters inherited from:
kdims
= param.List(allow_refs=False, bounds=(1, 2), default=[Dimension(‘X’), Dimension(‘Y’)], label=’Kdims’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15c4eafd0>)The key dimensions defined as list of dimensions that may be used in indexing (and potential slicing) semantics. The order of the dimensions listed here determines the semantics of each component of a multi-dimensional indexing operation. Aliased with key_dimensions.
- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace
Decollation allows packing a GridSpace of DynamicMaps into a single DynamicMap that returns a GridSpace of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns a GridSpace
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- keys(full_grid=False)[source]#
Returns the keys of the GridSpace
- Args:
full_grid (bool, optional): Return full cross-product of keys
- Returns:
List of keys
- property label#
Label inherited from items
- property last#
The last of a GridSpace is another GridSpace constituted of the last of the individual elements. To access the elements by their X,Y position, either index the position directly or use the items() method.
- property last_key#
Returns the last key value.
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, clone=True, **kwargs)[source]#
Applies simplified option definition returning a new object.
Applies options on an object or nested group of objects in a flat format returning a new object with the options applied. If the options are to be set directly on the object a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
Identical to the .opts method but returns a clone of the object by default.
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=0)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- property shape#
Returns the 2D shape of the GridSpace as (rows, cols).
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- class holoviews.core.spaces.HoloMap(initial_items=None, kdims=None, group=None, label=None, **params)[source]#
Bases:
Layoutable
,UniformNdMapping
,Overlayable
A HoloMap is an n-dimensional mapping of viewable elements or overlays. Each item in a HoloMap has an tuple key defining the values along each of the declared key dimensions, defining the discretely sampled space of values.
The visual representation of a HoloMap consists of the viewable objects inside the HoloMap which can be explored by varying one or more widgets mapping onto the key dimensions of the HoloMap.
Parameters inherited from:
holoviews.core.dimension.LabelledData
: labelholoviews.core.dimension.Dimensioned
: cdimsholoviews.core.ndmapping.MultiDimensionalMapping
: kdims, vdims, sort- add_dimension(dimension, dim_pos, dim_val, vdim=False, **kwargs)[source]#
Adds a dimension and its values to the object
Requires the dimension name or object, the desired position in the key dimensions and a key value scalar or sequence of the same length as the existing keys.
- Args:
dimension: Dimension or dimension spec to add dim_pos (int) Integer index to insert dimension at dim_val (scalar or ndarray): Dimension value(s) to add vdim: Disabled, this type does not have value dimensions **kwargs: Keyword arguments passed to the cloned element
- Returns:
Cloned object containing the new dimension
- clone(data=None, shared_data=True, new_type=None, link=True, *args, **overrides)[source]#
Clones the object, overriding data and parameters.
- Args:
data: New data replacing the existing data shared_data (bool, optional): Whether to use existing data new_type (optional): Type to cast object to link (bool, optional): Whether clone should be linked
Determines whether Streams and Links attached to original object will be inherited.
*args: Additional arguments to pass to constructor **overrides: New keyword arguments to pass to constructor
- Returns:
Cloned object
- collapse(dimensions=None, function=None, spreadfn=None, **kwargs)[source]#
Concatenates and aggregates along supplied dimensions
Useful to collapse stacks of objects into a single object, e.g. to average a stack of Images or Curves.
- Args:
- dimensions: Dimension(s) to collapse
Defaults to all key dimensions
function: Aggregation function to apply, e.g. numpy.mean spreadfn: Secondary reduction to compute value spread
Useful for computing a confidence interval, spread, or standard deviation.
**kwargs: Keyword arguments passed to the aggregation function
- Returns:
Returns the collapsed element or HoloMap of collapsed elements
- collate(merge_type=None, drop=None, drop_constant=False)[source]#
Collate allows reordering nested containers
Collation allows collapsing nested mapping types by merging their dimensions. In simple terms in merges nested containers into a single merged type.
In the simple case a HoloMap containing other HoloMaps can easily be joined in this way. However collation is particularly useful when the objects being joined are deeply nested, e.g. you want to join multiple Layouts recorded at different times, collation will return one Layout containing HoloMaps indexed by Time. Changing the merge_type will allow merging the outer Dimension into any other UniformNdMapping type.
- Args:
merge_type: Type of the object to merge with drop: List of dimensions to drop drop_constant: Drop constant dimensions automatically
- Returns:
Collated Layout or HoloMap
- property ddims#
The list of deep dimensions
- decollate()[source]#
Packs HoloMap of DynamicMaps into a single DynamicMap that returns an HoloMap
Decollation allows packing a HoloMap of DynamicMaps into a single DynamicMap that returns an HoloMap of simple (non-dynamic) elements. All nested streams are lifted to the resulting DynamicMap, and are available in the streams property. The callback property of the resulting DynamicMap is a pure, stateless function of the stream values. To avoid stream parameter name conflicts, the resulting DynamicMap is configured with positional_stream_args=True, and the callback function accepts stream values as positional dict arguments.
- Returns:
DynamicMap that returns an HoloMap
- dframe(dimensions=None, multi_index=False)[source]#
Convert dimension values to DataFrame.
Returns a pandas dataframe of columns along each dimension, either completely flat or indexed by key dimensions.
- Args:
dimensions: Dimensions to return as columns multi_index: Convert key dimensions to (multi-)index
- Returns:
DataFrame of columns corresponding to each dimension
- dimension_values(dimension, expanded=True, flat=True)[source]#
Return the values along the requested dimension.
- Args:
dimension: The dimension to return values for expanded (bool, optional): Whether to expand values
Whether to return the expanded values, behavior depends on the type of data:
Columnar: If false returns unique values
Geometry: If false returns scalar values per geometry
Gridded: If false returns 1D coordinates
flat (bool, optional): Whether to flatten array
- Returns:
NumPy array of values along the requested dimension
- dimensions(selection='all', label=False)[source]#
Lists the available dimensions on the object
Provides convenient access to Dimensions on nested Dimensioned objects. Dimensions can be selected by their type, i.e. ‘key’ or ‘value’ dimensions. By default ‘all’ dimensions are returned.
- Args:
- selection: Type of dimensions to return
The type of dimension, i.e. one of ‘key’, ‘value’, ‘constant’ or ‘all’.
- label: Whether to return the name, label or Dimension
Whether to return the Dimension objects (False), the Dimension names (True/’name’) or labels (‘label’).
- Returns:
List of Dimension objects or their names or labels
- drop_dimension(dimensions)[source]#
Drops dimension(s) from keys
- Args:
dimensions: Dimension(s) to drop
- Returns:
Clone of object with with dropped dimension(s)
- get_dimension(dimension, default=None, strict=False)[source]#
Get a Dimension object by name or index.
- Args:
dimension: Dimension to look up by name or integer index default (optional): Value returned if Dimension not found strict (bool, optional): Raise a KeyError if not found
- Returns:
Dimension object for the requested dimension or default
- get_dimension_index(dimension)[source]#
Get the index of the requested dimension.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Integer index of the requested dimension
- get_dimension_type(dim)[source]#
Get the type of the requested dimension.
Type is determined by Dimension.type attribute or common type of the dimension values, otherwise None.
- Args:
dimension: Dimension to look up by name or by index
- Returns:
Declared type of values along the dimension
- grid(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and lay out groups in grid
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a GridSpace.
Args: dimensions: Dimension/str or list
Dimension or list of dimensions to group by
- Returns:
GridSpace with supplied dimensions
- property group#
Group inherited from items
- groupby(dimensions, container_type=None, group_type=None, **kwargs)[source]#
Groups object by one or more dimensions
Applies groupby operation over the specified dimensions returning an object of type container_type (expected to be dictionary-like) containing the groups.
- Args:
dimensions: Dimension(s) to group by container_type: Type to cast group container to group_type: Type to cast each group to dynamic: Whether to return a DynamicMap **kwargs: Keyword arguments to pass to each group
- Returns:
Returns object of supplied container_type containing the groups. If dynamic=True returns a DynamicMap instead.
- hist(dimension=None, num_bins=20, bin_range=None, adjoin=True, individually=True, **kwargs)[source]#
Computes and adjoins histogram along specified dimension(s).
Defaults to first value dimension if present otherwise falls back to first key dimension.
- Args:
dimension: Dimension(s) to compute histogram on num_bins (int, optional): Number of bins bin_range (tuple optional): Lower and upper bounds of bins adjoin (bool, optional): Whether to adjoin histogram
- Returns:
AdjointLayout of HoloMap and histograms or just the histograms
- property info#
Prints information about the Dimensioned object, including the number and type of objects contained within it and information about its dimensions.
- property label#
Label inherited from items
- property last#
Returns the item highest data item along the map dimensions.
- property last_key#
Returns the last key value.
- layout(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and lay out groups
Groups data by supplied dimension(s) laying the groups along the dimension(s) out in a NdLayout.
- Args:
dimensions: Dimension(s) to group by
- Returns:
NdLayout with supplied dimensions
- map(map_fn, specs=None, clone=True)[source]#
Map a function to all objects matching the specs
Recursively replaces elements using a map function when the specs apply, by default applies to all objects, e.g. to apply the function to all contained Curve objects:
dmap.map(fn, hv.Curve)
- Args:
map_fn: Function to apply to each object specs: List of specs to match
List of types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
clone: Whether to clone the object or transform inplace
- Returns:
Returns the object after the map_fn has been applied
- matches(spec)[source]#
Whether the spec applies to this object.
- Args:
- spec: A function, spec or type to check for a match
A ‘type[[.group].label]’ string which is compared against the type, group and label of this object
A function which is given the object and returns a boolean.
An object type matched using isinstance.
- Returns:
bool: Whether the spec matched this object.
- options(*args, **kwargs)[source]#
Applies simplified option definition returning a new object
Applies options defined in a flat format to the objects returned by the DynamicMap. If the options are to be set directly on the objects in the HoloMap a simple format may be used, e.g.:
obj.options(cmap=’viridis’, show_title=False)
If the object is nested the options must be qualified using a type[.group][.label] specification, e.g.:
obj.options(‘Image’, cmap=’viridis’, show_title=False)
or using:
obj.options({‘Image’: dict(cmap=’viridis’, show_title=False)})
- Args:
- *args: Sets of options to apply to object
Supports a number of formats including lists of Options objects, a type[.group][.label] followed by a set of keyword options to apply and a dictionary indexed by type[.group][.label] specs.
- backend (optional): Backend to apply options to
Defaults to current selected backend
- clone (bool, optional): Whether to clone object
Options can be applied inplace with clone=False
- **kwargs: Keywords of options
Set of options to apply to the object
- Returns:
Returns the cloned object with the options applied
- overlay(dimensions=None, **kwargs)[source]#
Group by supplied dimension(s) and overlay each group
Groups data by supplied dimension(s) overlaying the groups along the dimension(s).
- Args:
dimensions: Dimension(s) of dimensions to group by
- Returns:
NdOverlay object(s) with supplied dimensions
- range(dimension, data_range=True, dimension_range=True)[source]#
Return the lower and upper bounds of values along dimension.
- Args:
dimension: The dimension to compute the range on. data_range (bool): Compute range from data values dimension_range (bool): Include Dimension ranges
Whether to include Dimension range and soft_range in range calculation
- Returns:
Tuple containing the lower and upper bound
- reindex(kdims=None, force=False)[source]#
Reindexes object dropping static or supplied kdims
Creates a new object with a reordered or reduced set of key dimensions. By default drops all non-varying key dimensions.
Reducing the number of key dimensions will discard information from the keys. All data values are accessible in the newly created object as the new labels must be sufficient to address each value uniquely.
- Args:
kdims (optional): New list of key dimensions after reindexing force (bool, optional): Whether to drop non-unique items
- Returns:
Reindexed object
- relabel(label=None, group=None, depth=1)[source]#
Clone object and apply new group and/or label.
Applies relabeling to children up to the supplied depth.
- Args:
label (str, optional): New label to apply to returned object group (str, optional): New group to apply to returned object depth (int, optional): Depth to which relabel will be applied
If applied to container allows applying relabeling to contained objects up to the specified depth
- Returns:
Returns relabelled object
- select(selection_specs=None, **kwargs)[source]#
Applies selection by dimension name
Applies a selection along the dimensions of the object using keyword arguments. The selection may be narrowed to certain objects using selection_specs. For container objects the selection will be applied to all children as well.
Selections may select a specific value, slice or set of values:
- value: Scalar values will select rows along with an exact
match, e.g.:
ds.select(x=3)
- slice: Slices may be declared as tuples of the upper and
lower bound, e.g.:
ds.select(x=(0, 3))
- values: A list of values may be selected using a list or
set, e.g.:
ds.select(x=[0, 1, 2])
- Args:
- selection_specs: List of specs to match on
A list of types, functions, or type[.group][.label] strings specifying which objects to apply the selection on.
- **selection: Dictionary declaring selections by dimension
Selections can be scalar values, tuple ranges, lists of discrete values and boolean arrays
- Returns:
Returns an Dimensioned object containing the selected data or a scalar if a single value was selected
- traverse(fn=None, specs=None, full_breadth=True)[source]#
Traverses object returning matching items Traverses the set of children of the object, collecting the all objects matching the defined specs. Each object can be processed with the supplied function. Args:
fn (function, optional): Function applied to matched objects specs: List of specs to match
Specs must be types, functions or type[.group][.label] specs to select objects to return, by default applies to all objects.
- full_breadth: Whether to traverse all objects
Whether to traverse the full set of objects on each container or only the first.
- Returns:
list: List of objects that matched
- property type#
The type of elements stored in the mapping.
- holoviews.core.spaces.dynamicmap_memoization(callable_obj, streams)[source]#
Determine whether the Callable should have memoization enabled based on the supplied streams (typically by a DynamicMap). Memoization is disabled if any of the streams require it it and are currently in a triggered state.
- holoviews.core.spaces.get_nested_dmaps(dmap)[source]#
Recurses DynamicMap to find DynamicMaps inputs
- Args:
dmap: DynamicMap to recurse to look for DynamicMap inputs
- Returns:
List of DynamicMap instances that were found
traversal
Module#
Advanced utilities for traversing nesting/hierarchical Dimensioned objects either to inspect the structure of their declared dimensions or mutate the matching elements.
- holoviews.core.traversal.hierarchical(keys)[source]#
Iterates over dimension values in keys, taking two sets of dimension values at a time to determine whether two consecutive dimensions have a one-to-many relationship. If they do a mapping between the first and second dimension values is returned. Returns a list of n-1 mappings, between consecutive dimensions.
tree
Module#

- class holoviews.core.tree.AttrTree(items=None, identifier=None, parent=None, dir_mode='default')[source]#
Bases:
object
An AttrTree offers convenient, multi-level attribute access for collections of objects. AttrTree objects may also be combined together using the update method or merge classmethod. Here is an example of adding a ViewableElement to an AttrTree and accessing it:
>>> t = AttrTree() >>> t.Example.Path = 1 >>> t.Example.Path 1
- property fixed#
If fixed, no new paths can be created via attribute access
- get(identifier, default=None)[source]#
Get a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The indexed node of the AttrTree
- property path#
Returns the path up to the root for the current node.
- pop(identifier, default=None)[source]#
Pop a node of the AttrTree using its path string.
- Args:
identifier: Path string of the node to return default: Value to return if no node is found
- Returns:
The node that was removed from the AttrTree
- set_path(path, val)[source]#
Set the given value at the supplied path where path is either a tuple of strings or a string in A.B.C format.
util
Module#
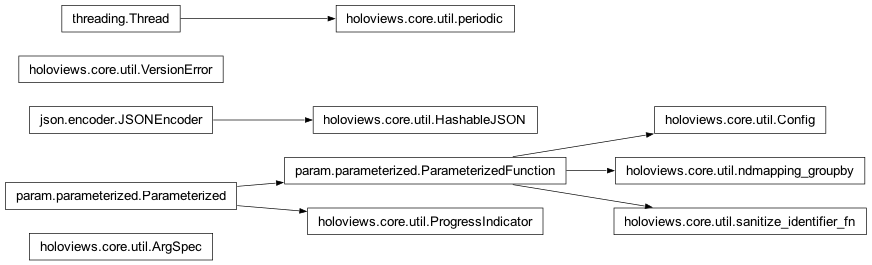
- class holoviews.core.util.ArgSpec(args, varargs, keywords, defaults)#
Bases:
tuple
- args#
Alias for field number 0
- count(value, /)#
Return number of occurrences of value.
- defaults#
Alias for field number 3
- index(value, start=0, stop=9223372036854775807, /)#
Return first index of value.
Raises ValueError if the value is not present.
- keywords#
Alias for field number 2
- varargs#
Alias for field number 1
- class holoviews.core.util.Config(*, default_cmap, default_gridded_cmap, default_heatmap_cmap, future_deprecations, image_rtol, no_padding, warn_options_call, name)[source]#
Bases:
ParameterizedFunction
Set of boolean configuration values to change HoloViews’ global behavior. Typically used to control warnings relating to deprecations or set global parameter such as style ‘themes’.
future_deprecations
= param.Boolean(allow_refs=False, default=False, label=’Future deprecations’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ca8d4d0>)Whether to warn about future deprecations
image_rtol
= param.Number(allow_refs=False, default=0.001, inclusive_bounds=(True, True), label=’Image rtol’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ca8e690>)The tolerance used to enforce regular sampling for regular, gridded data where regular sampling is expected. Expressed as the maximal allowable sampling difference between sample locations.
no_padding
= param.Boolean(allow_refs=False, default=False, label=’No padding’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ca8d550>)Disable default padding (introduced in 1.13.0).
warn_options_call
= param.Boolean(allow_refs=False, default=True, label=’Warn options call’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ca8d6d0>)Whether to warn when the deprecated __call__ options syntax is used (the opts method should now be used instead). It is recommended that users switch this on to update any uses of __call__ as it will be deprecated in future.
default_cmap
= param.String(allow_refs=False, default=’kbc_r’, label=’Default cmap’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ca8d550>)Global default colormap. Prior to HoloViews 1.14.0, the default value was ‘fire’ which can be set for backwards compatibility.
default_gridded_cmap
= param.String(allow_refs=False, default=’kbc_r’, label=’Default gridded cmap’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ca8d6d0>)Global default colormap for gridded elements (i.e. Image, Raster and QuadMesh). Can be set to ‘fire’ to match raster defaults prior to HoloViews 1.14.0 while allowing the default_cmap to be the value of ‘kbc_r’ used in HoloViews >= 1.14.0
default_heatmap_cmap
= param.String(allow_refs=False, default=’kbc_r’, label=’Default heatmap cmap’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ca8de10>)Global default colormap for HeatMap elements. Prior to HoloViews 1.14.0, the default value was the ‘RdYlBu_r’ colormap.
- class holoviews.core.util.HashableJSON(*, skipkeys=False, ensure_ascii=True, check_circular=True, allow_nan=True, sort_keys=False, indent=None, separators=None, default=None)[source]#
Bases:
JSONEncoder
Extends JSONEncoder to generate a hashable string for as many types of object as possible including nested objects and objects that are not normally hashable. The purpose of this class is to generate unique strings that once hashed are suitable for use in memoization and other cases where deep equality must be tested without storing the entire object.
By default JSONEncoder supports booleans, numbers, strings, lists, tuples and dictionaries. In order to support other types such as sets, datetime objects and mutable objects such as pandas Dataframes or numpy arrays, HashableJSON has to convert these types to datastructures that can normally be represented as JSON.
Support for other object types may need to be introduced in future. By default, unrecognized object types are represented by their id.
One limitation of this approach is that dictionaries with composite keys (e.g. tuples) are not supported due to the JSON spec.
- default(obj)[source]#
Implement this method in a subclass such that it returns a serializable object for
o
, or calls the base implementation (to raise aTypeError
).For example, to support arbitrary iterators, you could implement default like this:
def default(self, o): try: iterable = iter(o) except TypeError: pass else: return list(iterable) # Let the base class default method raise the TypeError return super().default(o)
- class holoviews.core.util.ProgressIndicator(*, label, percent_range, name)[source]#
Bases:
Parameterized
Baseclass for any ProgressIndicator that indicates progress as a completion percentage.
percent_range
= param.NumericTuple(allow_refs=False, default=(0.0, 100.0), label=’Percent range’, length=2, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15ccb8b50>)The total percentage spanned by the progress bar when called with a value between 0% and 100%. This allows an overall completion in percent to be broken down into smaller sub-tasks that individually complete to 100 percent.
label
= param.String(allow_None=True, allow_refs=False, default=’Progress’, label=’Label’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cdcf350>)The label of the current progress bar.
- exception holoviews.core.util.VersionError(msg, version=None, min_version=None, **kwargs)[source]#
Bases:
Exception
Raised when there is a library version mismatch.
- add_note()#
Exception.add_note(note) – add a note to the exception
- with_traceback()#
Exception.with_traceback(tb) – set self.__traceback__ to tb and return self.
- holoviews.core.util.arglexsort(arrays)[source]#
Returns the indices of the lexicographical sorting order of the supplied arrays.
- holoviews.core.util.argspec(callable_obj)[source]#
Returns an ArgSpec object for functions, staticmethods, instance methods, classmethods and partials.
Note that the args list for instance and class methods are those as seen by the user. In other words, the first argument which is conventionally called ‘self’ or ‘cls’ is omitted in these cases.
- holoviews.core.util.asarray(arraylike, strict=True)[source]#
Converts arraylike objects to NumPy ndarray types. Errors if object is not arraylike and strict option is enabled.
- holoviews.core.util.bound_range(vals, density, time_unit='us')[source]#
Computes a bounding range and density from a number of samples assumed to be evenly spaced. Density is rounded to machine precision using significant digits reported by sys.float_info.dig.
- holoviews.core.util.callable_name(callable_obj)[source]#
Attempt to return a meaningful name identifying a callable or generator
- holoviews.core.util.capitalize_unicode_name(s)[source]#
Turns a string such as ‘capital delta’ into the shortened, capitalized version, in this case simply ‘Delta’. Used as a transform in sanitize_identifier.
- holoviews.core.util.cartesian_product(arrays, flat=True, copy=False)[source]#
Efficient cartesian product of a list of 1D arrays returning the expanded array views for each dimensions. By default arrays are flattened, which may be controlled with the flat flag. The array views can be turned into regular arrays with the copy flag.
- holoviews.core.util.cast_array_to_int64(array)[source]#
Convert a numpy array to int64. Suppress the following warning emitted by Numpy, which as of 12/2021 has been extensively discussed (pandas-dev/pandas#22384) and whose fate (possible revert) has not yet been settled:
FutureWarning: casting datetime64[ns] values to int64 with .astype(…) is deprecated and will raise in a future version. Use .view(…) instead.
- holoviews.core.util.cftime_to_timestamp(date, time_unit='us')[source]#
Converts cftime to timestamp since epoch in milliseconds
Non-standard calendars (e.g. Julian or no leap calendars) are converted to standard Gregorian calendar. This can cause extra space to be added for dates that don’t exist in the original calendar. In order to handle these dates correctly a custom bokeh model with support for other calendars would have to be defined.
- Args:
date: cftime datetime object (or array)
- Returns:
time_unit since 1970-01-01 00:00:00
- holoviews.core.util.closest_match(match, specs, depth=0)[source]#
Recursively iterates over type, group, label and overlay key, finding the closest matching spec.
- holoviews.core.util.compute_density(start, end, length, time_unit='us')[source]#
Computes a grid density given the edges and number of samples. Handles datetime grids correctly by computing timedeltas and computing a density for the given time_unit.
- holoviews.core.util.compute_edges(edges)[source]#
Computes edges as midpoints of the bin centers. The first and last boundaries are equidistant from the first and last midpoints respectively.
- holoviews.core.util.cross_index(values, index)[source]#
Allows efficiently indexing into a cartesian product without expanding it. The values should be defined as a list of iterables making up the cartesian product and a linear index, returning the cross product of the values at the supplied index.
- holoviews.core.util.date_range(start, end, length, time_unit='us')[source]#
Computes a date range given a start date, end date and the number of samples.
- holoviews.core.util.deephash(obj)[source]#
Given an object, return a hash using HashableJSON. This hash is not architecture, Python version or platform independent.
- holoviews.core.util.deprecated_opts_signature(args, kwargs)[source]#
Utility to help with the deprecation of the old .opts method signature
Returns whether opts.apply_groups should be used (as a bool) and the corresponding options.
- holoviews.core.util.dimension_range(lower, upper, hard_range, soft_range, padding=None, log=False)[source]#
Computes the range along a dimension by combining the data range with the Dimension soft_range and range.
- holoviews.core.util.dimension_sort(odict, kdims, vdims, key_index)[source]#
Sorts data by key using usual Python tuple sorting semantics or sorts in categorical order for any categorical Dimensions.
- holoviews.core.util.dimensioned_streams(dmap)[source]#
Given a DynamicMap return all streams that have any dimensioned parameters, i.e. parameters also listed in the key dimensions.
- holoviews.core.util.dimensionless_contents(streams, kdims, no_duplicates=True)[source]#
Return a list of stream parameters that have not been associated with any of the key dimensions.
- holoviews.core.util.disable_constant(parameterized)[source]#
Temporarily set parameters on Parameterized object to constant=False.
- holoviews.core.util.drop_streams(streams, kdims, keys)[source]#
Drop any dimensioned streams from the keys and kdims.
- holoviews.core.util.dt64_to_dt(dt64)[source]#
Safely converts NumPy datetime64 to a datetime object.
- holoviews.core.util.dt_to_int(value, time_unit='us')[source]#
Converts a datetime type to an integer with the supplied time unit.
- holoviews.core.util.expand_grid_coords(dataset, dim)[source]#
Expand the coordinates along a dimension of the gridded dataset into an ND-array matching the dimensionality of the dataset.
- holoviews.core.util.find_minmax(lims, olims)[source]#
Takes (a1, a2) and (b1, b2) as input and returns (np.nanmin(a1, b1), np.nanmax(a2, b2)). Used to calculate min and max values of a number of items.
- holoviews.core.util.find_range(values, soft_range=None)[source]#
Safely finds either the numerical min and max of a set of values, falling back to the first and the last value in the sorted list of values.
- holoviews.core.util.flatten(line)[source]#
Flatten an arbitrarily nested sequence.
Inspired by: pd.core.common.flatten
Parameters#
- linesequence
The sequence to flatten
Notes#
This only flattens list, tuple, and dict sequences.
Returns#
flattened : generator
- holoviews.core.util.get_method_owner(method)[source]#
Gets the instance that owns the supplied method
- holoviews.core.util.get_ndmapping_label(ndmapping, attr)[source]#
Function to get the first non-auxiliary object label attribute from an NdMapping.
- holoviews.core.util.get_overlay_spec(o, k, v)[source]#
Gets the type.group.label + key spec from an Element in an Overlay.
- holoviews.core.util.get_path(item)[source]#
Gets a path from an Labelled object or from a tuple of an existing path and a labelled object. The path strings are sanitized and capitalized.
- holoviews.core.util.group_select(selects, length=None, depth=None)[source]#
Given a list of key tuples to select, groups them into sensible chunks to avoid duplicating indexing operations.
- holoviews.core.util.int_to_alpha(n, upper=True)[source]#
Generates alphanumeric labels of form A-Z, AA-ZZ etc.
- holoviews.core.util.is_cyclic(graph)[source]#
Return True if the directed graph g has a cycle. The directed graph should be represented as a dictionary mapping of edges for each node.
- holoviews.core.util.is_dataframe(data)[source]#
Checks whether the supplied data is of DataFrame type.
- holoviews.core.util.is_int(obj, int_like=False)[source]#
Checks for int types including the native Python type and NumPy-like objects
- Args:
obj: Object to check for integer type int_like (boolean): Check for float types with integer value
- Returns:
Boolean indicating whether the supplied value is of integer type.
- holoviews.core.util.is_param_method(obj, has_deps=False)[source]#
Whether the object is a method on a parameterized object.
- Args:
obj: Object to check has_deps (boolean, optional): Check for dependencies
Whether to also check whether the method has been annotated with param.depends
- Returns:
A boolean value indicating whether the object is a method on a Parameterized object and if enabled whether it has any dependencies
- holoviews.core.util.isdatetime(value)[source]#
Whether the array or scalar is recognized datetime type.
- holoviews.core.util.isequal(value1, value2)[source]#
Compare two values, returning a boolean.
Will apply the comparison to all elements of an array/dataframe.
- holoviews.core.util.isfinite(val)[source]#
Helper function to determine if scalar or array value is finite extending np.isfinite with support for None, string, datetime types.
- holoviews.core.util.isnat(val)[source]#
Checks if the value is a NaT. Should only be called on datetimelike objects.
- holoviews.core.util.iterative_select(obj, dimensions, selects, depth=None)[source]#
Takes the output of group_select selecting subgroups iteratively, avoiding duplicating select operations.
- holoviews.core.util.layer_groups(ordering, length=2)[source]#
Splits a global ordering of Layers into groups based on a slice of the spec. The grouping behavior can be modified by changing the length of spec the entries are grouped by.
- holoviews.core.util.layer_sort(hmap)[source]#
Find a global ordering for layers in a HoloMap of CompositeOverlay types.
- holoviews.core.util.make_path_unique(path, counts, new)[source]#
Given a path, a list of existing paths and counts for each of the existing paths.
- holoviews.core.util.match_spec(element, specification)[source]#
Matches the group.label specification of the supplied element against the supplied specification dictionary returning the value of the best match.
- holoviews.core.util.max_extents(extents, zrange=False)[source]#
Computes the maximal extent in 2D and 3D space from list of 4-tuples or 6-tuples. If zrange is enabled all extents are converted to 6-tuples to compute x-, y- and z-limits.
- holoviews.core.util.max_range(ranges, combined=True)[source]#
Computes the maximal lower and upper bounds from a list bounds.
- Args:
ranges (list of tuples): A list of range tuples combined (boolean, optional): Whether to combine bounds
Whether range should be computed on lower and upper bound independently or both at once
- Returns:
The maximum range as a single tuple
- holoviews.core.util.merge_dimensions(dimensions_list)[source]#
Merges lists of fully or partially overlapping dimensions by merging their values.
>>> from holoviews import Dimension >>> dim_list = [[Dimension('A', values=[1, 2, 3]), Dimension('B')], ... [Dimension('A', values=[2, 3, 4])]] >>> dimensions = merge_dimensions(dim_list) >>> dimensions [Dimension('A'), Dimension('B')] >>> dimensions[0].values [1, 2, 3, 4]
- holoviews.core.util.merge_option_dicts(old_opts, new_opts)[source]#
Update the old_opts option dictionary with the options defined in new_opts. Instead of a shallow update as would be performed by calling old_opts.update(new_opts), this updates the dictionaries of all option types separately.
- Given two dictionaries
old_opts = {‘a’: {‘x’: ‘old’, ‘y’: ‘old’}}
- and
new_opts = {‘a’: {‘y’: ‘new’, ‘z’: ‘new’}, ‘b’: {‘k’: ‘new’}}
- this returns a dictionary
{‘a’: {‘x’: ‘old’, ‘y’: ‘new’, ‘z’: ‘new’}, ‘b’: {‘k’: ‘new’}}
- holoviews.core.util.merge_options_to_dict(options)[source]#
Given a collection of Option objects or partial option dictionaries, merge everything to a single dictionary.
- class holoviews.core.util.ndmapping_groupby(*, sort, name)[source]#
Bases:
ParameterizedFunction
Apply a groupby operation to an NdMapping, using pandas to improve performance (if available).
sort
= param.Boolean(allow_refs=False, default=False, label=’Sort’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cc60590>)Whether to apply a sorted groupby
- holoviews.core.util.numpy_scalar_to_python(scalar)[source]#
Converts a NumPy scalar to a regular python type.
- holoviews.core.util.one_to_one(graph, nodes)[source]#
Return True if graph contains only one to one mappings. The directed graph should be represented as a dictionary mapping of edges for each node. Nodes should be passed a simple list.
- holoviews.core.util.parse_datetime(date)[source]#
Parses dates specified as string or integer or pandas Timestamp
- holoviews.core.util.parse_datetime_selection(sel)[source]#
Parses string selection specs as datetimes.
- class holoviews.core.util.periodic(period, count, callback, timeout=None, block=False)[source]#
Bases:
Thread
Run a callback count times with a given period without blocking.
If count is None, will run till timeout (which may be forever if None).
- property daemon#
A boolean value indicating whether this thread is a daemon thread.
This must be set before start() is called, otherwise RuntimeError is raised. Its initial value is inherited from the creating thread; the main thread is not a daemon thread and therefore all threads created in the main thread default to daemon = False.
The entire Python program exits when only daemon threads are left.
- getName()[source]#
Return a string used for identification purposes only.
This method is deprecated, use the name attribute instead.
- property ident#
Thread identifier of this thread or None if it has not been started.
This is a nonzero integer. See the get_ident() function. Thread identifiers may be recycled when a thread exits and another thread is created. The identifier is available even after the thread has exited.
- isDaemon()[source]#
Return whether this thread is a daemon.
This method is deprecated, use the daemon attribute instead.
- is_alive()[source]#
Return whether the thread is alive.
This method returns True just before the run() method starts until just after the run() method terminates. See also the module function enumerate().
- join(timeout=None)[source]#
Wait until the thread terminates.
This blocks the calling thread until the thread whose join() method is called terminates – either normally or through an unhandled exception or until the optional timeout occurs.
When the timeout argument is present and not None, it should be a floating point number specifying a timeout for the operation in seconds (or fractions thereof). As join() always returns None, you must call is_alive() after join() to decide whether a timeout happened – if the thread is still alive, the join() call timed out.
When the timeout argument is not present or None, the operation will block until the thread terminates.
A thread can be join()ed many times.
join() raises a RuntimeError if an attempt is made to join the current thread as that would cause a deadlock. It is also an error to join() a thread before it has been started and attempts to do so raises the same exception.
- property name#
A string used for identification purposes only.
It has no semantics. Multiple threads may be given the same name. The initial name is set by the constructor.
- property native_id#
Native integral thread ID of this thread, or None if it has not been started.
This is a non-negative integer. See the get_native_id() function. This represents the Thread ID as reported by the kernel.
- run()[source]#
Method representing the thread’s activity.
You may override this method in a subclass. The standard run() method invokes the callable object passed to the object’s constructor as the target argument, if any, with sequential and keyword arguments taken from the args and kwargs arguments, respectively.
- setDaemon(daemonic)[source]#
Set whether this thread is a daemon.
This method is deprecated, use the .daemon property instead.
- holoviews.core.util.process_ellipses(obj, key, vdim_selection=False)[source]#
Helper function to pad a __getitem__ key with the right number of empty slices (i.e. :) when the key contains an Ellipsis (…).
If the vdim_selection flag is true, check if the end of the key contains strings or Dimension objects in obj. If so, extra padding will not be applied for the value dimensions (i.e. the resulting key will be exactly one longer than the number of kdims). Note: this flag should not be used for composite types.
- holoviews.core.util.range_pad(lower, upper, padding=None, log=False)[source]#
Pads the range by a fraction of the interval
- holoviews.core.util.rename_stream_kwargs(stream, kwargs, reverse=False)[source]#
Given a stream and a kwargs dictionary of parameter values, map to the corresponding dictionary where the keys are substituted with the appropriately renamed string.
If reverse, the output will be a dictionary using the original parameter names given a dictionary using the renamed equivalents.
- holoviews.core.util.resolve_dependent_kwargs(kwargs)[source]#
Resolves parameter dependencies in the supplied dictionary
Resolves parameter values, Parameterized instance methods and parameterized functions with dependencies in the supplied dictionary.
- Args:
kwargs (dict): A dictionary of keyword arguments
- Returns:
A new dictionary where any parameter dependencies have been resolved.
- holoviews.core.util.resolve_dependent_value(value)[source]#
Resolves parameter dependencies on the supplied value
Resolves parameter values, Parameterized instance methods, parameterized functions with dependencies on the supplied value, including such parameters embedded in a list, tuple, dictionary, or slice.
- Args:
value: A value which will be resolved
- Returns:
A new value where any parameter dependencies have been resolved.
- class holoviews.core.util.sanitize_identifier_fn(*, _lookup_table, aliases, capitalize, disable_leading_underscore, disallowed, eliminations, substitutions, transforms, name)[source]#
Bases:
ParameterizedFunction
Sanitizes group/label values for use in AttrTree attribute access.
Special characters are sanitized using their (lowercase) unicode name using the unicodedata module. For instance:
>>> unicodedata.name(u'$').lower() 'dollar sign'
As these names are often very long, this parameterized function allows filtered, substitutions and transforms to help shorten these names appropriately.
capitalize
= param.Boolean(allow_refs=False, default=True, label=’Capitalize’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cdce810>)Whether the first letter should be converted to uppercase. Note, this will only be applied to ASCII characters in order to make sure paths aren’t confused with method names.
eliminations
= param.List(allow_refs=False, bounds=(0, None), default=[‘extended’, ‘accent’, ‘small’, ‘letter’, ‘sign’, ‘digit’, ‘latin’, ‘greek’, ‘arabic-indic’, ‘with’, ‘dollar’], label=’Eliminations’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cc73d10>)Lowercase strings to be eliminated from the unicode names in order to shorten the sanitized name ( lowercase). Redundant strings should be removed but too much elimination could cause two unique strings to map to the same sanitized output.
substitutions
= param.Dict(allow_refs=False, class_=<class ‘dict’>, default={‘circumflex’: ‘power’, ‘asterisk’: ‘times’, ‘solidus’: ‘over’}, label=’Substitutions’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cce15d0>)Lowercase substitutions of substrings in unicode names. For instance the ^ character has the name ‘circumflex accent’ even though it is more typically used for exponentiation. Note that substitutions occur after filtering and that there should be no ordering dependence between substitutions.
transforms
= param.List(allow_refs=False, bounds=(0, None), default=[<function capitalize_unicode_name at 0x11465a340>], label=’Transforms’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cc729d0>)List of string transformation functions to apply after filtering and substitution in order to further compress the unicode name. For instance, the default capitalize_unicode_name function will turn the string “capital delta” into “Delta”.
disallowed
= param.List(allow_refs=False, bounds=(0, None), default=[‘trait_names’, ‘_ipython_display_’, ‘_getAttributeNames’], label=’Disallowed’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cce15d0>)An explicit list of name that should not be allowed as attribute names on Tree objects. By default, prevents IPython from creating an entry called Trait_names due to an inconvenient getattr check (during tab-completion).
disable_leading_underscore
= param.Boolean(allow_refs=False, default=False, label=’Disable leading underscore’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cc72ad0>)Whether leading underscores should be allowed to be sanitized with the leading prefix.
aliases
= param.Dict(allow_refs=False, class_=<class ‘dict’>, default={}, label=’Aliases’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cc73d10>)A dictionary of aliases mapping long strings to their short, sanitized equivalents
_lookup_table
= param.Dict(allow_refs=False, class_=<class ‘dict’>, default={}, label=’ lookup table’, nested_refs=False, rx=<param.reactive.reactive_ops object at 0x15cc73a90>)Cache of previously computed sanitizations
- add_aliases(**kwargs)[source]#
Conveniently add new aliases as keyword arguments. For instance you can add a new alias with add_aliases(short=’Longer string’)
- classmethod instance(**params)[source]#
Return an instance of this class, copying parameters from any existing instance provided.
- prefixed(identifier)[source]#
Whether or not the identifier will be prefixed. Strings that require the prefix are generally not recommended.
- holoviews.core.util.search_indices(values, source)[source]#
Given a set of values returns the indices of each of those values in the source array.
- holoviews.core.util.sort_topologically(graph)[source]#
Stackless topological sorting.
- graph = {
3: [1], 5: [3], 4: [2], 6: [4],
}
sort_topologically(graph) [[1, 2], [3, 4], [5, 6]]
- holoviews.core.util.stream_name_mapping(stream, exclude_params=None, reverse=False)[source]#
Return a complete dictionary mapping between stream parameter names to their applicable renames, excluding parameters listed in exclude_params.
If reverse is True, the mapping is from the renamed strings to the original stream parameter names.
- holoviews.core.util.stream_parameters(streams, no_duplicates=True, exclude=None)[source]#
Given a list of streams, return a flat list of parameter name, excluding those listed in the exclude list.
If no_duplicates is enabled, a KeyError will be raised if there are parameter name clashes across the streams.
- holoviews.core.util.tree_attribute(identifier)[source]#
Predicate that returns True for custom attributes added to AttrTrees that are not methods, properties or internal attributes.
These custom attributes start with a capitalized character when applicable (not applicable to underscore or certain unicode characters)
- holoviews.core.util.unbound_dimensions(streams, kdims, no_duplicates=True)[source]#
Return a list of dimensions that have not been associated with any streams.
- holoviews.core.util.unique_array(arr)[source]#
Returns an array of unique values in the input order.
- Args:
arr (np.ndarray or list): The array to compute unique values on
- Returns:
A new array of unique values
- holoviews.core.util.unique_iterator(seq)[source]#
Returns an iterator containing all non-duplicate elements in the input sequence.
- holoviews.core.util.validate_dynamic_argspec(callback, kdims, streams)[source]#
Utility used by DynamicMap to ensure the supplied callback has an appropriate signature.
If validation succeeds, returns a list of strings to be zipped with the positional arguments, i.e. kdim values. The zipped values can then be merged with the stream values to pass everything to the Callable as keywords.
If the callbacks use *args, None is returned to indicate that kdim values must be passed to the Callable by position. In this situation, Callable passes *args and **kwargs directly to the callback.
If the callback doesn’t use **kwargs, the accepted keywords are validated against the stream parameter names.
Subpackages#
- holoviews.data Package
data
PackageDataConversion
Dataset
Dataset.add_dimension()
Dataset.aggregate()
Dataset.array()
Dataset.clone()
Dataset.closest()
Dataset.columns()
Dataset.compute()
Dataset.dataset
Dataset.ddims
Dataset.dframe()
Dataset.dimension_values()
Dataset.dimensions()
Dataset.get_dimension()
Dataset.get_dimension_index()
Dataset.get_dimension_type()
Dataset.groupby()
Dataset.hist()
Dataset.iloc
Dataset.map()
Dataset.matches()
Dataset.ndloc
Dataset.options()
Dataset.persist()
Dataset.pipeline
Dataset.range()
Dataset.reduce()
Dataset.reindex()
Dataset.relabel()
Dataset.sample()
Dataset.select()
Dataset.shape
Dataset.sort()
Dataset.to
Dataset.transform()
Dataset.traverse()
PipelineMeta
concat()
disable_pipeline()
array
ModuleArrayInterface
ArrayInterface.applies()
ArrayInterface.as_dframe()
ArrayInterface.cast()
ArrayInterface.compute()
ArrayInterface.concatenate()
ArrayInterface.indexed()
ArrayInterface.isunique()
ArrayInterface.loaded()
ArrayInterface.persist()
ArrayInterface.replace_value()
ArrayInterface.select_mask()
ArrayInterface.unpack_scalar()
cudf
ModulecuDFInterface
cuDFInterface.applies()
cuDFInterface.as_dframe()
cuDFInterface.cast()
cuDFInterface.compute()
cuDFInterface.concatenate()
cuDFInterface.indexed()
cuDFInterface.isunique()
cuDFInterface.loaded()
cuDFInterface.persist()
cuDFInterface.replace_value()
cuDFInterface.select_mask()
cuDFInterface.unpack_scalar()
dask
ModuleDaskInterface
DaskInterface.applies()
DaskInterface.as_dframe()
DaskInterface.cast()
DaskInterface.compute()
DaskInterface.concatenate()
DaskInterface.iloc()
DaskInterface.indexed()
DaskInterface.isunique()
DaskInterface.loaded()
DaskInterface.persist()
DaskInterface.replace_value()
DaskInterface.select_mask()
DaskInterface.unpack_scalar()
dictionary
ModuleDictInterface
DictInterface.applies()
DictInterface.as_dframe()
DictInterface.cast()
DictInterface.compute()
DictInterface.concatenate()
DictInterface.indexed()
DictInterface.isunique()
DictInterface.loaded()
DictInterface.persist()
DictInterface.replace_value()
DictInterface.select_mask()
DictInterface.unpack_scalar()
grid
ModuleGridInterface
GridInterface.applies()
GridInterface.as_dframe()
GridInterface.canonicalize()
GridInterface.cast()
GridInterface.compute()
GridInterface.concatenate()
GridInterface.coords()
GridInterface.indexed()
GridInterface.isunique()
GridInterface.loaded()
GridInterface.persist()
GridInterface.replace_value()
GridInterface.sample()
GridInterface.select_mask()
GridInterface.unpack_scalar()
ibis
ModuleIbisInterface
IbisInterface.applies()
IbisInterface.as_dframe()
IbisInterface.cast()
IbisInterface.compute()
IbisInterface.concatenate()
IbisInterface.indexed()
IbisInterface.isunique()
IbisInterface.loaded()
IbisInterface.persist()
IbisInterface.replace_value()
IbisInterface.select_mask()
IbisInterface.unpack_scalar()
image
ModuleImageInterface
ImageInterface.applies()
ImageInterface.as_dframe()
ImageInterface.canonicalize()
ImageInterface.cast()
ImageInterface.compute()
ImageInterface.concatenate()
ImageInterface.coords()
ImageInterface.indexed()
ImageInterface.irregular()
ImageInterface.isunique()
ImageInterface.loaded()
ImageInterface.persist()
ImageInterface.replace_value()
ImageInterface.sample()
ImageInterface.select()
ImageInterface.select_mask()
ImageInterface.unpack_scalar()
ImageInterface.values()
interface
Modulemultipath
ModuleMultiInterface
MultiInterface.applies()
MultiInterface.as_dframe()
MultiInterface.cast()
MultiInterface.compute()
MultiInterface.concatenate()
MultiInterface.indexed()
MultiInterface.isscalar()
MultiInterface.isunique()
MultiInterface.length()
MultiInterface.loaded()
MultiInterface.persist()
MultiInterface.replace_value()
MultiInterface.select()
MultiInterface.select_mask()
MultiInterface.select_paths()
MultiInterface.shape()
MultiInterface.split()
MultiInterface.values()
ensure_ring()
pandas
ModulePandasAPI
PandasInterface
PandasInterface.applies()
PandasInterface.as_dframe()
PandasInterface.cast()
PandasInterface.compute()
PandasInterface.concatenate()
PandasInterface.indexed()
PandasInterface.isunique()
PandasInterface.loaded()
PandasInterface.persist()
PandasInterface.replace_value()
PandasInterface.select_mask()
PandasInterface.unpack_scalar()
spatialpandas
ModuleSpatialPandasInterface
SpatialPandasInterface.applies()
SpatialPandasInterface.as_dframe()
SpatialPandasInterface.base_interface
SpatialPandasInterface.cast()
SpatialPandasInterface.compute()
SpatialPandasInterface.concatenate()
SpatialPandasInterface.indexed()
SpatialPandasInterface.isscalar()
SpatialPandasInterface.isunique()
SpatialPandasInterface.length()
SpatialPandasInterface.loaded()
SpatialPandasInterface.persist()
SpatialPandasInterface.replace_value()
SpatialPandasInterface.select()
SpatialPandasInterface.select_mask()
SpatialPandasInterface.select_paths()
SpatialPandasInterface.shape()
SpatialPandasInterface.split()
SpatialPandasInterface.values()
from_multi()
from_shapely()
geom_array_to_array()
geom_to_array()
geom_to_holes()
get_geom_type()
get_value_array()
to_geom_dict()
to_spatialpandas()
spatialpandas_dask
ModuleDaskSpatialPandasInterface
DaskSpatialPandasInterface.applies()
DaskSpatialPandasInterface.as_dframe()
DaskSpatialPandasInterface.base_interface
DaskSpatialPandasInterface.cast()
DaskSpatialPandasInterface.compute()
DaskSpatialPandasInterface.concatenate()
DaskSpatialPandasInterface.indexed()
DaskSpatialPandasInterface.isscalar()
DaskSpatialPandasInterface.isunique()
DaskSpatialPandasInterface.length()
DaskSpatialPandasInterface.loaded()
DaskSpatialPandasInterface.persist()
DaskSpatialPandasInterface.replace_value()
DaskSpatialPandasInterface.select()
DaskSpatialPandasInterface.select_mask()
DaskSpatialPandasInterface.select_paths()
DaskSpatialPandasInterface.shape()
DaskSpatialPandasInterface.split()
DaskSpatialPandasInterface.values()
util
Modulexarray
ModuleXArrayInterface
XArrayInterface.applies()
XArrayInterface.as_dframe()
XArrayInterface.canonicalize()
XArrayInterface.cast()
XArrayInterface.compute()
XArrayInterface.concatenate()
XArrayInterface.coords()
XArrayInterface.indexed()
XArrayInterface.isunique()
XArrayInterface.loaded()
XArrayInterface.persist()
XArrayInterface.replace_value()
XArrayInterface.sample()
XArrayInterface.select_mask()
XArrayInterface.unpack_scalar()